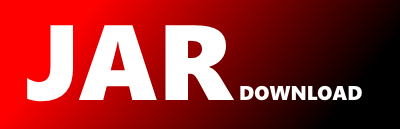
com.pulumi.azurenative.resources.DeploymentStackAtManagementGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.resources.DeploymentStackAtManagementGroupArgs;
import com.pulumi.azurenative.resources.outputs.DenySettingsResponse;
import com.pulumi.azurenative.resources.outputs.DeploymentStackPropertiesResponseActionOnUnmanage;
import com.pulumi.azurenative.resources.outputs.DeploymentStacksDebugSettingResponse;
import com.pulumi.azurenative.resources.outputs.DeploymentStacksParametersLinkResponse;
import com.pulumi.azurenative.resources.outputs.ErrorResponseResponse;
import com.pulumi.azurenative.resources.outputs.ManagedResourceReferenceResponse;
import com.pulumi.azurenative.resources.outputs.ResourceReferenceExtendedResponse;
import com.pulumi.azurenative.resources.outputs.ResourceReferenceResponse;
import com.pulumi.azurenative.resources.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Deployment stack object.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
* ## Example Usage
* ### DeploymentStacksCreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.resources.DeploymentStackAtManagementGroup;
* import com.pulumi.azurenative.resources.DeploymentStackAtManagementGroupArgs;
* import com.pulumi.azurenative.resources.inputs.DeploymentStackPropertiesActionOnUnmanageArgs;
* import com.pulumi.azurenative.resources.inputs.DenySettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var deploymentStackAtManagementGroup = new DeploymentStackAtManagementGroup("deploymentStackAtManagementGroup", DeploymentStackAtManagementGroupArgs.builder()
* .actionOnUnmanage(DeploymentStackPropertiesActionOnUnmanageArgs.builder()
* .managementGroups("detach")
* .resourceGroups("delete")
* .resources("delete")
* .build())
* .denySettings(DenySettingsArgs.builder()
* .applyToChildScopes(false)
* .excludedActions("action")
* .excludedPrincipals("principal")
* .mode("denyDelete")
* .build())
* .deploymentStackName("simpleDeploymentStack")
* .location("eastus")
* .managementGroupId("myMg")
* .parameters(Map.of("parameter1", Map.of("value", "a string")))
* .tags(Map.of("tagkey", "tagVal"))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:resources:DeploymentStackAtManagementGroup simpleDeploymentStack /providers/Microsoft.Management/managementGroups/{managementGroupId}/providers/Microsoft.Resources/deploymentStacks/{deploymentStackName}
* ```
*
*/
@ResourceType(type="azure-native:resources:DeploymentStackAtManagementGroup")
public class DeploymentStackAtManagementGroup extends com.pulumi.resources.CustomResource {
/**
* Defines the behavior of resources that are not managed immediately after the stack is updated.
*
*/
@Export(name="actionOnUnmanage", refs={DeploymentStackPropertiesResponseActionOnUnmanage.class}, tree="[0]")
private Output actionOnUnmanage;
/**
* @return Defines the behavior of resources that are not managed immediately after the stack is updated.
*
*/
public Output actionOnUnmanage() {
return this.actionOnUnmanage;
}
/**
* The debug setting of the deployment.
*
*/
@Export(name="debugSetting", refs={DeploymentStacksDebugSettingResponse.class}, tree="[0]")
private Output* @Nullable */ DeploymentStacksDebugSettingResponse> debugSetting;
/**
* @return The debug setting of the deployment.
*
*/
public Output> debugSetting() {
return Codegen.optional(this.debugSetting);
}
/**
* An array of resources that were deleted during the most recent update.
*
*/
@Export(name="deletedResources", refs={List.class,ResourceReferenceResponse.class}, tree="[0,1]")
private Output> deletedResources;
/**
* @return An array of resources that were deleted during the most recent update.
*
*/
public Output> deletedResources() {
return this.deletedResources;
}
/**
* Defines how resources deployed by the stack are locked.
*
*/
@Export(name="denySettings", refs={DenySettingsResponse.class}, tree="[0]")
private Output denySettings;
/**
* @return Defines how resources deployed by the stack are locked.
*
*/
public Output denySettings() {
return this.denySettings;
}
/**
* The resourceId of the deployment resource created by the deployment stack.
*
*/
@Export(name="deploymentId", refs={String.class}, tree="[0]")
private Output deploymentId;
/**
* @return The resourceId of the deployment resource created by the deployment stack.
*
*/
public Output deploymentId() {
return this.deploymentId;
}
/**
* The scope at which the initial deployment should be created. If a scope is not specified, it will default to the scope of the deployment stack. Valid scopes are: management group (format: '/providers/Microsoft.Management/managementGroups/{managementGroupId}'), subscription (format: '/subscriptions/{subscriptionId}'), resource group (format: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}').
*
*/
@Export(name="deploymentScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deploymentScope;
/**
* @return The scope at which the initial deployment should be created. If a scope is not specified, it will default to the scope of the deployment stack. Valid scopes are: management group (format: '/providers/Microsoft.Management/managementGroups/{managementGroupId}'), subscription (format: '/subscriptions/{subscriptionId}'), resource group (format: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}').
*
*/
public Output> deploymentScope() {
return Codegen.optional(this.deploymentScope);
}
/**
* Deployment stack description.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Deployment stack description.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* An array of resources that were detached during the most recent update.
*
*/
@Export(name="detachedResources", refs={List.class,ResourceReferenceResponse.class}, tree="[0,1]")
private Output> detachedResources;
/**
* @return An array of resources that were detached during the most recent update.
*
*/
public Output> detachedResources() {
return this.detachedResources;
}
/**
* The duration of the deployment stack update.
*
*/
@Export(name="duration", refs={String.class}, tree="[0]")
private Output duration;
/**
* @return The duration of the deployment stack update.
*
*/
public Output duration() {
return this.duration;
}
/**
* Common error response for all Azure Resource Manager APIs to return error details for failed operations. (This also follows the OData error response format.).
*
*/
@Export(name="error", refs={ErrorResponseResponse.class}, tree="[0]")
private Output* @Nullable */ ErrorResponseResponse> error;
/**
* @return Common error response for all Azure Resource Manager APIs to return error details for failed operations. (This also follows the OData error response format.).
*
*/
public Output> error() {
return Codegen.optional(this.error);
}
/**
* An array of resources that failed to reach goal state during the most recent update.
*
*/
@Export(name="failedResources", refs={List.class,ResourceReferenceExtendedResponse.class}, tree="[0,1]")
private Output> failedResources;
/**
* @return An array of resources that failed to reach goal state during the most recent update.
*
*/
public Output> failedResources() {
return this.failedResources;
}
/**
* The location of the deployment stack. It cannot be changed after creation. It must be one of the supported Azure locations.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The location of the deployment stack. It cannot be changed after creation. It must be one of the supported Azure locations.
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* Name of this resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of this resource.
*
*/
public Output name() {
return this.name;
}
/**
* The outputs of the underlying deployment.
*
*/
@Export(name="outputs", refs={Object.class}, tree="[0]")
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy