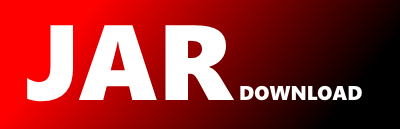
com.pulumi.azurenative.security.inputs.JitNetworkAccessRequestPortArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.inputs;
import com.pulumi.azurenative.security.enums.Status;
import com.pulumi.azurenative.security.enums.StatusReason;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class JitNetworkAccessRequestPortArgs extends com.pulumi.resources.ResourceArgs {
public static final JitNetworkAccessRequestPortArgs Empty = new JitNetworkAccessRequestPortArgs();
/**
* Mutually exclusive with the "allowedSourceAddressPrefixes" parameter. Should be an IP address or CIDR, for example "192.168.0.3" or "192.168.0.0/16".
*
*/
@Import(name="allowedSourceAddressPrefix")
private @Nullable Output allowedSourceAddressPrefix;
/**
* @return Mutually exclusive with the "allowedSourceAddressPrefixes" parameter. Should be an IP address or CIDR, for example "192.168.0.3" or "192.168.0.0/16".
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy