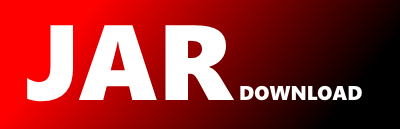
com.pulumi.azurenative.securityinsights.IncidentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.securityinsights.enums.IncidentClassification;
import com.pulumi.azurenative.securityinsights.enums.IncidentClassificationReason;
import com.pulumi.azurenative.securityinsights.enums.IncidentSeverity;
import com.pulumi.azurenative.securityinsights.enums.IncidentStatus;
import com.pulumi.azurenative.securityinsights.inputs.IncidentLabelArgs;
import com.pulumi.azurenative.securityinsights.inputs.IncidentOwnerInfoArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IncidentArgs extends com.pulumi.resources.ResourceArgs {
public static final IncidentArgs Empty = new IncidentArgs();
/**
* The reason the incident was closed
*
*/
@Import(name="classification")
private @Nullable Output> classification;
/**
* @return The reason the incident was closed
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy