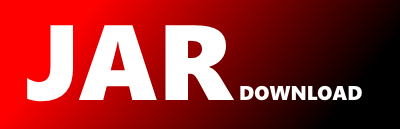
com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFabricSpecificDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.AgentDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.DraDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.IdentityProviderDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.MarsAgentDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.ProcessServerDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.PushInstallerDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.RcmProxyDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.ReplicationAgentDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.ReprotectAgentDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InMageRcmFabricSpecificDetailsResponse {
/**
* @return The list of agent details.
*
*/
private List agentDetails;
/**
* @return The control plane Uri.
*
*/
private String controlPlaneUri;
/**
* @return The data plane Uri.
*
*/
private String dataPlaneUri;
/**
* @return The list of DRAs.
*
*/
private List dras;
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'InMageRcm'.
*
*/
private String instanceType;
/**
* @return The list of Mars agents.
*
*/
private List marsAgents;
/**
* @return The ARM Id of the physical site.
*
*/
private String physicalSiteId;
/**
* @return The list of process servers.
*
*/
private List processServers;
/**
* @return The list of push installers.
*
*/
private List pushInstallers;
/**
* @return The list of RCM proxies.
*
*/
private List rcmProxies;
/**
* @return The list of replication agents.
*
*/
private List replicationAgents;
/**
* @return The list of reprotect agents.
*
*/
private List reprotectAgents;
/**
* @return The service container Id.
*
*/
private String serviceContainerId;
/**
* @return The service endpoint.
*
*/
private String serviceEndpoint;
/**
* @return The service resource Id.
*
*/
private String serviceResourceId;
/**
* @return The source agent identity details.
*
*/
private @Nullable IdentityProviderDetailsResponse sourceAgentIdentityDetails;
/**
* @return The ARM Id of the VMware site.
*
*/
private String vmwareSiteId;
private InMageRcmFabricSpecificDetailsResponse() {}
/**
* @return The list of agent details.
*
*/
public List agentDetails() {
return this.agentDetails;
}
/**
* @return The control plane Uri.
*
*/
public String controlPlaneUri() {
return this.controlPlaneUri;
}
/**
* @return The data plane Uri.
*
*/
public String dataPlaneUri() {
return this.dataPlaneUri;
}
/**
* @return The list of DRAs.
*
*/
public List dras() {
return this.dras;
}
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'InMageRcm'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The list of Mars agents.
*
*/
public List marsAgents() {
return this.marsAgents;
}
/**
* @return The ARM Id of the physical site.
*
*/
public String physicalSiteId() {
return this.physicalSiteId;
}
/**
* @return The list of process servers.
*
*/
public List processServers() {
return this.processServers;
}
/**
* @return The list of push installers.
*
*/
public List pushInstallers() {
return this.pushInstallers;
}
/**
* @return The list of RCM proxies.
*
*/
public List rcmProxies() {
return this.rcmProxies;
}
/**
* @return The list of replication agents.
*
*/
public List replicationAgents() {
return this.replicationAgents;
}
/**
* @return The list of reprotect agents.
*
*/
public List reprotectAgents() {
return this.reprotectAgents;
}
/**
* @return The service container Id.
*
*/
public String serviceContainerId() {
return this.serviceContainerId;
}
/**
* @return The service endpoint.
*
*/
public String serviceEndpoint() {
return this.serviceEndpoint;
}
/**
* @return The service resource Id.
*
*/
public String serviceResourceId() {
return this.serviceResourceId;
}
/**
* @return The source agent identity details.
*
*/
public Optional sourceAgentIdentityDetails() {
return Optional.ofNullable(this.sourceAgentIdentityDetails);
}
/**
* @return The ARM Id of the VMware site.
*
*/
public String vmwareSiteId() {
return this.vmwareSiteId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InMageRcmFabricSpecificDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List agentDetails;
private String controlPlaneUri;
private String dataPlaneUri;
private List dras;
private String instanceType;
private List marsAgents;
private String physicalSiteId;
private List processServers;
private List pushInstallers;
private List rcmProxies;
private List replicationAgents;
private List reprotectAgents;
private String serviceContainerId;
private String serviceEndpoint;
private String serviceResourceId;
private @Nullable IdentityProviderDetailsResponse sourceAgentIdentityDetails;
private String vmwareSiteId;
public Builder() {}
public Builder(InMageRcmFabricSpecificDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.agentDetails = defaults.agentDetails;
this.controlPlaneUri = defaults.controlPlaneUri;
this.dataPlaneUri = defaults.dataPlaneUri;
this.dras = defaults.dras;
this.instanceType = defaults.instanceType;
this.marsAgents = defaults.marsAgents;
this.physicalSiteId = defaults.physicalSiteId;
this.processServers = defaults.processServers;
this.pushInstallers = defaults.pushInstallers;
this.rcmProxies = defaults.rcmProxies;
this.replicationAgents = defaults.replicationAgents;
this.reprotectAgents = defaults.reprotectAgents;
this.serviceContainerId = defaults.serviceContainerId;
this.serviceEndpoint = defaults.serviceEndpoint;
this.serviceResourceId = defaults.serviceResourceId;
this.sourceAgentIdentityDetails = defaults.sourceAgentIdentityDetails;
this.vmwareSiteId = defaults.vmwareSiteId;
}
@CustomType.Setter
public Builder agentDetails(List agentDetails) {
if (agentDetails == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "agentDetails");
}
this.agentDetails = agentDetails;
return this;
}
public Builder agentDetails(AgentDetailsResponse... agentDetails) {
return agentDetails(List.of(agentDetails));
}
@CustomType.Setter
public Builder controlPlaneUri(String controlPlaneUri) {
if (controlPlaneUri == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "controlPlaneUri");
}
this.controlPlaneUri = controlPlaneUri;
return this;
}
@CustomType.Setter
public Builder dataPlaneUri(String dataPlaneUri) {
if (dataPlaneUri == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "dataPlaneUri");
}
this.dataPlaneUri = dataPlaneUri;
return this;
}
@CustomType.Setter
public Builder dras(List dras) {
if (dras == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "dras");
}
this.dras = dras;
return this;
}
public Builder dras(DraDetailsResponse... dras) {
return dras(List.of(dras));
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder marsAgents(List marsAgents) {
if (marsAgents == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "marsAgents");
}
this.marsAgents = marsAgents;
return this;
}
public Builder marsAgents(MarsAgentDetailsResponse... marsAgents) {
return marsAgents(List.of(marsAgents));
}
@CustomType.Setter
public Builder physicalSiteId(String physicalSiteId) {
if (physicalSiteId == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "physicalSiteId");
}
this.physicalSiteId = physicalSiteId;
return this;
}
@CustomType.Setter
public Builder processServers(List processServers) {
if (processServers == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "processServers");
}
this.processServers = processServers;
return this;
}
public Builder processServers(ProcessServerDetailsResponse... processServers) {
return processServers(List.of(processServers));
}
@CustomType.Setter
public Builder pushInstallers(List pushInstallers) {
if (pushInstallers == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "pushInstallers");
}
this.pushInstallers = pushInstallers;
return this;
}
public Builder pushInstallers(PushInstallerDetailsResponse... pushInstallers) {
return pushInstallers(List.of(pushInstallers));
}
@CustomType.Setter
public Builder rcmProxies(List rcmProxies) {
if (rcmProxies == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "rcmProxies");
}
this.rcmProxies = rcmProxies;
return this;
}
public Builder rcmProxies(RcmProxyDetailsResponse... rcmProxies) {
return rcmProxies(List.of(rcmProxies));
}
@CustomType.Setter
public Builder replicationAgents(List replicationAgents) {
if (replicationAgents == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "replicationAgents");
}
this.replicationAgents = replicationAgents;
return this;
}
public Builder replicationAgents(ReplicationAgentDetailsResponse... replicationAgents) {
return replicationAgents(List.of(replicationAgents));
}
@CustomType.Setter
public Builder reprotectAgents(List reprotectAgents) {
if (reprotectAgents == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "reprotectAgents");
}
this.reprotectAgents = reprotectAgents;
return this;
}
public Builder reprotectAgents(ReprotectAgentDetailsResponse... reprotectAgents) {
return reprotectAgents(List.of(reprotectAgents));
}
@CustomType.Setter
public Builder serviceContainerId(String serviceContainerId) {
if (serviceContainerId == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "serviceContainerId");
}
this.serviceContainerId = serviceContainerId;
return this;
}
@CustomType.Setter
public Builder serviceEndpoint(String serviceEndpoint) {
if (serviceEndpoint == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "serviceEndpoint");
}
this.serviceEndpoint = serviceEndpoint;
return this;
}
@CustomType.Setter
public Builder serviceResourceId(String serviceResourceId) {
if (serviceResourceId == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "serviceResourceId");
}
this.serviceResourceId = serviceResourceId;
return this;
}
@CustomType.Setter
public Builder sourceAgentIdentityDetails(@Nullable IdentityProviderDetailsResponse sourceAgentIdentityDetails) {
this.sourceAgentIdentityDetails = sourceAgentIdentityDetails;
return this;
}
@CustomType.Setter
public Builder vmwareSiteId(String vmwareSiteId) {
if (vmwareSiteId == null) {
throw new MissingRequiredPropertyException("InMageRcmFabricSpecificDetailsResponse", "vmwareSiteId");
}
this.vmwareSiteId = vmwareSiteId;
return this;
}
public InMageRcmFabricSpecificDetailsResponse build() {
final var _resultValue = new InMageRcmFabricSpecificDetailsResponse();
_resultValue.agentDetails = agentDetails;
_resultValue.controlPlaneUri = controlPlaneUri;
_resultValue.dataPlaneUri = dataPlaneUri;
_resultValue.dras = dras;
_resultValue.instanceType = instanceType;
_resultValue.marsAgents = marsAgents;
_resultValue.physicalSiteId = physicalSiteId;
_resultValue.processServers = processServers;
_resultValue.pushInstallers = pushInstallers;
_resultValue.rcmProxies = rcmProxies;
_resultValue.replicationAgents = replicationAgents;
_resultValue.reprotectAgents = reprotectAgents;
_resultValue.serviceContainerId = serviceContainerId;
_resultValue.serviceEndpoint = serviceEndpoint;
_resultValue.serviceResourceId = serviceResourceId;
_resultValue.sourceAgentIdentityDetails = sourceAgentIdentityDetails;
_resultValue.vmwareSiteId = vmwareSiteId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy