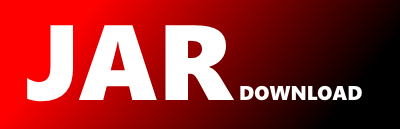
com.pulumi.azurenative.resources.ResourcesFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.resources.inputs.GetAzureCliScriptArgs;
import com.pulumi.azurenative.resources.inputs.GetAzureCliScriptPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetAzurePowerShellScriptArgs;
import com.pulumi.azurenative.resources.inputs.GetAzurePowerShellScriptPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtManagementGroupScopeArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtManagementGroupScopePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtScopeArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtScopePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtSubscriptionScopeArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtSubscriptionScopePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtTenantScopeArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentAtTenantScopePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtManagementGroupArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtManagementGroupPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtResourceGroupArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtResourceGroupPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtSubscriptionArgs;
import com.pulumi.azurenative.resources.inputs.GetDeploymentStackAtSubscriptionPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetResourceArgs;
import com.pulumi.azurenative.resources.inputs.GetResourceGroupArgs;
import com.pulumi.azurenative.resources.inputs.GetResourceGroupPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetResourcePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetTagAtScopeArgs;
import com.pulumi.azurenative.resources.inputs.GetTagAtScopePlainArgs;
import com.pulumi.azurenative.resources.inputs.GetTemplateSpecArgs;
import com.pulumi.azurenative.resources.inputs.GetTemplateSpecPlainArgs;
import com.pulumi.azurenative.resources.inputs.GetTemplateSpecVersionArgs;
import com.pulumi.azurenative.resources.inputs.GetTemplateSpecVersionPlainArgs;
import com.pulumi.azurenative.resources.outputs.GetAzureCliScriptResult;
import com.pulumi.azurenative.resources.outputs.GetAzurePowerShellScriptResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentAtManagementGroupScopeResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentAtScopeResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentAtSubscriptionScopeResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentAtTenantScopeResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentStackAtManagementGroupResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentStackAtResourceGroupResult;
import com.pulumi.azurenative.resources.outputs.GetDeploymentStackAtSubscriptionResult;
import com.pulumi.azurenative.resources.outputs.GetResourceGroupResult;
import com.pulumi.azurenative.resources.outputs.GetResourceResult;
import com.pulumi.azurenative.resources.outputs.GetTagAtScopeResult;
import com.pulumi.azurenative.resources.outputs.GetTemplateSpecResult;
import com.pulumi.azurenative.resources.outputs.GetTemplateSpecVersionResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ResourcesFunctions {
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static Output getAzureCliScript(GetAzureCliScriptArgs args) {
return getAzureCliScript(args, InvokeOptions.Empty);
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static CompletableFuture getAzureCliScriptPlain(GetAzureCliScriptPlainArgs args) {
return getAzureCliScriptPlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static Output getAzureCliScript(GetAzureCliScriptArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getAzureCliScript", TypeShape.of(GetAzureCliScriptResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static CompletableFuture getAzureCliScriptPlain(GetAzureCliScriptPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getAzureCliScript", TypeShape.of(GetAzureCliScriptResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static Output getAzurePowerShellScript(GetAzurePowerShellScriptArgs args) {
return getAzurePowerShellScript(args, InvokeOptions.Empty);
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static CompletableFuture getAzurePowerShellScriptPlain(GetAzurePowerShellScriptPlainArgs args) {
return getAzurePowerShellScriptPlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static Output getAzurePowerShellScript(GetAzurePowerShellScriptArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getAzurePowerShellScript", TypeShape.of(GetAzurePowerShellScriptResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment script with a given name.
* Azure REST API version: 2020-10-01.
*
*/
public static CompletableFuture getAzurePowerShellScriptPlain(GetAzurePowerShellScriptPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getAzurePowerShellScript", TypeShape.of(GetAzurePowerShellScriptResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2016-07-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeployment(GetDeploymentArgs args) {
return getDeployment(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2016-07-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args) {
return getDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2016-07-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeployment(GetDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2016-07-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtManagementGroupScope(GetDeploymentAtManagementGroupScopeArgs args) {
return getDeploymentAtManagementGroupScope(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtManagementGroupScopePlain(GetDeploymentAtManagementGroupScopePlainArgs args) {
return getDeploymentAtManagementGroupScopePlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtManagementGroupScope(GetDeploymentAtManagementGroupScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentAtManagementGroupScope", TypeShape.of(GetDeploymentAtManagementGroupScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtManagementGroupScopePlain(GetDeploymentAtManagementGroupScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentAtManagementGroupScope", TypeShape.of(GetDeploymentAtManagementGroupScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtScope(GetDeploymentAtScopeArgs args) {
return getDeploymentAtScope(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtScopePlain(GetDeploymentAtScopePlainArgs args) {
return getDeploymentAtScopePlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtScope(GetDeploymentAtScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentAtScope", TypeShape.of(GetDeploymentAtScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtScopePlain(GetDeploymentAtScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentAtScope", TypeShape.of(GetDeploymentAtScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtSubscriptionScope(GetDeploymentAtSubscriptionScopeArgs args) {
return getDeploymentAtSubscriptionScope(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtSubscriptionScopePlain(GetDeploymentAtSubscriptionScopePlainArgs args) {
return getDeploymentAtSubscriptionScopePlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtSubscriptionScope(GetDeploymentAtSubscriptionScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentAtSubscriptionScope", TypeShape.of(GetDeploymentAtSubscriptionScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtSubscriptionScopePlain(GetDeploymentAtSubscriptionScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentAtSubscriptionScope", TypeShape.of(GetDeploymentAtSubscriptionScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtTenantScope(GetDeploymentAtTenantScopeArgs args) {
return getDeploymentAtTenantScope(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtTenantScopePlain(GetDeploymentAtTenantScopePlainArgs args) {
return getDeploymentAtTenantScopePlain(args, InvokeOptions.Empty);
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getDeploymentAtTenantScope(GetDeploymentAtTenantScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentAtTenantScope", TypeShape.of(GetDeploymentAtTenantScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a deployment.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getDeploymentAtTenantScopePlain(GetDeploymentAtTenantScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentAtTenantScope", TypeShape.of(GetDeploymentAtTenantScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtManagementGroup(GetDeploymentStackAtManagementGroupArgs args) {
return getDeploymentStackAtManagementGroup(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtManagementGroupPlain(GetDeploymentStackAtManagementGroupPlainArgs args) {
return getDeploymentStackAtManagementGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtManagementGroup(GetDeploymentStackAtManagementGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentStackAtManagementGroup", TypeShape.of(GetDeploymentStackAtManagementGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtManagementGroupPlain(GetDeploymentStackAtManagementGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentStackAtManagementGroup", TypeShape.of(GetDeploymentStackAtManagementGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtResourceGroup(GetDeploymentStackAtResourceGroupArgs args) {
return getDeploymentStackAtResourceGroup(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtResourceGroupPlain(GetDeploymentStackAtResourceGroupPlainArgs args) {
return getDeploymentStackAtResourceGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtResourceGroup(GetDeploymentStackAtResourceGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentStackAtResourceGroup", TypeShape.of(GetDeploymentStackAtResourceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtResourceGroupPlain(GetDeploymentStackAtResourceGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentStackAtResourceGroup", TypeShape.of(GetDeploymentStackAtResourceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtSubscription(GetDeploymentStackAtSubscriptionArgs args) {
return getDeploymentStackAtSubscription(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtSubscriptionPlain(GetDeploymentStackAtSubscriptionPlainArgs args) {
return getDeploymentStackAtSubscriptionPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getDeploymentStackAtSubscription(GetDeploymentStackAtSubscriptionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getDeploymentStackAtSubscription", TypeShape.of(GetDeploymentStackAtSubscriptionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Deployment Stack with a given name.
* Azure REST API version: 2022-08-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getDeploymentStackAtSubscriptionPlain(GetDeploymentStackAtSubscriptionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getDeploymentStackAtSubscription", TypeShape.of(GetDeploymentStackAtSubscriptionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-11-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getResource(GetResourceArgs args) {
return getResource(args, InvokeOptions.Empty);
}
/**
* Gets a resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-11-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getResourcePlain(GetResourcePlainArgs args) {
return getResourcePlain(args, InvokeOptions.Empty);
}
/**
* Gets a resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-11-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getResource(GetResourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getResource", TypeShape.of(GetResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-11-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getResourcePlain(GetResourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getResource", TypeShape.of(GetResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource group.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-02-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getResourceGroup(GetResourceGroupArgs args) {
return getResourceGroup(args, InvokeOptions.Empty);
}
/**
* Gets a resource group.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-02-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getResourceGroupPlain(GetResourceGroupPlainArgs args) {
return getResourceGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets a resource group.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-02-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getResourceGroup(GetResourceGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getResourceGroup", TypeShape.of(GetResourceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a resource group.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-02-01, 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getResourceGroupPlain(GetResourceGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getResourceGroup", TypeShape.of(GetResourceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Wrapper resource for tags API requests and responses.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getTagAtScope(GetTagAtScopeArgs args) {
return getTagAtScope(args, InvokeOptions.Empty);
}
/**
* Wrapper resource for tags API requests and responses.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getTagAtScopePlain(GetTagAtScopePlainArgs args) {
return getTagAtScopePlain(args, InvokeOptions.Empty);
}
/**
* Wrapper resource for tags API requests and responses.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static Output getTagAtScope(GetTagAtScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getTagAtScope", TypeShape.of(GetTagAtScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Wrapper resource for tags API requests and responses.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-07-01, 2024-03-01, 2024-07-01.
*
*/
public static CompletableFuture getTagAtScopePlain(GetTagAtScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getTagAtScope", TypeShape.of(GetTagAtScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Template Spec with a given name.
* Azure REST API version: 2022-02-01.
*
*/
public static Output getTemplateSpec(GetTemplateSpecArgs args) {
return getTemplateSpec(args, InvokeOptions.Empty);
}
/**
* Gets a Template Spec with a given name.
* Azure REST API version: 2022-02-01.
*
*/
public static CompletableFuture getTemplateSpecPlain(GetTemplateSpecPlainArgs args) {
return getTemplateSpecPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Template Spec with a given name.
* Azure REST API version: 2022-02-01.
*
*/
public static Output getTemplateSpec(GetTemplateSpecArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getTemplateSpec", TypeShape.of(GetTemplateSpecResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Template Spec with a given name.
* Azure REST API version: 2022-02-01.
*
*/
public static CompletableFuture getTemplateSpecPlain(GetTemplateSpecPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getTemplateSpec", TypeShape.of(GetTemplateSpecResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Template Spec version from a specific Template Spec.
* Azure REST API version: 2022-02-01.
*
* Other available API versions: 2019-06-01-preview.
*
*/
public static Output getTemplateSpecVersion(GetTemplateSpecVersionArgs args) {
return getTemplateSpecVersion(args, InvokeOptions.Empty);
}
/**
* Gets a Template Spec version from a specific Template Spec.
* Azure REST API version: 2022-02-01.
*
* Other available API versions: 2019-06-01-preview.
*
*/
public static CompletableFuture getTemplateSpecVersionPlain(GetTemplateSpecVersionPlainArgs args) {
return getTemplateSpecVersionPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Template Spec version from a specific Template Spec.
* Azure REST API version: 2022-02-01.
*
* Other available API versions: 2019-06-01-preview.
*
*/
public static Output getTemplateSpecVersion(GetTemplateSpecVersionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:resources:getTemplateSpecVersion", TypeShape.of(GetTemplateSpecVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Template Spec version from a specific Template Spec.
* Azure REST API version: 2022-02-01.
*
* Other available API versions: 2019-06-01-preview.
*
*/
public static CompletableFuture getTemplateSpecVersionPlain(GetTemplateSpecVersionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:resources:getTemplateSpecVersion", TypeShape.of(GetTemplateSpecVersionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy