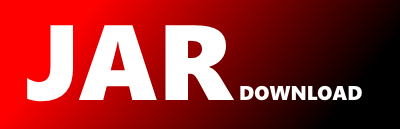
com.pulumi.azurenative.recoveryservices.outputs.RcmProxyDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.HealthErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class RcmProxyDetailsResponse {
/**
* @return The RCM proxy Bios Id.
*
*/
private String biosId;
/**
* @return The client authentication type.
*
*/
private String clientAuthenticationType;
/**
* @return The fabric object Id.
*
*/
private String fabricObjectId;
/**
* @return The RCM proxy Fqdn.
*
*/
private String fqdn;
/**
* @return The health of the RCM proxy.
*
*/
private String health;
/**
* @return The health errors.
*
*/
private List healthErrors;
/**
* @return The RCM proxy Id.
*
*/
private String id;
/**
* @return The last heartbeat received from the RCM proxy.
*
*/
private String lastHeartbeatUtc;
/**
* @return The RCM proxy name.
*
*/
private String name;
/**
* @return The version.
*
*/
private String version;
private RcmProxyDetailsResponse() {}
/**
* @return The RCM proxy Bios Id.
*
*/
public String biosId() {
return this.biosId;
}
/**
* @return The client authentication type.
*
*/
public String clientAuthenticationType() {
return this.clientAuthenticationType;
}
/**
* @return The fabric object Id.
*
*/
public String fabricObjectId() {
return this.fabricObjectId;
}
/**
* @return The RCM proxy Fqdn.
*
*/
public String fqdn() {
return this.fqdn;
}
/**
* @return The health of the RCM proxy.
*
*/
public String health() {
return this.health;
}
/**
* @return The health errors.
*
*/
public List healthErrors() {
return this.healthErrors;
}
/**
* @return The RCM proxy Id.
*
*/
public String id() {
return this.id;
}
/**
* @return The last heartbeat received from the RCM proxy.
*
*/
public String lastHeartbeatUtc() {
return this.lastHeartbeatUtc;
}
/**
* @return The RCM proxy name.
*
*/
public String name() {
return this.name;
}
/**
* @return The version.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RcmProxyDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String biosId;
private String clientAuthenticationType;
private String fabricObjectId;
private String fqdn;
private String health;
private List healthErrors;
private String id;
private String lastHeartbeatUtc;
private String name;
private String version;
public Builder() {}
public Builder(RcmProxyDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.biosId = defaults.biosId;
this.clientAuthenticationType = defaults.clientAuthenticationType;
this.fabricObjectId = defaults.fabricObjectId;
this.fqdn = defaults.fqdn;
this.health = defaults.health;
this.healthErrors = defaults.healthErrors;
this.id = defaults.id;
this.lastHeartbeatUtc = defaults.lastHeartbeatUtc;
this.name = defaults.name;
this.version = defaults.version;
}
@CustomType.Setter
public Builder biosId(String biosId) {
if (biosId == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "biosId");
}
this.biosId = biosId;
return this;
}
@CustomType.Setter
public Builder clientAuthenticationType(String clientAuthenticationType) {
if (clientAuthenticationType == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "clientAuthenticationType");
}
this.clientAuthenticationType = clientAuthenticationType;
return this;
}
@CustomType.Setter
public Builder fabricObjectId(String fabricObjectId) {
if (fabricObjectId == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "fabricObjectId");
}
this.fabricObjectId = fabricObjectId;
return this;
}
@CustomType.Setter
public Builder fqdn(String fqdn) {
if (fqdn == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "fqdn");
}
this.fqdn = fqdn;
return this;
}
@CustomType.Setter
public Builder health(String health) {
if (health == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "health");
}
this.health = health;
return this;
}
@CustomType.Setter
public Builder healthErrors(List healthErrors) {
if (healthErrors == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "healthErrors");
}
this.healthErrors = healthErrors;
return this;
}
public Builder healthErrors(HealthErrorResponse... healthErrors) {
return healthErrors(List.of(healthErrors));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastHeartbeatUtc(String lastHeartbeatUtc) {
if (lastHeartbeatUtc == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "lastHeartbeatUtc");
}
this.lastHeartbeatUtc = lastHeartbeatUtc;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("RcmProxyDetailsResponse", "version");
}
this.version = version;
return this;
}
public RcmProxyDetailsResponse build() {
final var _resultValue = new RcmProxyDetailsResponse();
_resultValue.biosId = biosId;
_resultValue.clientAuthenticationType = clientAuthenticationType;
_resultValue.fabricObjectId = fabricObjectId;
_resultValue.fqdn = fqdn;
_resultValue.health = health;
_resultValue.healthErrors = healthErrors;
_resultValue.id = id;
_resultValue.lastHeartbeatUtc = lastHeartbeatUtc;
_resultValue.name = name;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy