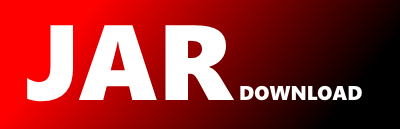
com.pulumi.azurenative.security.CustomRecommendationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security;
import com.pulumi.azurenative.security.enums.RecommendationSupportedClouds;
import com.pulumi.azurenative.security.enums.SecurityIssue;
import com.pulumi.azurenative.security.enums.SeverityEnum;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CustomRecommendationArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomRecommendationArgs Empty = new CustomRecommendationArgs();
/**
* List of all standard supported clouds.
*
*/
@Import(name="cloudProviders")
private @Nullable Output>> cloudProviders;
/**
* @return List of all standard supported clouds.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy