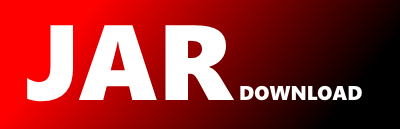
com.pulumi.azurenative.security.inputs.OnPremiseSqlResourceDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
/**
* Details of the On Premise Sql resource that was assessed
*
*/
public final class OnPremiseSqlResourceDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final OnPremiseSqlResourceDetailsArgs Empty = new OnPremiseSqlResourceDetailsArgs();
/**
* The Sql database name installed on the machine
*
*/
@Import(name="databaseName", required=true)
private Output databaseName;
/**
* @return The Sql database name installed on the machine
*
*/
public Output databaseName() {
return this.databaseName;
}
/**
* The name of the machine
*
*/
@Import(name="machineName", required=true)
private Output machineName;
/**
* @return The name of the machine
*
*/
public Output machineName() {
return this.machineName;
}
/**
* The Sql server name installed on the machine
*
*/
@Import(name="serverName", required=true)
private Output serverName;
/**
* @return The Sql server name installed on the machine
*
*/
public Output serverName() {
return this.serverName;
}
/**
* The platform where the assessed resource resides
* Expected value is 'OnPremiseSql'.
*
*/
@Import(name="source", required=true)
private Output source;
/**
* @return The platform where the assessed resource resides
* Expected value is 'OnPremiseSql'.
*
*/
public Output source() {
return this.source;
}
/**
* The oms agent Id installed on the machine
*
*/
@Import(name="sourceComputerId", required=true)
private Output sourceComputerId;
/**
* @return The oms agent Id installed on the machine
*
*/
public Output sourceComputerId() {
return this.sourceComputerId;
}
/**
* The unique Id of the machine
*
*/
@Import(name="vmuuid", required=true)
private Output vmuuid;
/**
* @return The unique Id of the machine
*
*/
public Output vmuuid() {
return this.vmuuid;
}
/**
* Azure resource Id of the workspace the machine is attached to
*
*/
@Import(name="workspaceId", required=true)
private Output workspaceId;
/**
* @return Azure resource Id of the workspace the machine is attached to
*
*/
public Output workspaceId() {
return this.workspaceId;
}
private OnPremiseSqlResourceDetailsArgs() {}
private OnPremiseSqlResourceDetailsArgs(OnPremiseSqlResourceDetailsArgs $) {
this.databaseName = $.databaseName;
this.machineName = $.machineName;
this.serverName = $.serverName;
this.source = $.source;
this.sourceComputerId = $.sourceComputerId;
this.vmuuid = $.vmuuid;
this.workspaceId = $.workspaceId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(OnPremiseSqlResourceDetailsArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private OnPremiseSqlResourceDetailsArgs $;
public Builder() {
$ = new OnPremiseSqlResourceDetailsArgs();
}
public Builder(OnPremiseSqlResourceDetailsArgs defaults) {
$ = new OnPremiseSqlResourceDetailsArgs(Objects.requireNonNull(defaults));
}
/**
* @param databaseName The Sql database name installed on the machine
*
* @return builder
*
*/
public Builder databaseName(Output databaseName) {
$.databaseName = databaseName;
return this;
}
/**
* @param databaseName The Sql database name installed on the machine
*
* @return builder
*
*/
public Builder databaseName(String databaseName) {
return databaseName(Output.of(databaseName));
}
/**
* @param machineName The name of the machine
*
* @return builder
*
*/
public Builder machineName(Output machineName) {
$.machineName = machineName;
return this;
}
/**
* @param machineName The name of the machine
*
* @return builder
*
*/
public Builder machineName(String machineName) {
return machineName(Output.of(machineName));
}
/**
* @param serverName The Sql server name installed on the machine
*
* @return builder
*
*/
public Builder serverName(Output serverName) {
$.serverName = serverName;
return this;
}
/**
* @param serverName The Sql server name installed on the machine
*
* @return builder
*
*/
public Builder serverName(String serverName) {
return serverName(Output.of(serverName));
}
/**
* @param source The platform where the assessed resource resides
* Expected value is 'OnPremiseSql'.
*
* @return builder
*
*/
public Builder source(Output source) {
$.source = source;
return this;
}
/**
* @param source The platform where the assessed resource resides
* Expected value is 'OnPremiseSql'.
*
* @return builder
*
*/
public Builder source(String source) {
return source(Output.of(source));
}
/**
* @param sourceComputerId The oms agent Id installed on the machine
*
* @return builder
*
*/
public Builder sourceComputerId(Output sourceComputerId) {
$.sourceComputerId = sourceComputerId;
return this;
}
/**
* @param sourceComputerId The oms agent Id installed on the machine
*
* @return builder
*
*/
public Builder sourceComputerId(String sourceComputerId) {
return sourceComputerId(Output.of(sourceComputerId));
}
/**
* @param vmuuid The unique Id of the machine
*
* @return builder
*
*/
public Builder vmuuid(Output vmuuid) {
$.vmuuid = vmuuid;
return this;
}
/**
* @param vmuuid The unique Id of the machine
*
* @return builder
*
*/
public Builder vmuuid(String vmuuid) {
return vmuuid(Output.of(vmuuid));
}
/**
* @param workspaceId Azure resource Id of the workspace the machine is attached to
*
* @return builder
*
*/
public Builder workspaceId(Output workspaceId) {
$.workspaceId = workspaceId;
return this;
}
/**
* @param workspaceId Azure resource Id of the workspace the machine is attached to
*
* @return builder
*
*/
public Builder workspaceId(String workspaceId) {
return workspaceId(Output.of(workspaceId));
}
public OnPremiseSqlResourceDetailsArgs build() {
if ($.databaseName == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "databaseName");
}
if ($.machineName == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "machineName");
}
if ($.serverName == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "serverName");
}
$.source = Codegen.stringProp("source").output().arg($.source).require();
if ($.sourceComputerId == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "sourceComputerId");
}
if ($.vmuuid == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "vmuuid");
}
if ($.workspaceId == null) {
throw new MissingRequiredPropertyException("OnPremiseSqlResourceDetailsArgs", "workspaceId");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy