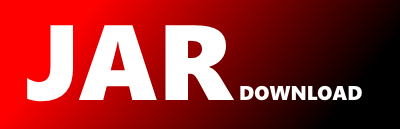
com.pulumi.azurenative.policyinsights.RemediationAtSubscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.policyinsights.RemediationAtSubscriptionArgs;
import com.pulumi.azurenative.policyinsights.outputs.RemediationDeploymentSummaryResponse;
import com.pulumi.azurenative.policyinsights.outputs.RemediationFiltersResponse;
import com.pulumi.azurenative.policyinsights.outputs.RemediationPropertiesResponseFailureThreshold;
import com.pulumi.azurenative.policyinsights.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The remediation definition.
* Azure REST API version: 2021-10-01. Prior API version in Azure Native 1.x: 2019-07-01.
*
* Other available API versions: 2018-07-01-preview.
*
* ## Example Usage
* ### Create remediation at subscription scope
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.policyinsights.RemediationAtSubscription;
* import com.pulumi.azurenative.policyinsights.RemediationAtSubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var remediationAtSubscription = new RemediationAtSubscription("remediationAtSubscription", RemediationAtSubscriptionArgs.builder()
* .policyAssignmentId("/subscriptions/35ee058e-5fa0-414c-8145-3ebb8d09b6e2/providers/microsoft.authorization/policyassignments/b101830944f246d8a14088c5")
* .remediationName("storageRemediation")
* .build());
*
* }
* }
*
* }
*
* ### Create remediation at subscription scope with all properties
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.policyinsights.RemediationAtSubscription;
* import com.pulumi.azurenative.policyinsights.RemediationAtSubscriptionArgs;
* import com.pulumi.azurenative.policyinsights.inputs.RemediationPropertiesFailureThresholdArgs;
* import com.pulumi.azurenative.policyinsights.inputs.RemediationFiltersArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var remediationAtSubscription = new RemediationAtSubscription("remediationAtSubscription", RemediationAtSubscriptionArgs.builder()
* .failureThreshold(RemediationPropertiesFailureThresholdArgs.builder()
* .percentage(0.1)
* .build())
* .filters(RemediationFiltersArgs.builder()
* .locations(
* "eastus",
* "westus")
* .build())
* .parallelDeployments(6)
* .policyAssignmentId("/subscriptions/35ee058e-5fa0-414c-8145-3ebb8d09b6e2/providers/microsoft.authorization/policyassignments/b101830944f246d8a14088c5")
* .policyDefinitionReferenceId("8c8fa9e4")
* .remediationName("storageRemediation")
* .resourceCount(42)
* .resourceDiscoveryMode("ReEvaluateCompliance")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:policyinsights:RemediationAtSubscription storageRemediation /subscriptions/{subscriptionId}/providers/Microsoft.PolicyInsights/remediations/{remediationName}
* ```
*
*/
@ResourceType(type="azure-native:policyinsights:RemediationAtSubscription")
public class RemediationAtSubscription extends com.pulumi.resources.CustomResource {
/**
* The remediation correlation Id. Can be used to find events related to the remediation in the activity log.
*
*/
@Export(name="correlationId", refs={String.class}, tree="[0]")
private Output correlationId;
/**
* @return The remediation correlation Id. Can be used to find events related to the remediation in the activity log.
*
*/
public Output correlationId() {
return this.correlationId;
}
/**
* The time at which the remediation was created.
*
*/
@Export(name="createdOn", refs={String.class}, tree="[0]")
private Output createdOn;
/**
* @return The time at which the remediation was created.
*
*/
public Output createdOn() {
return this.createdOn;
}
/**
* The deployment status summary for all deployments created by the remediation.
*
*/
@Export(name="deploymentStatus", refs={RemediationDeploymentSummaryResponse.class}, tree="[0]")
private Output deploymentStatus;
/**
* @return The deployment status summary for all deployments created by the remediation.
*
*/
public Output deploymentStatus() {
return this.deploymentStatus;
}
/**
* The remediation failure threshold settings
*
*/
@Export(name="failureThreshold", refs={RemediationPropertiesResponseFailureThreshold.class}, tree="[0]")
private Output* @Nullable */ RemediationPropertiesResponseFailureThreshold> failureThreshold;
/**
* @return The remediation failure threshold settings
*
*/
public Output> failureThreshold() {
return Codegen.optional(this.failureThreshold);
}
/**
* The filters that will be applied to determine which resources to remediate.
*
*/
@Export(name="filters", refs={RemediationFiltersResponse.class}, tree="[0]")
private Output* @Nullable */ RemediationFiltersResponse> filters;
/**
* @return The filters that will be applied to determine which resources to remediate.
*
*/
public Output> filters() {
return Codegen.optional(this.filters);
}
/**
* The time at which the remediation was last updated.
*
*/
@Export(name="lastUpdatedOn", refs={String.class}, tree="[0]")
private Output lastUpdatedOn;
/**
* @return The time at which the remediation was last updated.
*
*/
public Output lastUpdatedOn() {
return this.lastUpdatedOn;
}
/**
* The name of the remediation.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the remediation.
*
*/
public Output name() {
return this.name;
}
/**
* Determines how many resources to remediate at any given time. Can be used to increase or reduce the pace of the remediation. If not provided, the default parallel deployments value is used.
*
*/
@Export(name="parallelDeployments", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> parallelDeployments;
/**
* @return Determines how many resources to remediate at any given time. Can be used to increase or reduce the pace of the remediation. If not provided, the default parallel deployments value is used.
*
*/
public Output> parallelDeployments() {
return Codegen.optional(this.parallelDeployments);
}
/**
* The resource ID of the policy assignment that should be remediated.
*
*/
@Export(name="policyAssignmentId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> policyAssignmentId;
/**
* @return The resource ID of the policy assignment that should be remediated.
*
*/
public Output> policyAssignmentId() {
return Codegen.optional(this.policyAssignmentId);
}
/**
* The policy definition reference ID of the individual definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
*
*/
@Export(name="policyDefinitionReferenceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> policyDefinitionReferenceId;
/**
* @return The policy definition reference ID of the individual definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
*
*/
public Output> policyDefinitionReferenceId() {
return Codegen.optional(this.policyDefinitionReferenceId);
}
/**
* The status of the remediation. This refers to the entire remediation task, not individual deployments. Allowed values are Evaluating, Canceled, Cancelling, Failed, Complete, or Succeeded.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The status of the remediation. This refers to the entire remediation task, not individual deployments. Allowed values are Evaluating, Canceled, Cancelling, Failed, Complete, or Succeeded.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Determines the max number of resources that can be remediated by the remediation job. If not provided, the default resource count is used.
*
*/
@Export(name="resourceCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> resourceCount;
/**
* @return Determines the max number of resources that can be remediated by the remediation job. If not provided, the default resource count is used.
*
*/
public Output> resourceCount() {
return Codegen.optional(this.resourceCount);
}
/**
* The way resources to remediate are discovered. Defaults to ExistingNonCompliant if not specified.
*
*/
@Export(name="resourceDiscoveryMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> resourceDiscoveryMode;
/**
* @return The way resources to remediate are discovered. Defaults to ExistingNonCompliant if not specified.
*
*/
public Output> resourceDiscoveryMode() {
return Codegen.optional(this.resourceDiscoveryMode);
}
/**
* The remediation status message. Provides additional details regarding the state of the remediation.
*
*/
@Export(name="statusMessage", refs={String.class}, tree="[0]")
private Output statusMessage;
/**
* @return The remediation status message. Provides additional details regarding the state of the remediation.
*
*/
public Output statusMessage() {
return this.statusMessage;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the remediation.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the remediation.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public RemediationAtSubscription(java.lang.String name) {
this(name, RemediationAtSubscriptionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public RemediationAtSubscription(java.lang.String name, @Nullable RemediationAtSubscriptionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public RemediationAtSubscription(java.lang.String name, @Nullable RemediationAtSubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:policyinsights:RemediationAtSubscription", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private RemediationAtSubscription(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:policyinsights:RemediationAtSubscription", name, null, makeResourceOptions(options, id), false);
}
private static RemediationAtSubscriptionArgs makeArgs(@Nullable RemediationAtSubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? RemediationAtSubscriptionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:policyinsights/v20180701preview:RemediationAtSubscription").build()),
Output.of(Alias.builder().type("azure-native:policyinsights/v20190701:RemediationAtSubscription").build()),
Output.of(Alias.builder().type("azure-native:policyinsights/v20211001:RemediationAtSubscription").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static RemediationAtSubscription get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new RemediationAtSubscription(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy