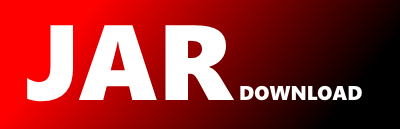
com.pulumi.azurenative.securityinsights.ContentPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.securityinsights.ContentPackageArgs;
import com.pulumi.azurenative.securityinsights.outputs.MetadataAuthorResponse;
import com.pulumi.azurenative.securityinsights.outputs.MetadataCategoriesResponse;
import com.pulumi.azurenative.securityinsights.outputs.MetadataDependenciesResponse;
import com.pulumi.azurenative.securityinsights.outputs.MetadataSourceResponse;
import com.pulumi.azurenative.securityinsights.outputs.MetadataSupportResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Represents a Package in Azure Security Insights.
* Azure REST API version: 2023-06-01-preview.
*
* Other available API versions: 2023-07-01-preview, 2023-08-01-preview, 2023-09-01-preview, 2023-10-01-preview, 2023-11-01, 2023-12-01-preview, 2024-01-01-preview, 2024-03-01.
*
* ## Example Usage
* ### Install a package to the workspace.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.ContentPackage;
* import com.pulumi.azurenative.securityinsights.ContentPackageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var contentPackage = new ContentPackage("contentPackage", ContentPackageArgs.builder()
* .contentId("str.azure-sentinel-solution-str")
* .contentKind("Solution")
* .displayName("str")
* .packageId("str.azure-sentinel-solution-str")
* .resourceGroupName("myRg")
* .version("2.0.0")
* .workspaceName("myWorkspace")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:securityinsights:ContentPackage str.azure-sentinel-solution-str /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/providers/Microsoft.SecurityInsights/contentPackages/{packageId}
* ```
*
*/
@ResourceType(type="azure-native:securityinsights:ContentPackage")
public class ContentPackage extends com.pulumi.resources.CustomResource {
/**
* The author of the package
*
*/
@Export(name="author", refs={MetadataAuthorResponse.class}, tree="[0]")
private Output* @Nullable */ MetadataAuthorResponse> author;
/**
* @return The author of the package
*
*/
public Output> author() {
return Codegen.optional(this.author);
}
/**
* The categories of the package
*
*/
@Export(name="categories", refs={MetadataCategoriesResponse.class}, tree="[0]")
private Output* @Nullable */ MetadataCategoriesResponse> categories;
/**
* @return The categories of the package
*
*/
public Output> categories() {
return Codegen.optional(this.categories);
}
/**
* The package id
*
*/
@Export(name="contentId", refs={String.class}, tree="[0]")
private Output contentId;
/**
* @return The package id
*
*/
public Output contentId() {
return this.contentId;
}
/**
* The package kind
*
*/
@Export(name="contentKind", refs={String.class}, tree="[0]")
private Output contentKind;
/**
* @return The package kind
*
*/
public Output contentKind() {
return this.contentKind;
}
/**
* The version of the content schema.
*
*/
@Export(name="contentSchemaVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> contentSchemaVersion;
/**
* @return The version of the content schema.
*
*/
public Output> contentSchemaVersion() {
return Codegen.optional(this.contentSchemaVersion);
}
/**
* The support tier of the package
*
*/
@Export(name="dependencies", refs={MetadataDependenciesResponse.class}, tree="[0]")
private Output* @Nullable */ MetadataDependenciesResponse> dependencies;
/**
* @return The support tier of the package
*
*/
public Output> dependencies() {
return Codegen.optional(this.dependencies);
}
/**
* The description of the package
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description of the package
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The display name of the package
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output displayName;
/**
* @return The display name of the package
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Etag of the azure resource
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> etag;
/**
* @return Etag of the azure resource
*
*/
public Output> etag() {
return Codegen.optional(this.etag);
}
/**
* first publish date package item
*
*/
@Export(name="firstPublishDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> firstPublishDate;
/**
* @return first publish date package item
*
*/
public Output> firstPublishDate() {
return Codegen.optional(this.firstPublishDate);
}
/**
* the icon identifier. this id can later be fetched from the content metadata
*
*/
@Export(name="icon", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> icon;
/**
* @return the icon identifier. this id can later be fetched from the content metadata
*
*/
public Output> icon() {
return Codegen.optional(this.icon);
}
/**
* Flag indicates if this package is among the featured list.
*
*/
@Export(name="isFeatured", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> isFeatured;
/**
* @return Flag indicates if this package is among the featured list.
*
*/
public Output> isFeatured() {
return Codegen.optional(this.isFeatured);
}
/**
* Flag indicates if this is a newly published package.
*
*/
@Export(name="isNew", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> isNew;
/**
* @return Flag indicates if this is a newly published package.
*
*/
public Output> isNew() {
return Codegen.optional(this.isNew);
}
/**
* Flag indicates if this package is in preview.
*
*/
@Export(name="isPreview", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> isPreview;
/**
* @return Flag indicates if this package is in preview.
*
*/
public Output> isPreview() {
return Codegen.optional(this.isPreview);
}
/**
* last publish date for the package item
*
*/
@Export(name="lastPublishDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lastPublishDate;
/**
* @return last publish date for the package item
*
*/
public Output> lastPublishDate() {
return Codegen.optional(this.lastPublishDate);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Providers for the package item
*
*/
@Export(name="providers", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> providers;
/**
* @return Providers for the package item
*
*/
public Output>> providers() {
return Codegen.optional(this.providers);
}
/**
* The publisher display name of the package
*
*/
@Export(name="publisherDisplayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publisherDisplayName;
/**
* @return The publisher display name of the package
*
*/
public Output> publisherDisplayName() {
return Codegen.optional(this.publisherDisplayName);
}
/**
* The source of the package
*
*/
@Export(name="source", refs={MetadataSourceResponse.class}, tree="[0]")
private Output* @Nullable */ MetadataSourceResponse> source;
/**
* @return The source of the package
*
*/
public Output> source() {
return Codegen.optional(this.source);
}
/**
* The support tier of the package
*
*/
@Export(name="support", refs={MetadataSupportResponse.class}, tree="[0]")
private Output* @Nullable */ MetadataSupportResponse> support;
/**
* @return The support tier of the package
*
*/
public Output> support() {
return Codegen.optional(this.support);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* the tactics the resource covers
*
*/
@Export(name="threatAnalysisTactics", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> threatAnalysisTactics;
/**
* @return the tactics the resource covers
*
*/
public Output>> threatAnalysisTactics() {
return Codegen.optional(this.threatAnalysisTactics);
}
/**
* the techniques the resource covers, these have to be aligned with the tactics being used
*
*/
@Export(name="threatAnalysisTechniques", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> threatAnalysisTechniques;
/**
* @return the techniques the resource covers, these have to be aligned with the tactics being used
*
*/
public Output>> threatAnalysisTechniques() {
return Codegen.optional(this.threatAnalysisTechniques);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* the latest version number of the package
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return the latest version number of the package
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ContentPackage(java.lang.String name) {
this(name, ContentPackageArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ContentPackage(java.lang.String name, ContentPackageArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ContentPackage(java.lang.String name, ContentPackageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:securityinsights:ContentPackage", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ContentPackage(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:securityinsights:ContentPackage", name, null, makeResourceOptions(options, id), false);
}
private static ContentPackageArgs makeArgs(ContentPackageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ContentPackageArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:securityinsights/v20230401preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230501preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230601preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230701preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230801preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230901preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20231001preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20231101:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20231201preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20240101preview:ContentPackage").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20240301:ContentPackage").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ContentPackage get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ContentPackage(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy