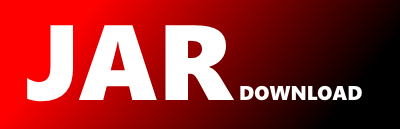
com.pulumi.azurenative.solutions.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.solutions;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.solutions.ApplicationArgs;
import com.pulumi.azurenative.solutions.outputs.ApplicationArtifactResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationAuthorizationResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationBillingDetailsDefinitionResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationClientDetailsResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationJitAccessPolicyResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationPackageContactResponse;
import com.pulumi.azurenative.solutions.outputs.ApplicationPackageSupportUrlsResponse;
import com.pulumi.azurenative.solutions.outputs.IdentityResponse;
import com.pulumi.azurenative.solutions.outputs.PlanResponse;
import com.pulumi.azurenative.solutions.outputs.SkuResponse;
import com.pulumi.azurenative.solutions.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Information about managed application.
* Azure REST API version: 2021-07-01. Prior API version in Azure Native 1.x: 2019-07-01.
*
* Other available API versions: 2017-12-01, 2018-06-01, 2023-12-01-preview.
*
* ## Example Usage
* ### Create or update managed application
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.solutions.Application;
* import com.pulumi.azurenative.solutions.ApplicationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var application = new Application("application", ApplicationArgs.builder()
* .applicationDefinitionId("/subscriptions/subid/resourceGroups/rg/providers/Microsoft.Solutions/applicationDefinitions/myAppDef")
* .applicationName("myManagedApplication")
* .kind("ServiceCatalog")
* .managedResourceGroupId("/subscriptions/subid/resourceGroups/myManagedRG")
* .resourceGroupName("rg")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:solutions:Application myManagedApplication /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Solutions/applications/{applicationName}
* ```
*
*/
@ResourceType(type="azure-native:solutions:Application")
public class Application extends com.pulumi.resources.CustomResource {
/**
* The fully qualified path of managed application definition Id.
*
*/
@Export(name="applicationDefinitionId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> applicationDefinitionId;
/**
* @return The fully qualified path of managed application definition Id.
*
*/
public Output> applicationDefinitionId() {
return Codegen.optional(this.applicationDefinitionId);
}
/**
* The collection of managed application artifacts.
*
*/
@Export(name="artifacts", refs={List.class,ApplicationArtifactResponse.class}, tree="[0,1]")
private Output> artifacts;
/**
* @return The collection of managed application artifacts.
*
*/
public Output> artifacts() {
return this.artifacts;
}
/**
* The read-only authorizations property that is retrieved from the application package.
*
*/
@Export(name="authorizations", refs={List.class,ApplicationAuthorizationResponse.class}, tree="[0,1]")
private Output> authorizations;
/**
* @return The read-only authorizations property that is retrieved from the application package.
*
*/
public Output> authorizations() {
return this.authorizations;
}
/**
* The managed application billing details.
*
*/
@Export(name="billingDetails", refs={ApplicationBillingDetailsDefinitionResponse.class}, tree="[0]")
private Output billingDetails;
/**
* @return The managed application billing details.
*
*/
public Output billingDetails() {
return this.billingDetails;
}
/**
* The client entity that created the JIT request.
*
*/
@Export(name="createdBy", refs={ApplicationClientDetailsResponse.class}, tree="[0]")
private Output createdBy;
/**
* @return The client entity that created the JIT request.
*
*/
public Output createdBy() {
return this.createdBy;
}
/**
* The read-only customer support property that is retrieved from the application package.
*
*/
@Export(name="customerSupport", refs={ApplicationPackageContactResponse.class}, tree="[0]")
private Output customerSupport;
/**
* @return The read-only customer support property that is retrieved from the application package.
*
*/
public Output customerSupport() {
return this.customerSupport;
}
/**
* The identity of the resource.
*
*/
@Export(name="identity", refs={IdentityResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityResponse> identity;
/**
* @return The identity of the resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The managed application Jit access policy.
*
*/
@Export(name="jitAccessPolicy", refs={ApplicationJitAccessPolicyResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationJitAccessPolicyResponse> jitAccessPolicy;
/**
* @return The managed application Jit access policy.
*
*/
public Output> jitAccessPolicy() {
return Codegen.optional(this.jitAccessPolicy);
}
/**
* The kind of the managed application. Allowed values are MarketPlace and ServiceCatalog.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The kind of the managed application. Allowed values are MarketPlace and ServiceCatalog.
*
*/
public Output kind() {
return this.kind;
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return Resource location
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* ID of the resource that manages this resource.
*
*/
@Export(name="managedBy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> managedBy;
/**
* @return ID of the resource that manages this resource.
*
*/
public Output> managedBy() {
return Codegen.optional(this.managedBy);
}
/**
* The managed resource group Id.
*
*/
@Export(name="managedResourceGroupId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> managedResourceGroupId;
/**
* @return The managed resource group Id.
*
*/
public Output> managedResourceGroupId() {
return Codegen.optional(this.managedResourceGroupId);
}
/**
* The managed application management mode.
*
*/
@Export(name="managementMode", refs={String.class}, tree="[0]")
private Output managementMode;
/**
* @return The managed application management mode.
*
*/
public Output managementMode() {
return this.managementMode;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Name and value pairs that define the managed application outputs.
*
*/
@Export(name="outputs", refs={Object.class}, tree="[0]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy