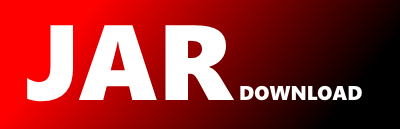
com.pulumi.azurenative.securityinsights.ActivityCustomEntityQueryArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.securityinsights.enums.EntityType;
import com.pulumi.azurenative.securityinsights.inputs.ActivityEntityQueriesPropertiesQueryDefinitionsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ActivityCustomEntityQueryArgs extends com.pulumi.resources.ResourceArgs {
public static final ActivityCustomEntityQueryArgs Empty = new ActivityCustomEntityQueryArgs();
/**
* The entity query content to display in timeline
*
*/
@Import(name="content")
private @Nullable Output content;
/**
* @return The entity query content to display in timeline
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy