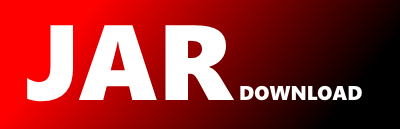
com.pulumi.azurenative.servicefabric.inputs.NetworkSecurityRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabric.inputs;
import com.pulumi.azurenative.servicefabric.enums.Access;
import com.pulumi.azurenative.servicefabric.enums.Direction;
import com.pulumi.azurenative.servicefabric.enums.NsgProtocol;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a network security rule.
*
*/
public final class NetworkSecurityRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkSecurityRuleArgs Empty = new NetworkSecurityRuleArgs();
/**
* The network traffic is allowed or denied.
*
*/
@Import(name="access", required=true)
private Output> access;
/**
* @return The network traffic is allowed or denied.
*
*/
public Output> access() {
return this.access;
}
/**
* Network security rule description.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return Network security rule description.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy