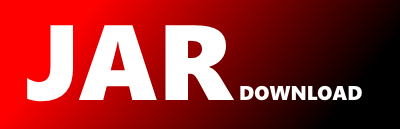
com.pulumi.azurenative.policyinsights.outputs.GetAttestationAtResourceGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights.outputs;
import com.pulumi.azurenative.policyinsights.outputs.AttestationEvidenceResponse;
import com.pulumi.azurenative.policyinsights.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAttestationAtResourceGroupResult {
/**
* @return The time the evidence was assessed
*
*/
private @Nullable String assessmentDate;
/**
* @return Comments describing why this attestation was created.
*
*/
private @Nullable String comments;
/**
* @return The compliance state that should be set on the resource.
*
*/
private @Nullable String complianceState;
/**
* @return The evidence supporting the compliance state set in this attestation.
*
*/
private @Nullable List evidence;
/**
* @return The time the compliance state should expire.
*
*/
private @Nullable String expiresOn;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The time the compliance state was last changed in this attestation.
*
*/
private String lastComplianceStateChangeAt;
/**
* @return Additional metadata for this attestation
*
*/
private @Nullable Object metadata;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The person responsible for setting the state of the resource. This value is typically an Azure Active Directory object ID.
*
*/
private @Nullable String owner;
/**
* @return The resource ID of the policy assignment that the attestation is setting the state for.
*
*/
private String policyAssignmentId;
/**
* @return The policy definition reference ID from a policy set definition that the attestation is setting the state for. If the policy assignment assigns a policy set definition the attestation can choose a definition within the set definition with this property or omit this and set the state for the entire set definition.
*
*/
private @Nullable String policyDefinitionReferenceId;
/**
* @return The status of the attestation.
*
*/
private String provisioningState;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetAttestationAtResourceGroupResult() {}
/**
* @return The time the evidence was assessed
*
*/
public Optional assessmentDate() {
return Optional.ofNullable(this.assessmentDate);
}
/**
* @return Comments describing why this attestation was created.
*
*/
public Optional comments() {
return Optional.ofNullable(this.comments);
}
/**
* @return The compliance state that should be set on the resource.
*
*/
public Optional complianceState() {
return Optional.ofNullable(this.complianceState);
}
/**
* @return The evidence supporting the compliance state set in this attestation.
*
*/
public List evidence() {
return this.evidence == null ? List.of() : this.evidence;
}
/**
* @return The time the compliance state should expire.
*
*/
public Optional expiresOn() {
return Optional.ofNullable(this.expiresOn);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The time the compliance state was last changed in this attestation.
*
*/
public String lastComplianceStateChangeAt() {
return this.lastComplianceStateChangeAt;
}
/**
* @return Additional metadata for this attestation
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy