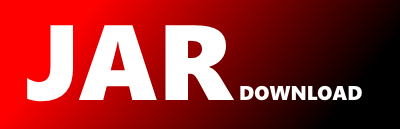
com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtProtectionContainerMappingDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class VMwareCbtProtectionContainerMappingDetailsResponse {
/**
* @return The SKUs to be excluded.
*
*/
private @Nullable List excludedSkus;
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'VMwareCbt'.
*
*/
private String instanceType;
/**
* @return The target key vault ARM Id.
*
*/
private String keyVaultId;
/**
* @return The target key vault URI.
*
*/
private String keyVaultUri;
/**
* @return The role size to NIC count map.
*
*/
private Map roleSizeToNicCountMap;
/**
* @return The secret name of the service bus connection string.
*
*/
private String serviceBusConnectionStringSecretName;
/**
* @return The storage account ARM Id.
*
*/
private String storageAccountId;
/**
* @return The secret name of the storage account.
*
*/
private String storageAccountSasSecretName;
/**
* @return The target location.
*
*/
private String targetLocation;
private VMwareCbtProtectionContainerMappingDetailsResponse() {}
/**
* @return The SKUs to be excluded.
*
*/
public List excludedSkus() {
return this.excludedSkus == null ? List.of() : this.excludedSkus;
}
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'VMwareCbt'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The target key vault ARM Id.
*
*/
public String keyVaultId() {
return this.keyVaultId;
}
/**
* @return The target key vault URI.
*
*/
public String keyVaultUri() {
return this.keyVaultUri;
}
/**
* @return The role size to NIC count map.
*
*/
public Map roleSizeToNicCountMap() {
return this.roleSizeToNicCountMap;
}
/**
* @return The secret name of the service bus connection string.
*
*/
public String serviceBusConnectionStringSecretName() {
return this.serviceBusConnectionStringSecretName;
}
/**
* @return The storage account ARM Id.
*
*/
public String storageAccountId() {
return this.storageAccountId;
}
/**
* @return The secret name of the storage account.
*
*/
public String storageAccountSasSecretName() {
return this.storageAccountSasSecretName;
}
/**
* @return The target location.
*
*/
public String targetLocation() {
return this.targetLocation;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareCbtProtectionContainerMappingDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List excludedSkus;
private String instanceType;
private String keyVaultId;
private String keyVaultUri;
private Map roleSizeToNicCountMap;
private String serviceBusConnectionStringSecretName;
private String storageAccountId;
private String storageAccountSasSecretName;
private String targetLocation;
public Builder() {}
public Builder(VMwareCbtProtectionContainerMappingDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.excludedSkus = defaults.excludedSkus;
this.instanceType = defaults.instanceType;
this.keyVaultId = defaults.keyVaultId;
this.keyVaultUri = defaults.keyVaultUri;
this.roleSizeToNicCountMap = defaults.roleSizeToNicCountMap;
this.serviceBusConnectionStringSecretName = defaults.serviceBusConnectionStringSecretName;
this.storageAccountId = defaults.storageAccountId;
this.storageAccountSasSecretName = defaults.storageAccountSasSecretName;
this.targetLocation = defaults.targetLocation;
}
@CustomType.Setter
public Builder excludedSkus(@Nullable List excludedSkus) {
this.excludedSkus = excludedSkus;
return this;
}
public Builder excludedSkus(String... excludedSkus) {
return excludedSkus(List.of(excludedSkus));
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder keyVaultId(String keyVaultId) {
if (keyVaultId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "keyVaultId");
}
this.keyVaultId = keyVaultId;
return this;
}
@CustomType.Setter
public Builder keyVaultUri(String keyVaultUri) {
if (keyVaultUri == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "keyVaultUri");
}
this.keyVaultUri = keyVaultUri;
return this;
}
@CustomType.Setter
public Builder roleSizeToNicCountMap(Map roleSizeToNicCountMap) {
if (roleSizeToNicCountMap == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "roleSizeToNicCountMap");
}
this.roleSizeToNicCountMap = roleSizeToNicCountMap;
return this;
}
@CustomType.Setter
public Builder serviceBusConnectionStringSecretName(String serviceBusConnectionStringSecretName) {
if (serviceBusConnectionStringSecretName == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "serviceBusConnectionStringSecretName");
}
this.serviceBusConnectionStringSecretName = serviceBusConnectionStringSecretName;
return this;
}
@CustomType.Setter
public Builder storageAccountId(String storageAccountId) {
if (storageAccountId == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "storageAccountId");
}
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder storageAccountSasSecretName(String storageAccountSasSecretName) {
if (storageAccountSasSecretName == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "storageAccountSasSecretName");
}
this.storageAccountSasSecretName = storageAccountSasSecretName;
return this;
}
@CustomType.Setter
public Builder targetLocation(String targetLocation) {
if (targetLocation == null) {
throw new MissingRequiredPropertyException("VMwareCbtProtectionContainerMappingDetailsResponse", "targetLocation");
}
this.targetLocation = targetLocation;
return this;
}
public VMwareCbtProtectionContainerMappingDetailsResponse build() {
final var _resultValue = new VMwareCbtProtectionContainerMappingDetailsResponse();
_resultValue.excludedSkus = excludedSkus;
_resultValue.instanceType = instanceType;
_resultValue.keyVaultId = keyVaultId;
_resultValue.keyVaultUri = keyVaultUri;
_resultValue.roleSizeToNicCountMap = roleSizeToNicCountMap;
_resultValue.serviceBusConnectionStringSecretName = serviceBusConnectionStringSecretName;
_resultValue.storageAccountId = storageAccountId;
_resultValue.storageAccountSasSecretName = storageAccountSasSecretName;
_resultValue.targetLocation = targetLocation;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy