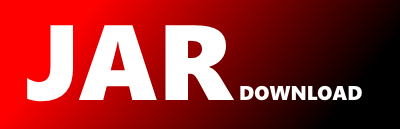
com.pulumi.azurenative.recoveryservices.outputs.AzureSqlProtectionPolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.LongTermRetentionPolicyResponse;
import com.pulumi.azurenative.recoveryservices.outputs.SimpleRetentionPolicyResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureSqlProtectionPolicyResponse {
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureSql'.
*
*/
private String backupManagementType;
/**
* @return Number of items associated with this policy.
*
*/
private @Nullable Integer protectedItemsCount;
/**
* @return ResourceGuard Operation Requests
*
*/
private @Nullable List resourceGuardOperationRequests;
/**
* @return Retention policy details.
*
*/
private @Nullable Either retentionPolicy;
private AzureSqlProtectionPolicyResponse() {}
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureSql'.
*
*/
public String backupManagementType() {
return this.backupManagementType;
}
/**
* @return Number of items associated with this policy.
*
*/
public Optional protectedItemsCount() {
return Optional.ofNullable(this.protectedItemsCount);
}
/**
* @return ResourceGuard Operation Requests
*
*/
public List resourceGuardOperationRequests() {
return this.resourceGuardOperationRequests == null ? List.of() : this.resourceGuardOperationRequests;
}
/**
* @return Retention policy details.
*
*/
public Optional> retentionPolicy() {
return Optional.ofNullable(this.retentionPolicy);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureSqlProtectionPolicyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String backupManagementType;
private @Nullable Integer protectedItemsCount;
private @Nullable List resourceGuardOperationRequests;
private @Nullable Either retentionPolicy;
public Builder() {}
public Builder(AzureSqlProtectionPolicyResponse defaults) {
Objects.requireNonNull(defaults);
this.backupManagementType = defaults.backupManagementType;
this.protectedItemsCount = defaults.protectedItemsCount;
this.resourceGuardOperationRequests = defaults.resourceGuardOperationRequests;
this.retentionPolicy = defaults.retentionPolicy;
}
@CustomType.Setter
public Builder backupManagementType(String backupManagementType) {
if (backupManagementType == null) {
throw new MissingRequiredPropertyException("AzureSqlProtectionPolicyResponse", "backupManagementType");
}
this.backupManagementType = backupManagementType;
return this;
}
@CustomType.Setter
public Builder protectedItemsCount(@Nullable Integer protectedItemsCount) {
this.protectedItemsCount = protectedItemsCount;
return this;
}
@CustomType.Setter
public Builder resourceGuardOperationRequests(@Nullable List resourceGuardOperationRequests) {
this.resourceGuardOperationRequests = resourceGuardOperationRequests;
return this;
}
public Builder resourceGuardOperationRequests(String... resourceGuardOperationRequests) {
return resourceGuardOperationRequests(List.of(resourceGuardOperationRequests));
}
@CustomType.Setter
public Builder retentionPolicy(@Nullable Either retentionPolicy) {
this.retentionPolicy = retentionPolicy;
return this;
}
public AzureSqlProtectionPolicyResponse build() {
final var _resultValue = new AzureSqlProtectionPolicyResponse();
_resultValue.backupManagementType = backupManagementType;
_resultValue.protectedItemsCount = protectedItemsCount;
_resultValue.resourceGuardOperationRequests = resourceGuardOperationRequests;
_resultValue.retentionPolicy = retentionPolicy;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy