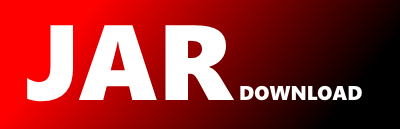
com.pulumi.azurenative.professionalservice.outputs.ProfessionalServiceResourceResponseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.professionalservice.outputs;
import com.pulumi.azurenative.professionalservice.outputs.ProfessionalServicePropertiesResponseTerm;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProfessionalServiceResourceResponseProperties {
/**
* @return Whether the ProfessionalService subscription will auto renew upon term end.
*
*/
private @Nullable Boolean autoRenew;
/**
* @return The billing period eg P1M,P1Y for monthly,yearly respectively
*
*/
private @Nullable String billingPeriod;
/**
* @return The created date of this resource.
*
*/
private String created;
/**
* @return Whether the current term is a Free Trial term
*
*/
private @Nullable Boolean isFreeTrial;
/**
* @return The last modifier date if this resource.
*
*/
private @Nullable String lastModified;
/**
* @return The offer id.
*
*/
private @Nullable String offerId;
/**
* @return The metadata about the ProfessionalService subscription such as the AzureSubscriptionId and ResourceUri.
*
*/
private @Nullable Map paymentChannelMetadata;
/**
* @return The Payment channel for the ProfessionalServiceSubscription.
*
*/
private @Nullable String paymentChannelType;
/**
* @return The publisher id.
*
*/
private @Nullable String publisherId;
/**
* @return The quote id which the ProfessionalService will be purchase with.
*
*/
private @Nullable String quoteId;
/**
* @return The plan id.
*
*/
private @Nullable String skuId;
/**
* @return The ProfessionalService Subscription Status.
*
*/
private @Nullable String status;
/**
* @return The store front which initiates the purchase.
*
*/
private @Nullable String storeFront;
/**
* @return The current Term object.
*
*/
private @Nullable ProfessionalServicePropertiesResponseTerm term;
/**
* @return The unit term eg P1M,P1Y,P2Y,P3Y meaning month,1year,2year,3year respectively
*
*/
private @Nullable String termUnit;
private ProfessionalServiceResourceResponseProperties() {}
/**
* @return Whether the ProfessionalService subscription will auto renew upon term end.
*
*/
public Optional autoRenew() {
return Optional.ofNullable(this.autoRenew);
}
/**
* @return The billing period eg P1M,P1Y for monthly,yearly respectively
*
*/
public Optional billingPeriod() {
return Optional.ofNullable(this.billingPeriod);
}
/**
* @return The created date of this resource.
*
*/
public String created() {
return this.created;
}
/**
* @return Whether the current term is a Free Trial term
*
*/
public Optional isFreeTrial() {
return Optional.ofNullable(this.isFreeTrial);
}
/**
* @return The last modifier date if this resource.
*
*/
public Optional lastModified() {
return Optional.ofNullable(this.lastModified);
}
/**
* @return The offer id.
*
*/
public Optional offerId() {
return Optional.ofNullable(this.offerId);
}
/**
* @return The metadata about the ProfessionalService subscription such as the AzureSubscriptionId and ResourceUri.
*
*/
public Map paymentChannelMetadata() {
return this.paymentChannelMetadata == null ? Map.of() : this.paymentChannelMetadata;
}
/**
* @return The Payment channel for the ProfessionalServiceSubscription.
*
*/
public Optional paymentChannelType() {
return Optional.ofNullable(this.paymentChannelType);
}
/**
* @return The publisher id.
*
*/
public Optional publisherId() {
return Optional.ofNullable(this.publisherId);
}
/**
* @return The quote id which the ProfessionalService will be purchase with.
*
*/
public Optional quoteId() {
return Optional.ofNullable(this.quoteId);
}
/**
* @return The plan id.
*
*/
public Optional skuId() {
return Optional.ofNullable(this.skuId);
}
/**
* @return The ProfessionalService Subscription Status.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return The store front which initiates the purchase.
*
*/
public Optional storeFront() {
return Optional.ofNullable(this.storeFront);
}
/**
* @return The current Term object.
*
*/
public Optional term() {
return Optional.ofNullable(this.term);
}
/**
* @return The unit term eg P1M,P1Y,P2Y,P3Y meaning month,1year,2year,3year respectively
*
*/
public Optional termUnit() {
return Optional.ofNullable(this.termUnit);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProfessionalServiceResourceResponseProperties defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean autoRenew;
private @Nullable String billingPeriod;
private String created;
private @Nullable Boolean isFreeTrial;
private @Nullable String lastModified;
private @Nullable String offerId;
private @Nullable Map paymentChannelMetadata;
private @Nullable String paymentChannelType;
private @Nullable String publisherId;
private @Nullable String quoteId;
private @Nullable String skuId;
private @Nullable String status;
private @Nullable String storeFront;
private @Nullable ProfessionalServicePropertiesResponseTerm term;
private @Nullable String termUnit;
public Builder() {}
public Builder(ProfessionalServiceResourceResponseProperties defaults) {
Objects.requireNonNull(defaults);
this.autoRenew = defaults.autoRenew;
this.billingPeriod = defaults.billingPeriod;
this.created = defaults.created;
this.isFreeTrial = defaults.isFreeTrial;
this.lastModified = defaults.lastModified;
this.offerId = defaults.offerId;
this.paymentChannelMetadata = defaults.paymentChannelMetadata;
this.paymentChannelType = defaults.paymentChannelType;
this.publisherId = defaults.publisherId;
this.quoteId = defaults.quoteId;
this.skuId = defaults.skuId;
this.status = defaults.status;
this.storeFront = defaults.storeFront;
this.term = defaults.term;
this.termUnit = defaults.termUnit;
}
@CustomType.Setter
public Builder autoRenew(@Nullable Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
@CustomType.Setter
public Builder billingPeriod(@Nullable String billingPeriod) {
this.billingPeriod = billingPeriod;
return this;
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("ProfessionalServiceResourceResponseProperties", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder isFreeTrial(@Nullable Boolean isFreeTrial) {
this.isFreeTrial = isFreeTrial;
return this;
}
@CustomType.Setter
public Builder lastModified(@Nullable String lastModified) {
this.lastModified = lastModified;
return this;
}
@CustomType.Setter
public Builder offerId(@Nullable String offerId) {
this.offerId = offerId;
return this;
}
@CustomType.Setter
public Builder paymentChannelMetadata(@Nullable Map paymentChannelMetadata) {
this.paymentChannelMetadata = paymentChannelMetadata;
return this;
}
@CustomType.Setter
public Builder paymentChannelType(@Nullable String paymentChannelType) {
this.paymentChannelType = paymentChannelType;
return this;
}
@CustomType.Setter
public Builder publisherId(@Nullable String publisherId) {
this.publisherId = publisherId;
return this;
}
@CustomType.Setter
public Builder quoteId(@Nullable String quoteId) {
this.quoteId = quoteId;
return this;
}
@CustomType.Setter
public Builder skuId(@Nullable String skuId) {
this.skuId = skuId;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder storeFront(@Nullable String storeFront) {
this.storeFront = storeFront;
return this;
}
@CustomType.Setter
public Builder term(@Nullable ProfessionalServicePropertiesResponseTerm term) {
this.term = term;
return this;
}
@CustomType.Setter
public Builder termUnit(@Nullable String termUnit) {
this.termUnit = termUnit;
return this;
}
public ProfessionalServiceResourceResponseProperties build() {
final var _resultValue = new ProfessionalServiceResourceResponseProperties();
_resultValue.autoRenew = autoRenew;
_resultValue.billingPeriod = billingPeriod;
_resultValue.created = created;
_resultValue.isFreeTrial = isFreeTrial;
_resultValue.lastModified = lastModified;
_resultValue.offerId = offerId;
_resultValue.paymentChannelMetadata = paymentChannelMetadata;
_resultValue.paymentChannelType = paymentChannelType;
_resultValue.publisherId = publisherId;
_resultValue.quoteId = quoteId;
_resultValue.skuId = skuId;
_resultValue.status = status;
_resultValue.storeFront = storeFront;
_resultValue.term = term;
_resultValue.termUnit = termUnit;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy