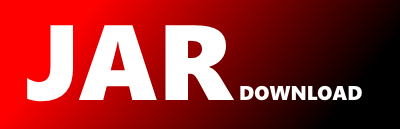
com.pulumi.azurenative.recoveryservices.outputs.InnerHealthErrorResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InnerHealthErrorResponse {
/**
* @return Error creation time (UTC).
*
*/
private @Nullable String creationTimeUtc;
/**
* @return Value indicating whether the health error is customer resolvable.
*
*/
private @Nullable String customerResolvability;
/**
* @return ID of the entity.
*
*/
private @Nullable String entityId;
/**
* @return Category of error.
*
*/
private @Nullable String errorCategory;
/**
* @return Error code.
*
*/
private @Nullable String errorCode;
/**
* @return The health error unique id.
*
*/
private @Nullable String errorId;
/**
* @return Level of error.
*
*/
private @Nullable String errorLevel;
/**
* @return Error message.
*
*/
private @Nullable String errorMessage;
/**
* @return Source of error.
*
*/
private @Nullable String errorSource;
/**
* @return Type of error.
*
*/
private @Nullable String errorType;
/**
* @return Possible causes of error.
*
*/
private @Nullable String possibleCauses;
/**
* @return Recommended action to resolve error.
*
*/
private @Nullable String recommendedAction;
/**
* @return DRA error message.
*
*/
private @Nullable String recoveryProviderErrorMessage;
/**
* @return Summary message of the entity.
*
*/
private @Nullable String summaryMessage;
private InnerHealthErrorResponse() {}
/**
* @return Error creation time (UTC).
*
*/
public Optional creationTimeUtc() {
return Optional.ofNullable(this.creationTimeUtc);
}
/**
* @return Value indicating whether the health error is customer resolvable.
*
*/
public Optional customerResolvability() {
return Optional.ofNullable(this.customerResolvability);
}
/**
* @return ID of the entity.
*
*/
public Optional entityId() {
return Optional.ofNullable(this.entityId);
}
/**
* @return Category of error.
*
*/
public Optional errorCategory() {
return Optional.ofNullable(this.errorCategory);
}
/**
* @return Error code.
*
*/
public Optional errorCode() {
return Optional.ofNullable(this.errorCode);
}
/**
* @return The health error unique id.
*
*/
public Optional errorId() {
return Optional.ofNullable(this.errorId);
}
/**
* @return Level of error.
*
*/
public Optional errorLevel() {
return Optional.ofNullable(this.errorLevel);
}
/**
* @return Error message.
*
*/
public Optional errorMessage() {
return Optional.ofNullable(this.errorMessage);
}
/**
* @return Source of error.
*
*/
public Optional errorSource() {
return Optional.ofNullable(this.errorSource);
}
/**
* @return Type of error.
*
*/
public Optional errorType() {
return Optional.ofNullable(this.errorType);
}
/**
* @return Possible causes of error.
*
*/
public Optional possibleCauses() {
return Optional.ofNullable(this.possibleCauses);
}
/**
* @return Recommended action to resolve error.
*
*/
public Optional recommendedAction() {
return Optional.ofNullable(this.recommendedAction);
}
/**
* @return DRA error message.
*
*/
public Optional recoveryProviderErrorMessage() {
return Optional.ofNullable(this.recoveryProviderErrorMessage);
}
/**
* @return Summary message of the entity.
*
*/
public Optional summaryMessage() {
return Optional.ofNullable(this.summaryMessage);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InnerHealthErrorResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String creationTimeUtc;
private @Nullable String customerResolvability;
private @Nullable String entityId;
private @Nullable String errorCategory;
private @Nullable String errorCode;
private @Nullable String errorId;
private @Nullable String errorLevel;
private @Nullable String errorMessage;
private @Nullable String errorSource;
private @Nullable String errorType;
private @Nullable String possibleCauses;
private @Nullable String recommendedAction;
private @Nullable String recoveryProviderErrorMessage;
private @Nullable String summaryMessage;
public Builder() {}
public Builder(InnerHealthErrorResponse defaults) {
Objects.requireNonNull(defaults);
this.creationTimeUtc = defaults.creationTimeUtc;
this.customerResolvability = defaults.customerResolvability;
this.entityId = defaults.entityId;
this.errorCategory = defaults.errorCategory;
this.errorCode = defaults.errorCode;
this.errorId = defaults.errorId;
this.errorLevel = defaults.errorLevel;
this.errorMessage = defaults.errorMessage;
this.errorSource = defaults.errorSource;
this.errorType = defaults.errorType;
this.possibleCauses = defaults.possibleCauses;
this.recommendedAction = defaults.recommendedAction;
this.recoveryProviderErrorMessage = defaults.recoveryProviderErrorMessage;
this.summaryMessage = defaults.summaryMessage;
}
@CustomType.Setter
public Builder creationTimeUtc(@Nullable String creationTimeUtc) {
this.creationTimeUtc = creationTimeUtc;
return this;
}
@CustomType.Setter
public Builder customerResolvability(@Nullable String customerResolvability) {
this.customerResolvability = customerResolvability;
return this;
}
@CustomType.Setter
public Builder entityId(@Nullable String entityId) {
this.entityId = entityId;
return this;
}
@CustomType.Setter
public Builder errorCategory(@Nullable String errorCategory) {
this.errorCategory = errorCategory;
return this;
}
@CustomType.Setter
public Builder errorCode(@Nullable String errorCode) {
this.errorCode = errorCode;
return this;
}
@CustomType.Setter
public Builder errorId(@Nullable String errorId) {
this.errorId = errorId;
return this;
}
@CustomType.Setter
public Builder errorLevel(@Nullable String errorLevel) {
this.errorLevel = errorLevel;
return this;
}
@CustomType.Setter
public Builder errorMessage(@Nullable String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
@CustomType.Setter
public Builder errorSource(@Nullable String errorSource) {
this.errorSource = errorSource;
return this;
}
@CustomType.Setter
public Builder errorType(@Nullable String errorType) {
this.errorType = errorType;
return this;
}
@CustomType.Setter
public Builder possibleCauses(@Nullable String possibleCauses) {
this.possibleCauses = possibleCauses;
return this;
}
@CustomType.Setter
public Builder recommendedAction(@Nullable String recommendedAction) {
this.recommendedAction = recommendedAction;
return this;
}
@CustomType.Setter
public Builder recoveryProviderErrorMessage(@Nullable String recoveryProviderErrorMessage) {
this.recoveryProviderErrorMessage = recoveryProviderErrorMessage;
return this;
}
@CustomType.Setter
public Builder summaryMessage(@Nullable String summaryMessage) {
this.summaryMessage = summaryMessage;
return this;
}
public InnerHealthErrorResponse build() {
final var _resultValue = new InnerHealthErrorResponse();
_resultValue.creationTimeUtc = creationTimeUtc;
_resultValue.customerResolvability = customerResolvability;
_resultValue.entityId = entityId;
_resultValue.errorCategory = errorCategory;
_resultValue.errorCode = errorCode;
_resultValue.errorId = errorId;
_resultValue.errorLevel = errorLevel;
_resultValue.errorMessage = errorMessage;
_resultValue.errorSource = errorSource;
_resultValue.errorType = errorType;
_resultValue.possibleCauses = possibleCauses;
_resultValue.recommendedAction = recommendedAction;
_resultValue.recoveryProviderErrorMessage = recoveryProviderErrorMessage;
_resultValue.summaryMessage = summaryMessage;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy