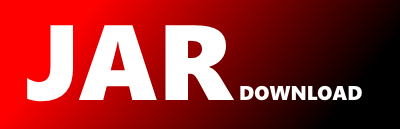
com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtNicDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VMwareCbtNicDetailsResponse {
/**
* @return A value indicating whether this is the primary NIC.
*
*/
private @Nullable String isPrimaryNic;
/**
* @return A value indicating whether this NIC is selected for migration.
*
*/
private @Nullable String isSelectedForMigration;
/**
* @return The NIC Id.
*
*/
private String nicId;
/**
* @return The source IP address.
*
*/
private String sourceIPAddress;
/**
* @return The source IP address type.
*
*/
private String sourceIPAddressType;
/**
* @return Source network Id.
*
*/
private String sourceNetworkId;
/**
* @return The target IP address.
*
*/
private @Nullable String targetIPAddress;
/**
* @return The target IP address type.
*
*/
private @Nullable String targetIPAddressType;
/**
* @return Target NIC name.
*
*/
private @Nullable String targetNicName;
/**
* @return Target subnet name.
*
*/
private @Nullable String targetSubnetName;
/**
* @return The test IP address.
*
*/
private @Nullable String testIPAddress;
/**
* @return The test IP address type.
*
*/
private @Nullable String testIPAddressType;
/**
* @return Source network Id.
*
*/
private @Nullable String testNetworkId;
/**
* @return Test subnet name.
*
*/
private @Nullable String testSubnetName;
private VMwareCbtNicDetailsResponse() {}
/**
* @return A value indicating whether this is the primary NIC.
*
*/
public Optional isPrimaryNic() {
return Optional.ofNullable(this.isPrimaryNic);
}
/**
* @return A value indicating whether this NIC is selected for migration.
*
*/
public Optional isSelectedForMigration() {
return Optional.ofNullable(this.isSelectedForMigration);
}
/**
* @return The NIC Id.
*
*/
public String nicId() {
return this.nicId;
}
/**
* @return The source IP address.
*
*/
public String sourceIPAddress() {
return this.sourceIPAddress;
}
/**
* @return The source IP address type.
*
*/
public String sourceIPAddressType() {
return this.sourceIPAddressType;
}
/**
* @return Source network Id.
*
*/
public String sourceNetworkId() {
return this.sourceNetworkId;
}
/**
* @return The target IP address.
*
*/
public Optional targetIPAddress() {
return Optional.ofNullable(this.targetIPAddress);
}
/**
* @return The target IP address type.
*
*/
public Optional targetIPAddressType() {
return Optional.ofNullable(this.targetIPAddressType);
}
/**
* @return Target NIC name.
*
*/
public Optional targetNicName() {
return Optional.ofNullable(this.targetNicName);
}
/**
* @return Target subnet name.
*
*/
public Optional targetSubnetName() {
return Optional.ofNullable(this.targetSubnetName);
}
/**
* @return The test IP address.
*
*/
public Optional testIPAddress() {
return Optional.ofNullable(this.testIPAddress);
}
/**
* @return The test IP address type.
*
*/
public Optional testIPAddressType() {
return Optional.ofNullable(this.testIPAddressType);
}
/**
* @return Source network Id.
*
*/
public Optional testNetworkId() {
return Optional.ofNullable(this.testNetworkId);
}
/**
* @return Test subnet name.
*
*/
public Optional testSubnetName() {
return Optional.ofNullable(this.testSubnetName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareCbtNicDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String isPrimaryNic;
private @Nullable String isSelectedForMigration;
private String nicId;
private String sourceIPAddress;
private String sourceIPAddressType;
private String sourceNetworkId;
private @Nullable String targetIPAddress;
private @Nullable String targetIPAddressType;
private @Nullable String targetNicName;
private @Nullable String targetSubnetName;
private @Nullable String testIPAddress;
private @Nullable String testIPAddressType;
private @Nullable String testNetworkId;
private @Nullable String testSubnetName;
public Builder() {}
public Builder(VMwareCbtNicDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.isPrimaryNic = defaults.isPrimaryNic;
this.isSelectedForMigration = defaults.isSelectedForMigration;
this.nicId = defaults.nicId;
this.sourceIPAddress = defaults.sourceIPAddress;
this.sourceIPAddressType = defaults.sourceIPAddressType;
this.sourceNetworkId = defaults.sourceNetworkId;
this.targetIPAddress = defaults.targetIPAddress;
this.targetIPAddressType = defaults.targetIPAddressType;
this.targetNicName = defaults.targetNicName;
this.targetSubnetName = defaults.targetSubnetName;
this.testIPAddress = defaults.testIPAddress;
this.testIPAddressType = defaults.testIPAddressType;
this.testNetworkId = defaults.testNetworkId;
this.testSubnetName = defaults.testSubnetName;
}
@CustomType.Setter
public Builder isPrimaryNic(@Nullable String isPrimaryNic) {
this.isPrimaryNic = isPrimaryNic;
return this;
}
@CustomType.Setter
public Builder isSelectedForMigration(@Nullable String isSelectedForMigration) {
this.isSelectedForMigration = isSelectedForMigration;
return this;
}
@CustomType.Setter
public Builder nicId(String nicId) {
if (nicId == null) {
throw new MissingRequiredPropertyException("VMwareCbtNicDetailsResponse", "nicId");
}
this.nicId = nicId;
return this;
}
@CustomType.Setter
public Builder sourceIPAddress(String sourceIPAddress) {
if (sourceIPAddress == null) {
throw new MissingRequiredPropertyException("VMwareCbtNicDetailsResponse", "sourceIPAddress");
}
this.sourceIPAddress = sourceIPAddress;
return this;
}
@CustomType.Setter
public Builder sourceIPAddressType(String sourceIPAddressType) {
if (sourceIPAddressType == null) {
throw new MissingRequiredPropertyException("VMwareCbtNicDetailsResponse", "sourceIPAddressType");
}
this.sourceIPAddressType = sourceIPAddressType;
return this;
}
@CustomType.Setter
public Builder sourceNetworkId(String sourceNetworkId) {
if (sourceNetworkId == null) {
throw new MissingRequiredPropertyException("VMwareCbtNicDetailsResponse", "sourceNetworkId");
}
this.sourceNetworkId = sourceNetworkId;
return this;
}
@CustomType.Setter
public Builder targetIPAddress(@Nullable String targetIPAddress) {
this.targetIPAddress = targetIPAddress;
return this;
}
@CustomType.Setter
public Builder targetIPAddressType(@Nullable String targetIPAddressType) {
this.targetIPAddressType = targetIPAddressType;
return this;
}
@CustomType.Setter
public Builder targetNicName(@Nullable String targetNicName) {
this.targetNicName = targetNicName;
return this;
}
@CustomType.Setter
public Builder targetSubnetName(@Nullable String targetSubnetName) {
this.targetSubnetName = targetSubnetName;
return this;
}
@CustomType.Setter
public Builder testIPAddress(@Nullable String testIPAddress) {
this.testIPAddress = testIPAddress;
return this;
}
@CustomType.Setter
public Builder testIPAddressType(@Nullable String testIPAddressType) {
this.testIPAddressType = testIPAddressType;
return this;
}
@CustomType.Setter
public Builder testNetworkId(@Nullable String testNetworkId) {
this.testNetworkId = testNetworkId;
return this;
}
@CustomType.Setter
public Builder testSubnetName(@Nullable String testSubnetName) {
this.testSubnetName = testSubnetName;
return this;
}
public VMwareCbtNicDetailsResponse build() {
final var _resultValue = new VMwareCbtNicDetailsResponse();
_resultValue.isPrimaryNic = isPrimaryNic;
_resultValue.isSelectedForMigration = isSelectedForMigration;
_resultValue.nicId = nicId;
_resultValue.sourceIPAddress = sourceIPAddress;
_resultValue.sourceIPAddressType = sourceIPAddressType;
_resultValue.sourceNetworkId = sourceNetworkId;
_resultValue.targetIPAddress = targetIPAddress;
_resultValue.targetIPAddressType = targetIPAddressType;
_resultValue.targetNicName = targetNicName;
_resultValue.targetSubnetName = targetSubnetName;
_resultValue.testIPAddress = testIPAddress;
_resultValue.testIPAddressType = testIPAddressType;
_resultValue.testNetworkId = testNetworkId;
_resultValue.testSubnetName = testSubnetName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy