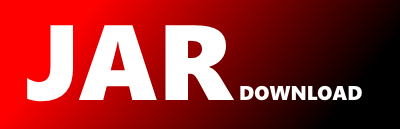
com.pulumi.azurenative.servicefabric.ManagedCluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabric;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.servicefabric.ManagedClusterArgs;
import com.pulumi.azurenative.servicefabric.outputs.ApplicationTypeVersionsCleanupPolicyResponse;
import com.pulumi.azurenative.servicefabric.outputs.AzureActiveDirectoryResponse;
import com.pulumi.azurenative.servicefabric.outputs.ClientCertificateResponse;
import com.pulumi.azurenative.servicefabric.outputs.IPTagResponse;
import com.pulumi.azurenative.servicefabric.outputs.LoadBalancingRuleResponse;
import com.pulumi.azurenative.servicefabric.outputs.NetworkSecurityRuleResponse;
import com.pulumi.azurenative.servicefabric.outputs.ServiceEndpointResponse;
import com.pulumi.azurenative.servicefabric.outputs.SettingsSectionDescriptionResponse;
import com.pulumi.azurenative.servicefabric.outputs.SkuResponse;
import com.pulumi.azurenative.servicefabric.outputs.SubnetResponse;
import com.pulumi.azurenative.servicefabric.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The managed cluster resource
*
* Azure REST API version: 2023-03-01-preview. Prior API version in Azure Native 1.x: 2020-01-01-preview.
*
* Other available API versions: 2020-01-01-preview, 2022-01-01, 2022-10-01-preview, 2023-07-01-preview, 2023-09-01-preview, 2023-11-01-preview, 2023-12-01-preview, 2024-02-01-preview, 2024-04-01, 2024-06-01-preview.
*
* ## Example Usage
* ### Put a cluster with maximum parameters
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicefabric.ManagedCluster;
* import com.pulumi.azurenative.servicefabric.ManagedClusterArgs;
* import com.pulumi.azurenative.servicefabric.inputs.ApplicationTypeVersionsCleanupPolicyArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SubnetArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SettingsSectionDescriptionArgs;
* import com.pulumi.azurenative.servicefabric.inputs.IPTagArgs;
* import com.pulumi.azurenative.servicefabric.inputs.LoadBalancingRuleArgs;
* import com.pulumi.azurenative.servicefabric.inputs.NetworkSecurityRuleArgs;
* import com.pulumi.azurenative.servicefabric.inputs.ServiceEndpointArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var managedCluster = new ManagedCluster("managedCluster", ManagedClusterArgs.builder()
* .addonFeatures(
* "DnsService",
* "BackupRestoreService",
* "ResourceMonitorService")
* .adminPassword("{vm-password}")
* .adminUserName("vmadmin")
* .allowRdpAccess(true)
* .applicationTypeVersionsCleanupPolicy(ApplicationTypeVersionsCleanupPolicyArgs.builder()
* .maxUnusedVersionsToKeep(3)
* .build())
* .auxiliarySubnets(SubnetArgs.builder()
* .enableIpv6(true)
* .name("testSubnet1")
* .networkSecurityGroupId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resRg/providers/Microsoft.Network/networkSecurityGroups/sn1")
* .privateEndpointNetworkPolicies("enabled")
* .privateLinkServiceNetworkPolicies("enabled")
* .build())
* .clientConnectionPort(19000)
* .clusterCodeVersion("7.1.168.9494")
* .clusterName("myCluster")
* .clusterUpgradeMode("Manual")
* .dnsName("myCluster")
* .enableAutoOSUpgrade(true)
* .enableIpv6(true)
* .fabricSettings(SettingsSectionDescriptionArgs.builder()
* .name("ManagedIdentityTokenService")
* .parameters(SettingsParameterDescriptionArgs.builder()
* .name("IsEnabled")
* .value("true")
* .build())
* .build())
* .httpGatewayConnectionPort(19080)
* .ipTags(IPTagArgs.builder()
* .ipTagType("FirstPartyUsage")
* .tag("SQL")
* .build())
* .loadBalancingRules(
* LoadBalancingRuleArgs.builder()
* .backendPort(80)
* .frontendPort(80)
* .probePort(80)
* .probeProtocol("http")
* .protocol("http")
* .build(),
* LoadBalancingRuleArgs.builder()
* .backendPort(443)
* .frontendPort(443)
* .probePort(443)
* .probeProtocol("http")
* .protocol("http")
* .build(),
* LoadBalancingRuleArgs.builder()
* .backendPort(10000)
* .frontendPort(10000)
* .loadDistribution("Default")
* .probePort(10000)
* .probeProtocol("http")
* .protocol("tcp")
* .build())
* .location("eastus")
* .networkSecurityRules(
* NetworkSecurityRuleArgs.builder()
* .access("allow")
* .description("Test description")
* .destinationAddressPrefixes("*")
* .destinationPortRanges("*")
* .direction("inbound")
* .name("TestName")
* .priority(1010)
* .protocol("tcp")
* .sourceAddressPrefixes("*")
* .sourcePortRanges("*")
* .build(),
* NetworkSecurityRuleArgs.builder()
* .access("allow")
* .destinationAddressPrefix("*")
* .destinationPortRange("33500-33699")
* .direction("inbound")
* .name("AllowARM")
* .priority(2002)
* .protocol("*")
* .sourceAddressPrefix("AzureResourceManager")
* .sourcePortRange("*")
* .build())
* .publicIPPrefixId("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/resRg/providers/Microsoft.Network/publicIPPrefixes/myPublicIPPrefix")
* .resourceGroupName("resRg")
* .serviceEndpoints(ServiceEndpointArgs.builder()
* .locations(
* "eastus2",
* "usnorth")
* .service("Microsoft.Storage")
* .build())
* .sku(SkuArgs.builder()
* .name("Basic")
* .build())
* .tags()
* .useCustomVnet(true)
* .zonalResiliency(true)
* .zonalUpdateMode("Fast")
* .build());
*
* }
* }
*
* }
*
* ### Put a cluster with minimum parameters
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicefabric.ManagedCluster;
* import com.pulumi.azurenative.servicefabric.ManagedClusterArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SettingsSectionDescriptionArgs;
* import com.pulumi.azurenative.servicefabric.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var managedCluster = new ManagedCluster("managedCluster", ManagedClusterArgs.builder()
* .adminPassword("{vm-password}")
* .adminUserName("vmadmin")
* .clusterName("myCluster")
* .clusterUpgradeCadence("Wave1")
* .clusterUpgradeMode("Automatic")
* .dnsName("myCluster")
* .fabricSettings(SettingsSectionDescriptionArgs.builder()
* .name("ManagedIdentityTokenService")
* .parameters(SettingsParameterDescriptionArgs.builder()
* .name("IsEnabled")
* .value("true")
* .build())
* .build())
* .location("eastus")
* .resourceGroupName("resRg")
* .sku(SkuArgs.builder()
* .name("Basic")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:servicefabric:ManagedCluster myCluster /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/managedClusters/{clusterName}
* ```
*
*/
@ResourceType(type="azure-native:servicefabric:ManagedCluster")
public class ManagedCluster extends com.pulumi.resources.CustomResource {
/**
* List of add-on features to enable on the cluster.
*
*/
@Export(name="addonFeatures", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> addonFeatures;
/**
* @return List of add-on features to enable on the cluster.
*
*/
public Output>> addonFeatures() {
return Codegen.optional(this.addonFeatures);
}
/**
* VM admin user password.
*
*/
@Export(name="adminPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> adminPassword;
/**
* @return VM admin user password.
*
*/
public Output> adminPassword() {
return Codegen.optional(this.adminPassword);
}
/**
* VM admin user name.
*
*/
@Export(name="adminUserName", refs={String.class}, tree="[0]")
private Output adminUserName;
/**
* @return VM admin user name.
*
*/
public Output adminUserName() {
return this.adminUserName;
}
/**
* Setting this to true enables RDP access to the VM. The default NSG rule opens RDP port to Internet which can be overridden with custom Network Security Rules. The default value for this setting is false.
*
*/
@Export(name="allowRdpAccess", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowRdpAccess;
/**
* @return Setting this to true enables RDP access to the VM. The default NSG rule opens RDP port to Internet which can be overridden with custom Network Security Rules. The default value for this setting is false.
*
*/
public Output> allowRdpAccess() {
return Codegen.optional(this.allowRdpAccess);
}
/**
* The policy used to clean up unused versions.
*
*/
@Export(name="applicationTypeVersionsCleanupPolicy", refs={ApplicationTypeVersionsCleanupPolicyResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationTypeVersionsCleanupPolicyResponse> applicationTypeVersionsCleanupPolicy;
/**
* @return The policy used to clean up unused versions.
*
*/
public Output> applicationTypeVersionsCleanupPolicy() {
return Codegen.optional(this.applicationTypeVersionsCleanupPolicy);
}
/**
* Auxiliary subnets for the cluster.
*
*/
@Export(name="auxiliarySubnets", refs={List.class,SubnetResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> auxiliarySubnets;
/**
* @return Auxiliary subnets for the cluster.
*
*/
public Output>> auxiliarySubnets() {
return Codegen.optional(this.auxiliarySubnets);
}
/**
* The AAD authentication settings of the cluster.
*
*/
@Export(name="azureActiveDirectory", refs={AzureActiveDirectoryResponse.class}, tree="[0]")
private Output* @Nullable */ AzureActiveDirectoryResponse> azureActiveDirectory;
/**
* @return The AAD authentication settings of the cluster.
*
*/
public Output> azureActiveDirectory() {
return Codegen.optional(this.azureActiveDirectory);
}
/**
* The port used for client connections to the cluster.
*
*/
@Export(name="clientConnectionPort", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> clientConnectionPort;
/**
* @return The port used for client connections to the cluster.
*
*/
public Output> clientConnectionPort() {
return Codegen.optional(this.clientConnectionPort);
}
/**
* Client certificates that are allowed to manage the cluster.
*
*/
@Export(name="clients", refs={List.class,ClientCertificateResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> clients;
/**
* @return Client certificates that are allowed to manage the cluster.
*
*/
public Output>> clients() {
return Codegen.optional(this.clients);
}
/**
* List of thumbprints of the cluster certificates.
*
*/
@Export(name="clusterCertificateThumbprints", refs={List.class,String.class}, tree="[0,1]")
private Output> clusterCertificateThumbprints;
/**
* @return List of thumbprints of the cluster certificates.
*
*/
public Output> clusterCertificateThumbprints() {
return this.clusterCertificateThumbprints;
}
/**
* The Service Fabric runtime version of the cluster. This property is required when **clusterUpgradeMode** is set to 'Manual'. To get list of available Service Fabric versions for new clusters use [ClusterVersion API](./ClusterVersion.md). To get the list of available version for existing clusters use **availableClusterVersions**.
*
*/
@Export(name="clusterCodeVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clusterCodeVersion;
/**
* @return The Service Fabric runtime version of the cluster. This property is required when **clusterUpgradeMode** is set to 'Manual'. To get list of available Service Fabric versions for new clusters use [ClusterVersion API](./ClusterVersion.md). To get the list of available version for existing clusters use **availableClusterVersions**.
*
*/
public Output> clusterCodeVersion() {
return Codegen.optional(this.clusterCodeVersion);
}
/**
* A service generated unique identifier for the cluster resource.
*
*/
@Export(name="clusterId", refs={String.class}, tree="[0]")
private Output clusterId;
/**
* @return A service generated unique identifier for the cluster resource.
*
*/
public Output clusterId() {
return this.clusterId;
}
/**
* The current state of the cluster.
*
*/
@Export(name="clusterState", refs={String.class}, tree="[0]")
private Output clusterState;
/**
* @return The current state of the cluster.
*
*/
public Output clusterState() {
return this.clusterState;
}
/**
* Indicates when new cluster runtime version upgrades will be applied after they are released. By default is Wave0. Only applies when **clusterUpgradeMode** is set to 'Automatic'.
*
*/
@Export(name="clusterUpgradeCadence", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clusterUpgradeCadence;
/**
* @return Indicates when new cluster runtime version upgrades will be applied after they are released. By default is Wave0. Only applies when **clusterUpgradeMode** is set to 'Automatic'.
*
*/
public Output> clusterUpgradeCadence() {
return Codegen.optional(this.clusterUpgradeCadence);
}
/**
* The upgrade mode of the cluster when new Service Fabric runtime version is available.
*
*/
@Export(name="clusterUpgradeMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clusterUpgradeMode;
/**
* @return The upgrade mode of the cluster when new Service Fabric runtime version is available.
*
*/
public Output> clusterUpgradeMode() {
return Codegen.optional(this.clusterUpgradeMode);
}
/**
* The cluster dns name.
*
*/
@Export(name="dnsName", refs={String.class}, tree="[0]")
private Output dnsName;
/**
* @return The cluster dns name.
*
*/
public Output dnsName() {
return this.dnsName;
}
/**
* Setting this to true enables automatic OS upgrade for the node types that are created using any platform OS image with version 'latest'. The default value for this setting is false.
*
*/
@Export(name="enableAutoOSUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableAutoOSUpgrade;
/**
* @return Setting this to true enables automatic OS upgrade for the node types that are created using any platform OS image with version 'latest'. The default value for this setting is false.
*
*/
public Output> enableAutoOSUpgrade() {
return Codegen.optional(this.enableAutoOSUpgrade);
}
/**
* Setting this to true creates IPv6 address space for the default VNet used by the cluster. This setting cannot be changed once the cluster is created. The default value for this setting is false.
*
*/
@Export(name="enableIpv6", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableIpv6;
/**
* @return Setting this to true creates IPv6 address space for the default VNet used by the cluster. This setting cannot be changed once the cluster is created. The default value for this setting is false.
*
*/
public Output> enableIpv6() {
return Codegen.optional(this.enableIpv6);
}
/**
* Setting this to true will link the IPv4 address as the ServicePublicIP of the IPv6 address. It can only be set to True if IPv6 is enabled on the cluster.
*
*/
@Export(name="enableServicePublicIP", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableServicePublicIP;
/**
* @return Setting this to true will link the IPv4 address as the ServicePublicIP of the IPv6 address. It can only be set to True if IPv6 is enabled on the cluster.
*
*/
public Output> enableServicePublicIP() {
return Codegen.optional(this.enableServicePublicIP);
}
/**
* Azure resource etag.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return Azure resource etag.
*
*/
public Output etag() {
return this.etag;
}
/**
* The list of custom fabric settings to configure the cluster.
*
*/
@Export(name="fabricSettings", refs={List.class,SettingsSectionDescriptionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> fabricSettings;
/**
* @return The list of custom fabric settings to configure the cluster.
*
*/
public Output>> fabricSettings() {
return Codegen.optional(this.fabricSettings);
}
/**
* The fully qualified domain name associated with the public load balancer of the cluster.
*
*/
@Export(name="fqdn", refs={String.class}, tree="[0]")
private Output fqdn;
/**
* @return The fully qualified domain name associated with the public load balancer of the cluster.
*
*/
public Output fqdn() {
return this.fqdn;
}
/**
* The port used for HTTP connections to the cluster.
*
*/
@Export(name="httpGatewayConnectionPort", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> httpGatewayConnectionPort;
/**
* @return The port used for HTTP connections to the cluster.
*
*/
public Output> httpGatewayConnectionPort() {
return Codegen.optional(this.httpGatewayConnectionPort);
}
/**
* The list of IP tags associated with the default public IP address of the cluster.
*
*/
@Export(name="ipTags", refs={List.class,IPTagResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> ipTags;
/**
* @return The list of IP tags associated with the default public IP address of the cluster.
*
*/
public Output>> ipTags() {
return Codegen.optional(this.ipTags);
}
/**
* The IPv4 address associated with the public load balancer of the cluster.
*
*/
@Export(name="ipv4Address", refs={String.class}, tree="[0]")
private Output ipv4Address;
/**
* @return The IPv4 address associated with the public load balancer of the cluster.
*
*/
public Output ipv4Address() {
return this.ipv4Address;
}
/**
* IPv6 address for the cluster if IPv6 is enabled.
*
*/
@Export(name="ipv6Address", refs={String.class}, tree="[0]")
private Output ipv6Address;
/**
* @return IPv6 address for the cluster if IPv6 is enabled.
*
*/
public Output ipv6Address() {
return this.ipv6Address;
}
/**
* Load balancing rules that are applied to the public load balancer of the cluster.
*
*/
@Export(name="loadBalancingRules", refs={List.class,LoadBalancingRuleResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> loadBalancingRules;
/**
* @return Load balancing rules that are applied to the public load balancer of the cluster.
*
*/
public Output>> loadBalancingRules() {
return Codegen.optional(this.loadBalancingRules);
}
/**
* Azure resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Azure resource location.
*
*/
public Output location() {
return this.location;
}
/**
* Azure resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Azure resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Custom Network Security Rules that are applied to the Virtual Network of the cluster.
*
*/
@Export(name="networkSecurityRules", refs={List.class,NetworkSecurityRuleResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> networkSecurityRules;
/**
* @return Custom Network Security Rules that are applied to the Virtual Network of the cluster.
*
*/
public Output>> networkSecurityRules() {
return Codegen.optional(this.networkSecurityRules);
}
/**
* The provisioning state of the managed cluster resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the managed cluster resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Specify the resource id of a public IP prefix that the load balancer will allocate a public IP address from. Only supports IPv4.
*
*/
@Export(name="publicIPPrefixId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicIPPrefixId;
/**
* @return Specify the resource id of a public IP prefix that the load balancer will allocate a public IP address from. Only supports IPv4.
*
*/
public Output> publicIPPrefixId() {
return Codegen.optional(this.publicIPPrefixId);
}
/**
* Service endpoints for subnets in the cluster.
*
*/
@Export(name="serviceEndpoints", refs={List.class,ServiceEndpointResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> serviceEndpoints;
/**
* @return Service endpoints for subnets in the cluster.
*
*/
public Output>> serviceEndpoints() {
return Codegen.optional(this.serviceEndpoints);
}
/**
* The sku of the managed cluster
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return The sku of the managed cluster
*
*/
public Output sku() {
return this.sku;
}
/**
* If specified, the node types for the cluster are created in this subnet instead of the default VNet. The **networkSecurityRules** specified for the cluster are also applied to this subnet. This setting cannot be changed once the cluster is created.
*
*/
@Export(name="subnetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> subnetId;
/**
* @return If specified, the node types for the cluster are created in this subnet instead of the default VNet. The **networkSecurityRules** specified for the cluster are also applied to this subnet. This setting cannot be changed once the cluster is created.
*
*/
public Output> subnetId() {
return Codegen.optional(this.subnetId);
}
/**
* Metadata pertaining to creation and last modification of the resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Azure resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Azure resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Azure resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Azure resource type.
*
*/
public Output type() {
return this.type;
}
/**
* For new clusters, this parameter indicates that it uses Bring your own VNet, but the subnet is specified at node type level; and for such clusters, the subnetId property is required for node types.
*
*/
@Export(name="useCustomVnet", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> useCustomVnet;
/**
* @return For new clusters, this parameter indicates that it uses Bring your own VNet, but the subnet is specified at node type level; and for such clusters, the subnetId property is required for node types.
*
*/
public Output> useCustomVnet() {
return Codegen.optional(this.useCustomVnet);
}
/**
* Indicates if the cluster has zone resiliency.
*
*/
@Export(name="zonalResiliency", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> zonalResiliency;
/**
* @return Indicates if the cluster has zone resiliency.
*
*/
public Output> zonalResiliency() {
return Codegen.optional(this.zonalResiliency);
}
/**
* Indicates the update mode for Cross Az clusters.
*
*/
@Export(name="zonalUpdateMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> zonalUpdateMode;
/**
* @return Indicates the update mode for Cross Az clusters.
*
*/
public Output> zonalUpdateMode() {
return Codegen.optional(this.zonalUpdateMode);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ManagedCluster(java.lang.String name) {
this(name, ManagedClusterArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ManagedCluster(java.lang.String name, ManagedClusterArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ManagedCluster(java.lang.String name, ManagedClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:servicefabric:ManagedCluster", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ManagedCluster(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:servicefabric:ManagedCluster", name, null, makeResourceOptions(options, id), false);
}
private static ManagedClusterArgs makeArgs(ManagedClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ManagedClusterArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:servicefabric/v20200101preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20210101preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20210501:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20210701preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20210901privatepreview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20211101preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20220101:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20220201preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20220601preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20220801preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20221001preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20230201preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20230301preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20230701preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20230901preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20231101preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20231201preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20240201preview:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20240401:ManagedCluster").build()),
Output.of(Alias.builder().type("azure-native:servicefabric/v20240601preview:ManagedCluster").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ManagedCluster get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ManagedCluster(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy