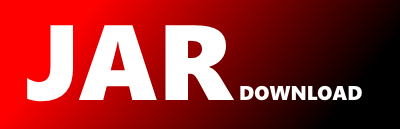
com.pulumi.azurenative.recoveryservices.inputs.AzureVmWorkloadProtectionPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.WorkloadType;
import com.pulumi.azurenative.recoveryservices.inputs.SettingsArgs;
import com.pulumi.azurenative.recoveryservices.inputs.SubProtectionPolicyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Azure VM (Mercury) workload-specific backup policy.
*
*/
public final class AzureVmWorkloadProtectionPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureVmWorkloadProtectionPolicyArgs Empty = new AzureVmWorkloadProtectionPolicyArgs();
/**
* This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
*
*/
@Import(name="backupManagementType", required=true)
private Output backupManagementType;
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
*
*/
public Output backupManagementType() {
return this.backupManagementType;
}
/**
* Fix the policy inconsistency
*
*/
@Import(name="makePolicyConsistent")
private @Nullable Output makePolicyConsistent;
/**
* @return Fix the policy inconsistency
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy