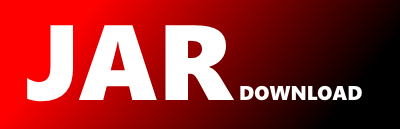
com.pulumi.azurenative.recoveryservices.outputs.ProcessServerDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.HealthErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class ProcessServerDetailsResponse {
/**
* @return The available memory.
*
*/
private Double availableMemoryInBytes;
/**
* @return The available disk space.
*
*/
private Double availableSpaceInBytes;
/**
* @return The process server Bios Id.
*
*/
private String biosId;
/**
* @return The disk usage status.
*
*/
private String diskUsageStatus;
/**
* @return The fabric object Id.
*
*/
private String fabricObjectId;
/**
* @return The process server Fqdn.
*
*/
private String fqdn;
/**
* @return The free disk space percentage.
*
*/
private Double freeSpacePercentage;
/**
* @return The health of the process server.
*
*/
private String health;
/**
* @return The health errors.
*
*/
private List healthErrors;
/**
* @return The historic health of the process server based on the health in last 24 hours.
*
*/
private String historicHealth;
/**
* @return The process server Id.
*
*/
private String id;
/**
* @return The list of IP addresses for communicating with the RCM component.
*
*/
private List ipAddresses;
/**
* @return The last heartbeat received from the process server.
*
*/
private String lastHeartbeatUtc;
/**
* @return The memory usage percentage.
*
*/
private Double memoryUsagePercentage;
/**
* @return The memory usage status.
*
*/
private String memoryUsageStatus;
/**
* @return The process server name.
*
*/
private String name;
/**
* @return The processor usage percentage.
*
*/
private Double processorUsagePercentage;
/**
* @return The processor usage status.
*
*/
private String processorUsageStatus;
/**
* @return The protected item count.
*
*/
private Integer protectedItemCount;
/**
* @return The system load.
*
*/
private Double systemLoad;
/**
* @return The system load status.
*
*/
private String systemLoadStatus;
/**
* @return The throughput in bytes.
*
*/
private Double throughputInBytes;
/**
* @return The throughput status.
*
*/
private String throughputStatus;
/**
* @return The uploading pending data in bytes.
*
*/
private Double throughputUploadPendingDataInBytes;
/**
* @return The total memory.
*
*/
private Double totalMemoryInBytes;
/**
* @return The total disk space.
*
*/
private Double totalSpaceInBytes;
/**
* @return The used memory.
*
*/
private Double usedMemoryInBytes;
/**
* @return The used disk space.
*
*/
private Double usedSpaceInBytes;
/**
* @return The version.
*
*/
private String version;
private ProcessServerDetailsResponse() {}
/**
* @return The available memory.
*
*/
public Double availableMemoryInBytes() {
return this.availableMemoryInBytes;
}
/**
* @return The available disk space.
*
*/
public Double availableSpaceInBytes() {
return this.availableSpaceInBytes;
}
/**
* @return The process server Bios Id.
*
*/
public String biosId() {
return this.biosId;
}
/**
* @return The disk usage status.
*
*/
public String diskUsageStatus() {
return this.diskUsageStatus;
}
/**
* @return The fabric object Id.
*
*/
public String fabricObjectId() {
return this.fabricObjectId;
}
/**
* @return The process server Fqdn.
*
*/
public String fqdn() {
return this.fqdn;
}
/**
* @return The free disk space percentage.
*
*/
public Double freeSpacePercentage() {
return this.freeSpacePercentage;
}
/**
* @return The health of the process server.
*
*/
public String health() {
return this.health;
}
/**
* @return The health errors.
*
*/
public List healthErrors() {
return this.healthErrors;
}
/**
* @return The historic health of the process server based on the health in last 24 hours.
*
*/
public String historicHealth() {
return this.historicHealth;
}
/**
* @return The process server Id.
*
*/
public String id() {
return this.id;
}
/**
* @return The list of IP addresses for communicating with the RCM component.
*
*/
public List ipAddresses() {
return this.ipAddresses;
}
/**
* @return The last heartbeat received from the process server.
*
*/
public String lastHeartbeatUtc() {
return this.lastHeartbeatUtc;
}
/**
* @return The memory usage percentage.
*
*/
public Double memoryUsagePercentage() {
return this.memoryUsagePercentage;
}
/**
* @return The memory usage status.
*
*/
public String memoryUsageStatus() {
return this.memoryUsageStatus;
}
/**
* @return The process server name.
*
*/
public String name() {
return this.name;
}
/**
* @return The processor usage percentage.
*
*/
public Double processorUsagePercentage() {
return this.processorUsagePercentage;
}
/**
* @return The processor usage status.
*
*/
public String processorUsageStatus() {
return this.processorUsageStatus;
}
/**
* @return The protected item count.
*
*/
public Integer protectedItemCount() {
return this.protectedItemCount;
}
/**
* @return The system load.
*
*/
public Double systemLoad() {
return this.systemLoad;
}
/**
* @return The system load status.
*
*/
public String systemLoadStatus() {
return this.systemLoadStatus;
}
/**
* @return The throughput in bytes.
*
*/
public Double throughputInBytes() {
return this.throughputInBytes;
}
/**
* @return The throughput status.
*
*/
public String throughputStatus() {
return this.throughputStatus;
}
/**
* @return The uploading pending data in bytes.
*
*/
public Double throughputUploadPendingDataInBytes() {
return this.throughputUploadPendingDataInBytes;
}
/**
* @return The total memory.
*
*/
public Double totalMemoryInBytes() {
return this.totalMemoryInBytes;
}
/**
* @return The total disk space.
*
*/
public Double totalSpaceInBytes() {
return this.totalSpaceInBytes;
}
/**
* @return The used memory.
*
*/
public Double usedMemoryInBytes() {
return this.usedMemoryInBytes;
}
/**
* @return The used disk space.
*
*/
public Double usedSpaceInBytes() {
return this.usedSpaceInBytes;
}
/**
* @return The version.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProcessServerDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double availableMemoryInBytes;
private Double availableSpaceInBytes;
private String biosId;
private String diskUsageStatus;
private String fabricObjectId;
private String fqdn;
private Double freeSpacePercentage;
private String health;
private List healthErrors;
private String historicHealth;
private String id;
private List ipAddresses;
private String lastHeartbeatUtc;
private Double memoryUsagePercentage;
private String memoryUsageStatus;
private String name;
private Double processorUsagePercentage;
private String processorUsageStatus;
private Integer protectedItemCount;
private Double systemLoad;
private String systemLoadStatus;
private Double throughputInBytes;
private String throughputStatus;
private Double throughputUploadPendingDataInBytes;
private Double totalMemoryInBytes;
private Double totalSpaceInBytes;
private Double usedMemoryInBytes;
private Double usedSpaceInBytes;
private String version;
public Builder() {}
public Builder(ProcessServerDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.availableMemoryInBytes = defaults.availableMemoryInBytes;
this.availableSpaceInBytes = defaults.availableSpaceInBytes;
this.biosId = defaults.biosId;
this.diskUsageStatus = defaults.diskUsageStatus;
this.fabricObjectId = defaults.fabricObjectId;
this.fqdn = defaults.fqdn;
this.freeSpacePercentage = defaults.freeSpacePercentage;
this.health = defaults.health;
this.healthErrors = defaults.healthErrors;
this.historicHealth = defaults.historicHealth;
this.id = defaults.id;
this.ipAddresses = defaults.ipAddresses;
this.lastHeartbeatUtc = defaults.lastHeartbeatUtc;
this.memoryUsagePercentage = defaults.memoryUsagePercentage;
this.memoryUsageStatus = defaults.memoryUsageStatus;
this.name = defaults.name;
this.processorUsagePercentage = defaults.processorUsagePercentage;
this.processorUsageStatus = defaults.processorUsageStatus;
this.protectedItemCount = defaults.protectedItemCount;
this.systemLoad = defaults.systemLoad;
this.systemLoadStatus = defaults.systemLoadStatus;
this.throughputInBytes = defaults.throughputInBytes;
this.throughputStatus = defaults.throughputStatus;
this.throughputUploadPendingDataInBytes = defaults.throughputUploadPendingDataInBytes;
this.totalMemoryInBytes = defaults.totalMemoryInBytes;
this.totalSpaceInBytes = defaults.totalSpaceInBytes;
this.usedMemoryInBytes = defaults.usedMemoryInBytes;
this.usedSpaceInBytes = defaults.usedSpaceInBytes;
this.version = defaults.version;
}
@CustomType.Setter
public Builder availableMemoryInBytes(Double availableMemoryInBytes) {
if (availableMemoryInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "availableMemoryInBytes");
}
this.availableMemoryInBytes = availableMemoryInBytes;
return this;
}
@CustomType.Setter
public Builder availableSpaceInBytes(Double availableSpaceInBytes) {
if (availableSpaceInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "availableSpaceInBytes");
}
this.availableSpaceInBytes = availableSpaceInBytes;
return this;
}
@CustomType.Setter
public Builder biosId(String biosId) {
if (biosId == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "biosId");
}
this.biosId = biosId;
return this;
}
@CustomType.Setter
public Builder diskUsageStatus(String diskUsageStatus) {
if (diskUsageStatus == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "diskUsageStatus");
}
this.diskUsageStatus = diskUsageStatus;
return this;
}
@CustomType.Setter
public Builder fabricObjectId(String fabricObjectId) {
if (fabricObjectId == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "fabricObjectId");
}
this.fabricObjectId = fabricObjectId;
return this;
}
@CustomType.Setter
public Builder fqdn(String fqdn) {
if (fqdn == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "fqdn");
}
this.fqdn = fqdn;
return this;
}
@CustomType.Setter
public Builder freeSpacePercentage(Double freeSpacePercentage) {
if (freeSpacePercentage == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "freeSpacePercentage");
}
this.freeSpacePercentage = freeSpacePercentage;
return this;
}
@CustomType.Setter
public Builder health(String health) {
if (health == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "health");
}
this.health = health;
return this;
}
@CustomType.Setter
public Builder healthErrors(List healthErrors) {
if (healthErrors == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "healthErrors");
}
this.healthErrors = healthErrors;
return this;
}
public Builder healthErrors(HealthErrorResponse... healthErrors) {
return healthErrors(List.of(healthErrors));
}
@CustomType.Setter
public Builder historicHealth(String historicHealth) {
if (historicHealth == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "historicHealth");
}
this.historicHealth = historicHealth;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipAddresses(List ipAddresses) {
if (ipAddresses == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "ipAddresses");
}
this.ipAddresses = ipAddresses;
return this;
}
public Builder ipAddresses(String... ipAddresses) {
return ipAddresses(List.of(ipAddresses));
}
@CustomType.Setter
public Builder lastHeartbeatUtc(String lastHeartbeatUtc) {
if (lastHeartbeatUtc == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "lastHeartbeatUtc");
}
this.lastHeartbeatUtc = lastHeartbeatUtc;
return this;
}
@CustomType.Setter
public Builder memoryUsagePercentage(Double memoryUsagePercentage) {
if (memoryUsagePercentage == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "memoryUsagePercentage");
}
this.memoryUsagePercentage = memoryUsagePercentage;
return this;
}
@CustomType.Setter
public Builder memoryUsageStatus(String memoryUsageStatus) {
if (memoryUsageStatus == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "memoryUsageStatus");
}
this.memoryUsageStatus = memoryUsageStatus;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder processorUsagePercentage(Double processorUsagePercentage) {
if (processorUsagePercentage == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "processorUsagePercentage");
}
this.processorUsagePercentage = processorUsagePercentage;
return this;
}
@CustomType.Setter
public Builder processorUsageStatus(String processorUsageStatus) {
if (processorUsageStatus == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "processorUsageStatus");
}
this.processorUsageStatus = processorUsageStatus;
return this;
}
@CustomType.Setter
public Builder protectedItemCount(Integer protectedItemCount) {
if (protectedItemCount == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "protectedItemCount");
}
this.protectedItemCount = protectedItemCount;
return this;
}
@CustomType.Setter
public Builder systemLoad(Double systemLoad) {
if (systemLoad == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "systemLoad");
}
this.systemLoad = systemLoad;
return this;
}
@CustomType.Setter
public Builder systemLoadStatus(String systemLoadStatus) {
if (systemLoadStatus == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "systemLoadStatus");
}
this.systemLoadStatus = systemLoadStatus;
return this;
}
@CustomType.Setter
public Builder throughputInBytes(Double throughputInBytes) {
if (throughputInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "throughputInBytes");
}
this.throughputInBytes = throughputInBytes;
return this;
}
@CustomType.Setter
public Builder throughputStatus(String throughputStatus) {
if (throughputStatus == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "throughputStatus");
}
this.throughputStatus = throughputStatus;
return this;
}
@CustomType.Setter
public Builder throughputUploadPendingDataInBytes(Double throughputUploadPendingDataInBytes) {
if (throughputUploadPendingDataInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "throughputUploadPendingDataInBytes");
}
this.throughputUploadPendingDataInBytes = throughputUploadPendingDataInBytes;
return this;
}
@CustomType.Setter
public Builder totalMemoryInBytes(Double totalMemoryInBytes) {
if (totalMemoryInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "totalMemoryInBytes");
}
this.totalMemoryInBytes = totalMemoryInBytes;
return this;
}
@CustomType.Setter
public Builder totalSpaceInBytes(Double totalSpaceInBytes) {
if (totalSpaceInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "totalSpaceInBytes");
}
this.totalSpaceInBytes = totalSpaceInBytes;
return this;
}
@CustomType.Setter
public Builder usedMemoryInBytes(Double usedMemoryInBytes) {
if (usedMemoryInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "usedMemoryInBytes");
}
this.usedMemoryInBytes = usedMemoryInBytes;
return this;
}
@CustomType.Setter
public Builder usedSpaceInBytes(Double usedSpaceInBytes) {
if (usedSpaceInBytes == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "usedSpaceInBytes");
}
this.usedSpaceInBytes = usedSpaceInBytes;
return this;
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("ProcessServerDetailsResponse", "version");
}
this.version = version;
return this;
}
public ProcessServerDetailsResponse build() {
final var _resultValue = new ProcessServerDetailsResponse();
_resultValue.availableMemoryInBytes = availableMemoryInBytes;
_resultValue.availableSpaceInBytes = availableSpaceInBytes;
_resultValue.biosId = biosId;
_resultValue.diskUsageStatus = diskUsageStatus;
_resultValue.fabricObjectId = fabricObjectId;
_resultValue.fqdn = fqdn;
_resultValue.freeSpacePercentage = freeSpacePercentage;
_resultValue.health = health;
_resultValue.healthErrors = healthErrors;
_resultValue.historicHealth = historicHealth;
_resultValue.id = id;
_resultValue.ipAddresses = ipAddresses;
_resultValue.lastHeartbeatUtc = lastHeartbeatUtc;
_resultValue.memoryUsagePercentage = memoryUsagePercentage;
_resultValue.memoryUsageStatus = memoryUsageStatus;
_resultValue.name = name;
_resultValue.processorUsagePercentage = processorUsagePercentage;
_resultValue.processorUsageStatus = processorUsageStatus;
_resultValue.protectedItemCount = protectedItemCount;
_resultValue.systemLoad = systemLoad;
_resultValue.systemLoadStatus = systemLoadStatus;
_resultValue.throughputInBytes = throughputInBytes;
_resultValue.throughputStatus = throughputStatus;
_resultValue.throughputUploadPendingDataInBytes = throughputUploadPendingDataInBytes;
_resultValue.totalMemoryInBytes = totalMemoryInBytes;
_resultValue.totalSpaceInBytes = totalSpaceInBytes;
_resultValue.usedMemoryInBytes = usedMemoryInBytes;
_resultValue.usedSpaceInBytes = usedSpaceInBytes;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy