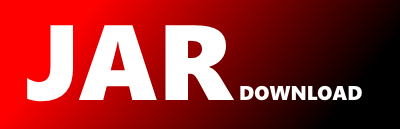
com.pulumi.azurenative.providerhub.ProviderhubFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.providerhub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.providerhub.inputs.GetDefaultRolloutArgs;
import com.pulumi.azurenative.providerhub.inputs.GetDefaultRolloutPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetNotificationRegistrationArgs;
import com.pulumi.azurenative.providerhub.inputs.GetNotificationRegistrationPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetProviderRegistrationArgs;
import com.pulumi.azurenative.providerhub.inputs.GetProviderRegistrationPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetResourceTypeRegistrationArgs;
import com.pulumi.azurenative.providerhub.inputs.GetResourceTypeRegistrationPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeFirstArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeFirstPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeSecondArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeSecondPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeThirdArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusNestedResourceTypeThirdPlainArgs;
import com.pulumi.azurenative.providerhub.inputs.GetSkusPlainArgs;
import com.pulumi.azurenative.providerhub.outputs.GetDefaultRolloutResult;
import com.pulumi.azurenative.providerhub.outputs.GetNotificationRegistrationResult;
import com.pulumi.azurenative.providerhub.outputs.GetProviderRegistrationResult;
import com.pulumi.azurenative.providerhub.outputs.GetResourceTypeRegistrationResult;
import com.pulumi.azurenative.providerhub.outputs.GetSkusNestedResourceTypeFirstResult;
import com.pulumi.azurenative.providerhub.outputs.GetSkusNestedResourceTypeSecondResult;
import com.pulumi.azurenative.providerhub.outputs.GetSkusNestedResourceTypeThirdResult;
import com.pulumi.azurenative.providerhub.outputs.GetSkusResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ProviderhubFunctions {
/**
* Gets the default rollout details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getDefaultRollout(GetDefaultRolloutArgs args) {
return getDefaultRollout(args, InvokeOptions.Empty);
}
/**
* Gets the default rollout details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getDefaultRolloutPlain(GetDefaultRolloutPlainArgs args) {
return getDefaultRolloutPlain(args, InvokeOptions.Empty);
}
/**
* Gets the default rollout details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getDefaultRollout(GetDefaultRolloutArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getDefaultRollout", TypeShape.of(GetDefaultRolloutResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the default rollout details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getDefaultRolloutPlain(GetDefaultRolloutPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getDefaultRollout", TypeShape.of(GetDefaultRolloutResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the notification registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getNotificationRegistration(GetNotificationRegistrationArgs args) {
return getNotificationRegistration(args, InvokeOptions.Empty);
}
/**
* Gets the notification registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getNotificationRegistrationPlain(GetNotificationRegistrationPlainArgs args) {
return getNotificationRegistrationPlain(args, InvokeOptions.Empty);
}
/**
* Gets the notification registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getNotificationRegistration(GetNotificationRegistrationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getNotificationRegistration", TypeShape.of(GetNotificationRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the notification registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getNotificationRegistrationPlain(GetNotificationRegistrationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getNotificationRegistration", TypeShape.of(GetNotificationRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the provider registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getProviderRegistration(GetProviderRegistrationArgs args) {
return getProviderRegistration(args, InvokeOptions.Empty);
}
/**
* Gets the provider registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getProviderRegistrationPlain(GetProviderRegistrationPlainArgs args) {
return getProviderRegistrationPlain(args, InvokeOptions.Empty);
}
/**
* Gets the provider registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getProviderRegistration(GetProviderRegistrationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getProviderRegistration", TypeShape.of(GetProviderRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the provider registration details.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getProviderRegistrationPlain(GetProviderRegistrationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getProviderRegistration", TypeShape.of(GetProviderRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource type details in the given subscription and provider.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getResourceTypeRegistration(GetResourceTypeRegistrationArgs args) {
return getResourceTypeRegistration(args, InvokeOptions.Empty);
}
/**
* Gets a resource type details in the given subscription and provider.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getResourceTypeRegistrationPlain(GetResourceTypeRegistrationPlainArgs args) {
return getResourceTypeRegistrationPlain(args, InvokeOptions.Empty);
}
/**
* Gets a resource type details in the given subscription and provider.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getResourceTypeRegistration(GetResourceTypeRegistrationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getResourceTypeRegistration", TypeShape.of(GetResourceTypeRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource type details in the given subscription and provider.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getResourceTypeRegistrationPlain(GetResourceTypeRegistrationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getResourceTypeRegistration", TypeShape.of(GetResourceTypeRegistrationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkus(GetSkusArgs args) {
return getSkus(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusPlain(GetSkusPlainArgs args) {
return getSkusPlain(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkus(GetSkusArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getSkus", TypeShape.of(GetSkusResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusPlain(GetSkusPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getSkus", TypeShape.of(GetSkusResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeFirst(GetSkusNestedResourceTypeFirstArgs args) {
return getSkusNestedResourceTypeFirst(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeFirstPlain(GetSkusNestedResourceTypeFirstPlainArgs args) {
return getSkusNestedResourceTypeFirstPlain(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeFirst(GetSkusNestedResourceTypeFirstArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getSkusNestedResourceTypeFirst", TypeShape.of(GetSkusNestedResourceTypeFirstResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeFirstPlain(GetSkusNestedResourceTypeFirstPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getSkusNestedResourceTypeFirst", TypeShape.of(GetSkusNestedResourceTypeFirstResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeSecond(GetSkusNestedResourceTypeSecondArgs args) {
return getSkusNestedResourceTypeSecond(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeSecondPlain(GetSkusNestedResourceTypeSecondPlainArgs args) {
return getSkusNestedResourceTypeSecondPlain(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeSecond(GetSkusNestedResourceTypeSecondArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getSkusNestedResourceTypeSecond", TypeShape.of(GetSkusNestedResourceTypeSecondResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeSecondPlain(GetSkusNestedResourceTypeSecondPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getSkusNestedResourceTypeSecond", TypeShape.of(GetSkusNestedResourceTypeSecondResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeThird(GetSkusNestedResourceTypeThirdArgs args) {
return getSkusNestedResourceTypeThird(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeThirdPlain(GetSkusNestedResourceTypeThirdPlainArgs args) {
return getSkusNestedResourceTypeThirdPlain(args, InvokeOptions.Empty);
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static Output getSkusNestedResourceTypeThird(GetSkusNestedResourceTypeThirdArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:providerhub:getSkusNestedResourceTypeThird", TypeShape.of(GetSkusNestedResourceTypeThirdResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the sku details for the given resource type and sku name.
* Azure REST API version: 2021-09-01-preview.
*
*/
public static CompletableFuture getSkusNestedResourceTypeThirdPlain(GetSkusNestedResourceTypeThirdPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:providerhub:getSkusNestedResourceTypeThird", TypeShape.of(GetSkusNestedResourceTypeThirdResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy