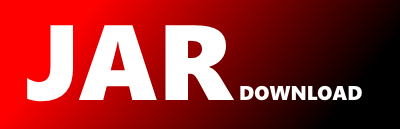
com.pulumi.azurenative.recoveryservices.inputs.A2ASharedDiskReplicationDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.inputs.A2AProtectedManagedDiskDetailsArgs;
import com.pulumi.azurenative.recoveryservices.inputs.A2AUnprotectedDiskDetailsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A2A provider specific settings.
*
*/
public final class A2ASharedDiskReplicationDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final A2ASharedDiskReplicationDetailsArgs Empty = new A2ASharedDiskReplicationDetailsArgs();
/**
* The recovery point id to which the Virtual node was failed over.
*
*/
@Import(name="failoverRecoveryPointId")
private @Nullable Output failoverRecoveryPointId;
/**
* @return The recovery point id to which the Virtual node was failed over.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy