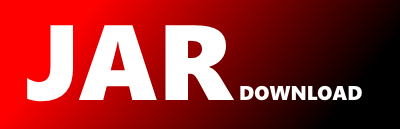
com.pulumi.azurenative.saas.inputs.SaasCreationPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.saas.inputs;
import com.pulumi.azurenative.saas.enums.PaymentChannelType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* properties for creation saas
*
*/
public final class SaasCreationPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final SaasCreationPropertiesArgs Empty = new SaasCreationPropertiesArgs();
/**
* Whether the SaaS subscription will auto renew upon term end.
*
*/
@Import(name="autoRenew")
private @Nullable Output autoRenew;
/**
* @return Whether the SaaS subscription will auto renew upon term end.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy