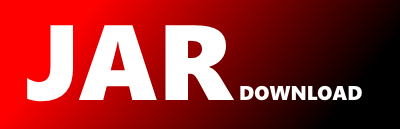
com.pulumi.azurenative.recoveryservices.inputs.SubProtectionPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.PolicyType;
import com.pulumi.azurenative.recoveryservices.inputs.LogSchedulePolicyArgs;
import com.pulumi.azurenative.recoveryservices.inputs.LongTermRetentionPolicyArgs;
import com.pulumi.azurenative.recoveryservices.inputs.LongTermSchedulePolicyArgs;
import com.pulumi.azurenative.recoveryservices.inputs.SimpleRetentionPolicyArgs;
import com.pulumi.azurenative.recoveryservices.inputs.SimpleSchedulePolicyArgs;
import com.pulumi.azurenative.recoveryservices.inputs.SimpleSchedulePolicyV2Args;
import com.pulumi.azurenative.recoveryservices.inputs.TieringPolicyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Object;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Sub-protection policy which includes schedule and retention
*
*/
public final class SubProtectionPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final SubProtectionPolicyArgs Empty = new SubProtectionPolicyArgs();
/**
* Type of backup policy type
*
*/
@Import(name="policyType")
private @Nullable Output> policyType;
/**
* @return Type of backup policy type
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy