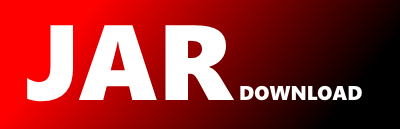
com.pulumi.azurenative.recoveryservices.inputs.SkuArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.SkuName;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Identifies the unique system identifier for each Azure resource.
*
*/
public final class SkuArgs extends com.pulumi.resources.ResourceArgs {
public static final SkuArgs Empty = new SkuArgs();
/**
* The sku capacity
*
*/
@Import(name="capacity")
private @Nullable Output capacity;
/**
* @return The sku capacity
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy