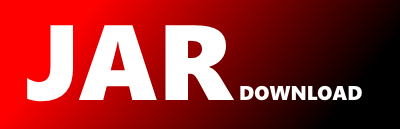
com.pulumi.azurenative.security.Pricing Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.security.PricingArgs;
import com.pulumi.azurenative.security.outputs.ExtensionResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Microsoft Defender for Cloud is provided in two pricing tiers: free and standard. The standard tier offers advanced security capabilities, while the free tier offers basic security features.
* Azure REST API version: 2024-01-01.
*
* ## Example Usage
* ### Update pricing on resource (example for VirtualMachines plan)
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.Pricing;
* import com.pulumi.azurenative.security.PricingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var pricing = new Pricing("pricing", PricingArgs.builder()
* .pricingName("virtualMachines")
* .pricingTier("Standard")
* .scopeId("subscriptions/20ff7fc3-e762-44dd-bd96-b71116dcdc23/resourceGroups/DEMO/providers/Microsoft.Compute/virtualMachines/VM-1")
* .subPlan("P1")
* .build());
*
* }
* }
*
* }
*
* ### Update pricing on subscription (example for CloudPosture plan)
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.Pricing;
* import com.pulumi.azurenative.security.PricingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var pricing = new Pricing("pricing", PricingArgs.builder()
* .pricingName("CloudPosture")
* .pricingTier("Standard")
* .scopeId("subscriptions/20ff7fc3-e762-44dd-bd96-b71116dcdc23")
* .build());
*
* }
* }
*
* }
*
* ### Update pricing on subscription (example for CloudPosture plan) - partial success
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.Pricing;
* import com.pulumi.azurenative.security.PricingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var pricing = new Pricing("pricing", PricingArgs.builder()
* .pricingName("CloudPosture")
* .pricingTier("Standard")
* .scopeId("subscriptions/20ff7fc3-e762-44dd-bd96-b71116dcdc23")
* .build());
*
* }
* }
*
* }
*
* ### Update pricing on subscription (example for VirtualMachines plan)
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.Pricing;
* import com.pulumi.azurenative.security.PricingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var pricing = new Pricing("pricing", PricingArgs.builder()
* .enforce("True")
* .pricingName("VirtualMachines")
* .pricingTier("Standard")
* .scopeId("subscriptions/20ff7fc3-e762-44dd-bd96-b71116dcdc23")
* .subPlan("P2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:security:Pricing VirtualMachines /{scopeId}/providers/Microsoft.Security/pricings/{pricingName}
* ```
*
*/
@ResourceType(type="azure-native:security:Pricing")
public class Pricing extends com.pulumi.resources.CustomResource {
/**
* Optional. True if the plan is deprecated. If there are replacing plans they will appear in `replacedBy` property
*
*/
@Export(name="deprecated", refs={Boolean.class}, tree="[0]")
private Output deprecated;
/**
* @return Optional. True if the plan is deprecated. If there are replacing plans they will appear in `replacedBy` property
*
*/
public Output deprecated() {
return this.deprecated;
}
/**
* Optional. If `pricingTier` is `Standard` then this property holds the date of the last time the `pricingTier` was set to `Standard`, when available (e.g 2023-03-01T12:42:42.1921106Z).
*
*/
@Export(name="enablementTime", refs={String.class}, tree="[0]")
private Output enablementTime;
/**
* @return Optional. If `pricingTier` is `Standard` then this property holds the date of the last time the `pricingTier` was set to `Standard`, when available (e.g 2023-03-01T12:42:42.1921106Z).
*
*/
public Output enablementTime() {
return this.enablementTime;
}
/**
* If set to "False", it allows the descendants of this scope to override the pricing configuration set on this scope (allows setting inherited="False"). If set to "True", it prevents overrides and forces this pricing configuration on all the descendants of this scope. This field is only available for subscription-level pricing.
*
*/
@Export(name="enforce", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> enforce;
/**
* @return If set to "False", it allows the descendants of this scope to override the pricing configuration set on this scope (allows setting inherited="False"). If set to "True", it prevents overrides and forces this pricing configuration on all the descendants of this scope. This field is only available for subscription-level pricing.
*
*/
public Output> enforce() {
return Codegen.optional(this.enforce);
}
/**
* Optional. List of extensions offered under a plan.
*
*/
@Export(name="extensions", refs={List.class,ExtensionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> extensions;
/**
* @return Optional. List of extensions offered under a plan.
*
*/
public Output>> extensions() {
return Codegen.optional(this.extensions);
}
/**
* The duration left for the subscriptions free trial period - in ISO 8601 format (e.g. P3Y6M4DT12H30M5S).
*
*/
@Export(name="freeTrialRemainingTime", refs={String.class}, tree="[0]")
private Output freeTrialRemainingTime;
/**
* @return The duration left for the subscriptions free trial period - in ISO 8601 format (e.g. P3Y6M4DT12H30M5S).
*
*/
public Output freeTrialRemainingTime() {
return this.freeTrialRemainingTime;
}
/**
* "inherited" = "True" indicates that the current scope inherits its pricing configuration from its parent. The ID of the parent scope that provides the inherited configuration is displayed in the "inheritedFrom" field. On the other hand, "inherited" = "False" indicates that the current scope has its own pricing configuration explicitly set, and does not inherit from its parent. This field is read only and available only for resource-level pricing.
*
*/
@Export(name="inherited", refs={String.class}, tree="[0]")
private Output inherited;
/**
* @return "inherited" = "True" indicates that the current scope inherits its pricing configuration from its parent. The ID of the parent scope that provides the inherited configuration is displayed in the "inheritedFrom" field. On the other hand, "inherited" = "False" indicates that the current scope has its own pricing configuration explicitly set, and does not inherit from its parent. This field is read only and available only for resource-level pricing.
*
*/
public Output inherited() {
return this.inherited;
}
/**
* The id of the scope inherited from. "Null" if not inherited. This field is only available for resource-level pricing.
*
*/
@Export(name="inheritedFrom", refs={String.class}, tree="[0]")
private Output inheritedFrom;
/**
* @return The id of the scope inherited from. "Null" if not inherited. This field is only available for resource-level pricing.
*
*/
public Output inheritedFrom() {
return this.inheritedFrom;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Indicates whether the Defender plan is enabled on the selected scope. Microsoft Defender for Cloud is provided in two pricing tiers: free and standard. The standard tier offers advanced security capabilities, while the free tier offers basic security features.
*
*/
@Export(name="pricingTier", refs={String.class}, tree="[0]")
private Output pricingTier;
/**
* @return Indicates whether the Defender plan is enabled on the selected scope. Microsoft Defender for Cloud is provided in two pricing tiers: free and standard. The standard tier offers advanced security capabilities, while the free tier offers basic security features.
*
*/
public Output pricingTier() {
return this.pricingTier;
}
/**
* Optional. List of plans that replace this plan. This property exists only if this plan is deprecated.
*
*/
@Export(name="replacedBy", refs={List.class,String.class}, tree="[0,1]")
private Output> replacedBy;
/**
* @return Optional. List of plans that replace this plan. This property exists only if this plan is deprecated.
*
*/
public Output> replacedBy() {
return this.replacedBy;
}
/**
* This field is available for subscription-level only, and reflects the coverage status of the resources under the subscription. Please note: The "pricingTier" field reflects the plan status of the subscription. However, since the plan status can also be defined at the resource level, there might be misalignment between the subscription's plan status and the resource status. This field helps indicate the coverage status of the resources.
*
*/
@Export(name="resourcesCoverageStatus", refs={String.class}, tree="[0]")
private Output resourcesCoverageStatus;
/**
* @return This field is available for subscription-level only, and reflects the coverage status of the resources under the subscription. Please note: The "pricingTier" field reflects the plan status of the subscription. However, since the plan status can also be defined at the resource level, there might be misalignment between the subscription's plan status and the resource status. This field helps indicate the coverage status of the resources.
*
*/
public Output resourcesCoverageStatus() {
return this.resourcesCoverageStatus;
}
/**
* The sub-plan selected for a Standard pricing configuration, when more than one sub-plan is available. Each sub-plan enables a set of security features. When not specified, full plan is applied. For VirtualMachines plan, available sub plans are 'P1' & 'P2', where for resource level only 'P1' sub plan is supported.
*
*/
@Export(name="subPlan", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> subPlan;
/**
* @return The sub-plan selected for a Standard pricing configuration, when more than one sub-plan is available. Each sub-plan enables a set of security features. When not specified, full plan is applied. For VirtualMachines plan, available sub plans are 'P1' & 'P2', where for resource level only 'P1' sub plan is supported.
*
*/
public Output> subPlan() {
return Codegen.optional(this.subPlan);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Pricing(java.lang.String name) {
this(name, PricingArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Pricing(java.lang.String name, PricingArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Pricing(java.lang.String name, PricingArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:security:Pricing", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Pricing(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:security:Pricing", name, null, makeResourceOptions(options, id), false);
}
private static PricingArgs makeArgs(PricingArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? PricingArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:security/v20240101:Pricing").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Pricing get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Pricing(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy