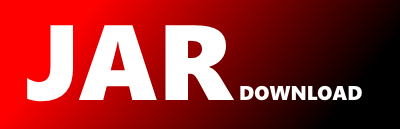
com.pulumi.azurenative.policyinsights.outputs.GetRemediationAtManagementGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights.outputs;
import com.pulumi.azurenative.policyinsights.outputs.RemediationDeploymentSummaryResponse;
import com.pulumi.azurenative.policyinsights.outputs.RemediationFiltersResponse;
import com.pulumi.azurenative.policyinsights.outputs.RemediationPropertiesResponseFailureThreshold;
import com.pulumi.azurenative.policyinsights.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetRemediationAtManagementGroupResult {
/**
* @return The remediation correlation Id. Can be used to find events related to the remediation in the activity log.
*
*/
private String correlationId;
/**
* @return The time at which the remediation was created.
*
*/
private String createdOn;
/**
* @return The deployment status summary for all deployments created by the remediation.
*
*/
private RemediationDeploymentSummaryResponse deploymentStatus;
/**
* @return The remediation failure threshold settings
*
*/
private @Nullable RemediationPropertiesResponseFailureThreshold failureThreshold;
/**
* @return The filters that will be applied to determine which resources to remediate.
*
*/
private @Nullable RemediationFiltersResponse filters;
/**
* @return The ID of the remediation.
*
*/
private String id;
/**
* @return The time at which the remediation was last updated.
*
*/
private String lastUpdatedOn;
/**
* @return The name of the remediation.
*
*/
private String name;
/**
* @return Determines how many resources to remediate at any given time. Can be used to increase or reduce the pace of the remediation. If not provided, the default parallel deployments value is used.
*
*/
private @Nullable Integer parallelDeployments;
/**
* @return The resource ID of the policy assignment that should be remediated.
*
*/
private @Nullable String policyAssignmentId;
/**
* @return The policy definition reference ID of the individual definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
*
*/
private @Nullable String policyDefinitionReferenceId;
/**
* @return The status of the remediation. This refers to the entire remediation task, not individual deployments. Allowed values are Evaluating, Canceled, Cancelling, Failed, Complete, or Succeeded.
*
*/
private String provisioningState;
/**
* @return Determines the max number of resources that can be remediated by the remediation job. If not provided, the default resource count is used.
*
*/
private @Nullable Integer resourceCount;
/**
* @return The way resources to remediate are discovered. Defaults to ExistingNonCompliant if not specified.
*
*/
private @Nullable String resourceDiscoveryMode;
/**
* @return The remediation status message. Provides additional details regarding the state of the remediation.
*
*/
private String statusMessage;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the remediation.
*
*/
private String type;
private GetRemediationAtManagementGroupResult() {}
/**
* @return The remediation correlation Id. Can be used to find events related to the remediation in the activity log.
*
*/
public String correlationId() {
return this.correlationId;
}
/**
* @return The time at which the remediation was created.
*
*/
public String createdOn() {
return this.createdOn;
}
/**
* @return The deployment status summary for all deployments created by the remediation.
*
*/
public RemediationDeploymentSummaryResponse deploymentStatus() {
return this.deploymentStatus;
}
/**
* @return The remediation failure threshold settings
*
*/
public Optional failureThreshold() {
return Optional.ofNullable(this.failureThreshold);
}
/**
* @return The filters that will be applied to determine which resources to remediate.
*
*/
public Optional filters() {
return Optional.ofNullable(this.filters);
}
/**
* @return The ID of the remediation.
*
*/
public String id() {
return this.id;
}
/**
* @return The time at which the remediation was last updated.
*
*/
public String lastUpdatedOn() {
return this.lastUpdatedOn;
}
/**
* @return The name of the remediation.
*
*/
public String name() {
return this.name;
}
/**
* @return Determines how many resources to remediate at any given time. Can be used to increase or reduce the pace of the remediation. If not provided, the default parallel deployments value is used.
*
*/
public Optional parallelDeployments() {
return Optional.ofNullable(this.parallelDeployments);
}
/**
* @return The resource ID of the policy assignment that should be remediated.
*
*/
public Optional policyAssignmentId() {
return Optional.ofNullable(this.policyAssignmentId);
}
/**
* @return The policy definition reference ID of the individual definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
*
*/
public Optional policyDefinitionReferenceId() {
return Optional.ofNullable(this.policyDefinitionReferenceId);
}
/**
* @return The status of the remediation. This refers to the entire remediation task, not individual deployments. Allowed values are Evaluating, Canceled, Cancelling, Failed, Complete, or Succeeded.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Determines the max number of resources that can be remediated by the remediation job. If not provided, the default resource count is used.
*
*/
public Optional resourceCount() {
return Optional.ofNullable(this.resourceCount);
}
/**
* @return The way resources to remediate are discovered. Defaults to ExistingNonCompliant if not specified.
*
*/
public Optional resourceDiscoveryMode() {
return Optional.ofNullable(this.resourceDiscoveryMode);
}
/**
* @return The remediation status message. Provides additional details regarding the state of the remediation.
*
*/
public String statusMessage() {
return this.statusMessage;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the remediation.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRemediationAtManagementGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String correlationId;
private String createdOn;
private RemediationDeploymentSummaryResponse deploymentStatus;
private @Nullable RemediationPropertiesResponseFailureThreshold failureThreshold;
private @Nullable RemediationFiltersResponse filters;
private String id;
private String lastUpdatedOn;
private String name;
private @Nullable Integer parallelDeployments;
private @Nullable String policyAssignmentId;
private @Nullable String policyDefinitionReferenceId;
private String provisioningState;
private @Nullable Integer resourceCount;
private @Nullable String resourceDiscoveryMode;
private String statusMessage;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetRemediationAtManagementGroupResult defaults) {
Objects.requireNonNull(defaults);
this.correlationId = defaults.correlationId;
this.createdOn = defaults.createdOn;
this.deploymentStatus = defaults.deploymentStatus;
this.failureThreshold = defaults.failureThreshold;
this.filters = defaults.filters;
this.id = defaults.id;
this.lastUpdatedOn = defaults.lastUpdatedOn;
this.name = defaults.name;
this.parallelDeployments = defaults.parallelDeployments;
this.policyAssignmentId = defaults.policyAssignmentId;
this.policyDefinitionReferenceId = defaults.policyDefinitionReferenceId;
this.provisioningState = defaults.provisioningState;
this.resourceCount = defaults.resourceCount;
this.resourceDiscoveryMode = defaults.resourceDiscoveryMode;
this.statusMessage = defaults.statusMessage;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder correlationId(String correlationId) {
if (correlationId == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "correlationId");
}
this.correlationId = correlationId;
return this;
}
@CustomType.Setter
public Builder createdOn(String createdOn) {
if (createdOn == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "createdOn");
}
this.createdOn = createdOn;
return this;
}
@CustomType.Setter
public Builder deploymentStatus(RemediationDeploymentSummaryResponse deploymentStatus) {
if (deploymentStatus == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "deploymentStatus");
}
this.deploymentStatus = deploymentStatus;
return this;
}
@CustomType.Setter
public Builder failureThreshold(@Nullable RemediationPropertiesResponseFailureThreshold failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable RemediationFiltersResponse filters) {
this.filters = filters;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastUpdatedOn(String lastUpdatedOn) {
if (lastUpdatedOn == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "lastUpdatedOn");
}
this.lastUpdatedOn = lastUpdatedOn;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder parallelDeployments(@Nullable Integer parallelDeployments) {
this.parallelDeployments = parallelDeployments;
return this;
}
@CustomType.Setter
public Builder policyAssignmentId(@Nullable String policyAssignmentId) {
this.policyAssignmentId = policyAssignmentId;
return this;
}
@CustomType.Setter
public Builder policyDefinitionReferenceId(@Nullable String policyDefinitionReferenceId) {
this.policyDefinitionReferenceId = policyDefinitionReferenceId;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceCount(@Nullable Integer resourceCount) {
this.resourceCount = resourceCount;
return this;
}
@CustomType.Setter
public Builder resourceDiscoveryMode(@Nullable String resourceDiscoveryMode) {
this.resourceDiscoveryMode = resourceDiscoveryMode;
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
if (statusMessage == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "statusMessage");
}
this.statusMessage = statusMessage;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetRemediationAtManagementGroupResult", "type");
}
this.type = type;
return this;
}
public GetRemediationAtManagementGroupResult build() {
final var _resultValue = new GetRemediationAtManagementGroupResult();
_resultValue.correlationId = correlationId;
_resultValue.createdOn = createdOn;
_resultValue.deploymentStatus = deploymentStatus;
_resultValue.failureThreshold = failureThreshold;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.lastUpdatedOn = lastUpdatedOn;
_resultValue.name = name;
_resultValue.parallelDeployments = parallelDeployments;
_resultValue.policyAssignmentId = policyAssignmentId;
_resultValue.policyDefinitionReferenceId = policyDefinitionReferenceId;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceCount = resourceCount;
_resultValue.resourceDiscoveryMode = resourceDiscoveryMode;
_resultValue.statusMessage = statusMessage;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy