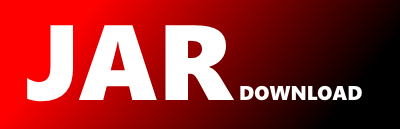
com.pulumi.azurenative.recoveryservices.inputs.AzureVmWorkloadSAPAseDatabaseProtectedItemArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.CreateMode;
import com.pulumi.azurenative.recoveryservices.enums.LastBackupStatus;
import com.pulumi.azurenative.recoveryservices.enums.ProtectedItemHealthStatus;
import com.pulumi.azurenative.recoveryservices.enums.ProtectionState;
import com.pulumi.azurenative.recoveryservices.inputs.AzureVmWorkloadProtectedItemExtendedInfoArgs;
import com.pulumi.azurenative.recoveryservices.inputs.DistributedNodesInfoArgs;
import com.pulumi.azurenative.recoveryservices.inputs.KPIResourceHealthDetailsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Azure VM workload-specific protected item representing SAP ASE Database.
*
*/
public final class AzureVmWorkloadSAPAseDatabaseProtectedItemArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureVmWorkloadSAPAseDatabaseProtectedItemArgs Empty = new AzureVmWorkloadSAPAseDatabaseProtectedItemArgs();
/**
* Name of the backup set the backup item belongs to
*
*/
@Import(name="backupSetName")
private @Nullable Output backupSetName;
/**
* @return Name of the backup set the backup item belongs to
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy