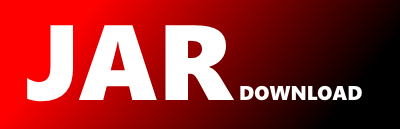
com.pulumi.azurenative.securityinsights.BookmarkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.securityinsights.inputs.IncidentInfoArgs;
import com.pulumi.azurenative.securityinsights.inputs.UserInfoArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BookmarkArgs extends com.pulumi.resources.ResourceArgs {
public static final BookmarkArgs Empty = new BookmarkArgs();
/**
* Bookmark ID
*
*/
@Import(name="bookmarkId")
private @Nullable Output bookmarkId;
/**
* @return Bookmark ID
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy