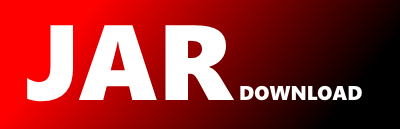
com.pulumi.azurenative.servicefabricmesh.inputs.ContainerCodePackagePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabricmesh.inputs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ApplicationScopedVolumeArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ContainerLabelArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.DiagnosticsRefArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.EndpointPropertiesArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.EnvironmentVariableArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ImageRegistryCredentialArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ReliableCollectionsRefArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ResourceRequirementsArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.SettingArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.VolumeReferenceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a container and its runtime properties.
*
*/
public final class ContainerCodePackagePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerCodePackagePropertiesArgs Empty = new ContainerCodePackagePropertiesArgs();
/**
* Command array to execute within the container in exec form.
*
*/
@Import(name="commands")
private @Nullable Output> commands;
/**
* @return Command array to execute within the container in exec form.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy