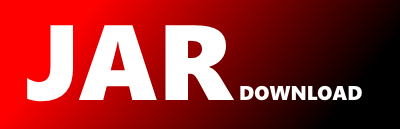
com.pulumi.azurenative.servicebus.RuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus;
import com.pulumi.azurenative.servicebus.enums.FilterType;
import com.pulumi.azurenative.servicebus.inputs.ActionArgs;
import com.pulumi.azurenative.servicebus.inputs.CorrelationFilterArgs;
import com.pulumi.azurenative.servicebus.inputs.SqlFilterArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RuleArgs extends com.pulumi.resources.ResourceArgs {
public static final RuleArgs Empty = new RuleArgs();
/**
* Represents the filter actions which are allowed for the transformation of a message that have been matched by a filter expression.
*
*/
@Import(name="action")
private @Nullable Output action;
/**
* @return Represents the filter actions which are allowed for the transformation of a message that have been matched by a filter expression.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy