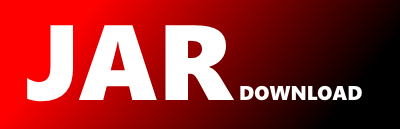
com.pulumi.azurenative.servicefabricmesh.outputs.GetApplicationResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabricmesh.outputs;
import com.pulumi.azurenative.servicefabricmesh.outputs.DiagnosticsDescriptionResponse;
import com.pulumi.azurenative.servicefabricmesh.outputs.ServiceResourceDescriptionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetApplicationResult {
/**
* @return Internal - used by Visual Studio to setup the debugging session on the local development environment.
*
*/
private @Nullable String debugParams;
/**
* @return User readable description of the application.
*
*/
private @Nullable String description;
/**
* @return Describes the diagnostics definition and usage for an application resource.
*
*/
private @Nullable DiagnosticsDescriptionResponse diagnostics;
/**
* @return Describes the health state of an application resource.
*
*/
private String healthState;
/**
* @return Fully qualified identifier for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return State of the resource.
*
*/
private String provisioningState;
/**
* @return Names of the services in the application.
*
*/
private List serviceNames;
/**
* @return Describes the services in the application. This property is used to create or modify services of the application. On get only the name of the service is returned. The service description can be obtained by querying for the service resource.
*
*/
private @Nullable List services;
/**
* @return Status of the application.
*
*/
private String status;
/**
* @return Gives additional information about the current status of the application.
*
*/
private String statusDetails;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
private String type;
/**
* @return When the application's health state is not 'Ok', this additional details from service fabric Health Manager for the user to know why the application is marked unhealthy.
*
*/
private String unhealthyEvaluation;
private GetApplicationResult() {}
/**
* @return Internal - used by Visual Studio to setup the debugging session on the local development environment.
*
*/
public Optional debugParams() {
return Optional.ofNullable(this.debugParams);
}
/**
* @return User readable description of the application.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Describes the diagnostics definition and usage for an application resource.
*
*/
public Optional diagnostics() {
return Optional.ofNullable(this.diagnostics);
}
/**
* @return Describes the health state of an application resource.
*
*/
public String healthState() {
return this.healthState;
}
/**
* @return Fully qualified identifier for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return State of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Names of the services in the application.
*
*/
public List serviceNames() {
return this.serviceNames;
}
/**
* @return Describes the services in the application. This property is used to create or modify services of the application. On get only the name of the service is returned. The service description can be obtained by querying for the service resource.
*
*/
public List services() {
return this.services == null ? List.of() : this.services;
}
/**
* @return Status of the application.
*
*/
public String status() {
return this.status;
}
/**
* @return Gives additional information about the current status of the application.
*
*/
public String statusDetails() {
return this.statusDetails;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public String type() {
return this.type;
}
/**
* @return When the application's health state is not 'Ok', this additional details from service fabric Health Manager for the user to know why the application is marked unhealthy.
*
*/
public String unhealthyEvaluation() {
return this.unhealthyEvaluation;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetApplicationResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String debugParams;
private @Nullable String description;
private @Nullable DiagnosticsDescriptionResponse diagnostics;
private String healthState;
private String id;
private String location;
private String name;
private String provisioningState;
private List serviceNames;
private @Nullable List services;
private String status;
private String statusDetails;
private @Nullable Map tags;
private String type;
private String unhealthyEvaluation;
public Builder() {}
public Builder(GetApplicationResult defaults) {
Objects.requireNonNull(defaults);
this.debugParams = defaults.debugParams;
this.description = defaults.description;
this.diagnostics = defaults.diagnostics;
this.healthState = defaults.healthState;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.serviceNames = defaults.serviceNames;
this.services = defaults.services;
this.status = defaults.status;
this.statusDetails = defaults.statusDetails;
this.tags = defaults.tags;
this.type = defaults.type;
this.unhealthyEvaluation = defaults.unhealthyEvaluation;
}
@CustomType.Setter
public Builder debugParams(@Nullable String debugParams) {
this.debugParams = debugParams;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder diagnostics(@Nullable DiagnosticsDescriptionResponse diagnostics) {
this.diagnostics = diagnostics;
return this;
}
@CustomType.Setter
public Builder healthState(String healthState) {
if (healthState == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "healthState");
}
this.healthState = healthState;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder serviceNames(List serviceNames) {
if (serviceNames == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "serviceNames");
}
this.serviceNames = serviceNames;
return this;
}
public Builder serviceNames(String... serviceNames) {
return serviceNames(List.of(serviceNames));
}
@CustomType.Setter
public Builder services(@Nullable List services) {
this.services = services;
return this;
}
public Builder services(ServiceResourceDescriptionResponse... services) {
return services(List.of(services));
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder statusDetails(String statusDetails) {
if (statusDetails == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "statusDetails");
}
this.statusDetails = statusDetails;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder unhealthyEvaluation(String unhealthyEvaluation) {
if (unhealthyEvaluation == null) {
throw new MissingRequiredPropertyException("GetApplicationResult", "unhealthyEvaluation");
}
this.unhealthyEvaluation = unhealthyEvaluation;
return this;
}
public GetApplicationResult build() {
final var _resultValue = new GetApplicationResult();
_resultValue.debugParams = debugParams;
_resultValue.description = description;
_resultValue.diagnostics = diagnostics;
_resultValue.healthState = healthState;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.serviceNames = serviceNames;
_resultValue.services = services;
_resultValue.status = status;
_resultValue.statusDetails = statusDetails;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.unhealthyEvaluation = unhealthyEvaluation;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy