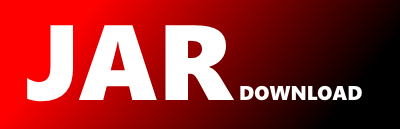
com.pulumi.azurenative.securityinsights.SourceControlArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights;
import com.pulumi.azurenative.securityinsights.enums.ContentType;
import com.pulumi.azurenative.securityinsights.enums.RepoType;
import com.pulumi.azurenative.securityinsights.enums.Version;
import com.pulumi.azurenative.securityinsights.inputs.DeploymentInfoArgs;
import com.pulumi.azurenative.securityinsights.inputs.RepositoryArgs;
import com.pulumi.azurenative.securityinsights.inputs.RepositoryResourceInfoArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SourceControlArgs extends com.pulumi.resources.ResourceArgs {
public static final SourceControlArgs Empty = new SourceControlArgs();
/**
* Array of source control content types.
*
*/
@Import(name="contentTypes", required=true)
private Output>> contentTypes;
/**
* @return Array of source control content types.
*
*/
public Output>> contentTypes() {
return this.contentTypes;
}
/**
* A description of the source control
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A description of the source control
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy