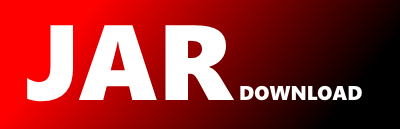
com.pulumi.azurenative.securityinsights.CustomizableConnectorDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.securityinsights.CustomizableConnectorDefinitionArgs;
import com.pulumi.azurenative.securityinsights.outputs.CustomizableConnectionsConfigResponse;
import com.pulumi.azurenative.securityinsights.outputs.CustomizableConnectorUiConfigResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Connector definition for kind 'Customizable'.
* Azure REST API version: 2023-07-01-preview.
*
* ## Example Usage
* ### Create data connector definition
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.securityinsights.CustomizableConnectorDefinition;
* import com.pulumi.azurenative.securityinsights.CustomizableConnectorDefinitionArgs;
* import com.pulumi.azurenative.securityinsights.inputs.CustomizableConnectorUiConfigArgs;
* import com.pulumi.azurenative.securityinsights.inputs.ConnectorDefinitionsAvailabilityArgs;
* import com.pulumi.azurenative.securityinsights.inputs.ConnectorDefinitionsPermissionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var customizableConnectorDefinition = new CustomizableConnectorDefinition("customizableConnectorDefinition", CustomizableConnectorDefinitionArgs.builder()
* .connectorUiConfig(CustomizableConnectorUiConfigArgs.builder()
* .availability(ConnectorDefinitionsAvailabilityArgs.builder()
* .isPreview(false)
* .status(1)
* .build())
* .connectivityCriteria(ConnectivityCriterionArgs.builder()
* .type("IsConnectedQuery")
* .value("""
* GitHubAuditLogPolling_CL
* | summarize LastLogReceived = max(TimeGenerated)
* | project IsConnected = LastLogReceived > ago(30d) """)
* .build())
* .dataTypes(ConnectorDataTypeArgs.builder()
* .lastDataReceivedQuery("""
* GitHubAuditLogPolling_CL
* | summarize Time = max(TimeGenerated)
* | where isnotempty(Time) """)
* .name("GitHubAuditLogPolling_CL")
* .build())
* .descriptionMarkdown("The GitHub audit log connector provides the capability to ingest GitHub logs into Azure Sentinel. By connecting GitHub audit logs into Azure Sentinel, you can view this data in workbooks, use it to create custom alerts, and improve your investigation process.")
* .graphQueries(GraphQueryArgs.builder()
* .baseQuery("GitHubAuditLogPolling_CL")
* .legend("GitHub audit log events")
* .metricName("Total events received")
* .build())
* .instructionSteps(InstructionStepArgs.builder()
* .description("""
* Enable GitHub audit Logs.
* Follow [this](https://docs.github.com/en/github/authenticating-to-github/keeping-your-account-and-data-secure/creating-a-personal-access-token) to create or find your personal key """)
* .instructions(InstructionStepDetailsArgs.builder()
* .parameters(Map.ofEntries(
* Map.entry("clientIdLabel", "Client ID"),
* Map.entry("clientSecretLabel", "Client Secret"),
* Map.entry("connectButtonLabel", "Connect"),
* Map.entry("disconnectButtonLabel", "Disconnect")
* ))
* .type("OAuthForm")
* .build())
* .title("Connect GitHub Enterprise Audit Log to Azure Sentinel")
* .build())
* .permissions(ConnectorDefinitionsPermissionsArgs.builder()
* .customs(CustomPermissionDetailsArgs.builder()
* .description("You need access to GitHub personal token, the key should have 'admin:org' scope")
* .name("GitHub API personal token Key")
* .build())
* .resourceProvider(ConnectorDefinitionsResourceProviderArgs.builder()
* .permissionsDisplayText("read and write permissions are required.")
* .provider("Microsoft.OperationalInsights/workspaces")
* .providerDisplayName("Workspace")
* .requiredPermissions(ResourceProviderRequiredPermissionsArgs.builder()
* .action(false)
* .delete(false)
* .read(false)
* .write(true)
* .build())
* .scope("Workspace")
* .build())
* .build())
* .publisher("GitHub")
* .sampleQueries(SampleQueryArgs.builder()
* .description("All logs")
* .query("""
* GitHubAuditLogPolling_CL
* | take 10 """)
* .build())
* .title("GitHub Enterprise Audit Log")
* .build())
* .dataConnectorDefinitionName("73e01a99-5cd7-4139-a149-9f2736ff2ab5")
* .kind("Customizable")
* .resourceGroupName("myRg")
* .workspaceName("myWorkspace")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:securityinsights:CustomizableConnectorDefinition 73e01a99-5cd7-4139-a149-9f2736ff2ab5 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/providers/Microsoft.SecurityInsights/dataConnectorDefinitions/{dataConnectorDefinitionName}
* ```
*
*/
@ResourceType(type="azure-native:securityinsights:CustomizableConnectorDefinition")
public class CustomizableConnectorDefinition extends com.pulumi.resources.CustomResource {
/**
* The UiConfig for 'Customizable' connector definition kind.
*
*/
@Export(name="connectionsConfig", refs={CustomizableConnectionsConfigResponse.class}, tree="[0]")
private Output* @Nullable */ CustomizableConnectionsConfigResponse> connectionsConfig;
/**
* @return The UiConfig for 'Customizable' connector definition kind.
*
*/
public Output> connectionsConfig() {
return Codegen.optional(this.connectionsConfig);
}
/**
* The UiConfig for 'Customizable' connector definition kind.
*
*/
@Export(name="connectorUiConfig", refs={CustomizableConnectorUiConfigResponse.class}, tree="[0]")
private Output connectorUiConfig;
/**
* @return The UiConfig for 'Customizable' connector definition kind.
*
*/
public Output connectorUiConfig() {
return this.connectorUiConfig;
}
/**
* Gets or sets the connector definition created date in UTC format.
*
*/
@Export(name="createdTimeUtc", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> createdTimeUtc;
/**
* @return Gets or sets the connector definition created date in UTC format.
*
*/
public Output> createdTimeUtc() {
return Codegen.optional(this.createdTimeUtc);
}
/**
* Etag of the azure resource
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> etag;
/**
* @return Etag of the azure resource
*
*/
public Output> etag() {
return Codegen.optional(this.etag);
}
/**
* The kind of the data connector definitions
* Expected value is 'Customizable'.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The kind of the data connector definitions
* Expected value is 'Customizable'.
*
*/
public Output kind() {
return this.kind;
}
/**
* Gets or sets the connector definition last modified date in UTC format.
*
*/
@Export(name="lastModifiedUtc", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lastModifiedUtc;
/**
* @return Gets or sets the connector definition last modified date in UTC format.
*
*/
public Output> lastModifiedUtc() {
return Codegen.optional(this.lastModifiedUtc);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public CustomizableConnectorDefinition(java.lang.String name) {
this(name, CustomizableConnectorDefinitionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public CustomizableConnectorDefinition(java.lang.String name, CustomizableConnectorDefinitionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public CustomizableConnectorDefinition(java.lang.String name, CustomizableConnectorDefinitionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:securityinsights:CustomizableConnectorDefinition", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private CustomizableConnectorDefinition(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:securityinsights:CustomizableConnectorDefinition", name, null, makeResourceOptions(options, id), false);
}
private static CustomizableConnectorDefinitionArgs makeArgs(CustomizableConnectorDefinitionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? CustomizableConnectorDefinitionArgs.builder() : CustomizableConnectorDefinitionArgs.builder(args);
return builder
.kind("Customizable")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:securityinsights/v20230701preview:CustomizableConnectorDefinition").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230801preview:CustomizableConnectorDefinition").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20230901preview:CustomizableConnectorDefinition").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20231001preview:CustomizableConnectorDefinition").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20231201preview:CustomizableConnectorDefinition").build()),
Output.of(Alias.builder().type("azure-native:securityinsights/v20240101preview:CustomizableConnectorDefinition").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static CustomizableConnectorDefinition get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new CustomizableConnectorDefinition(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy