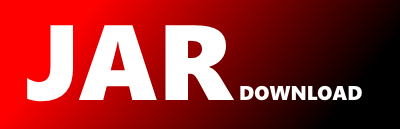
com.pulumi.azurenative.security.inputs.ExtensionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.inputs;
import com.pulumi.azurenative.security.enums.IsEnabled;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A plan's extension properties
*
*/
public final class ExtensionArgs extends com.pulumi.resources.ResourceArgs {
public static final ExtensionArgs Empty = new ExtensionArgs();
/**
* Property values associated with the extension.
*
*/
@Import(name="additionalExtensionProperties")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy