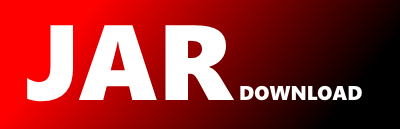
com.pulumi.azurenative.solutions.outputs.ManagedIdentityTokenResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.solutions.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ManagedIdentityTokenResponse {
/**
* @return The requested access token.
*
*/
private @Nullable String accessToken;
/**
* @return The aud (audience) the access token was request for. This is the same as what was provided in the listTokens request.
*
*/
private @Nullable String authorizationAudience;
/**
* @return The number of seconds the access token will be valid.
*
*/
private @Nullable String expiresIn;
/**
* @return The timespan when the access token expires. This is represented as the number of seconds from epoch.
*
*/
private @Nullable String expiresOn;
/**
* @return The timespan when the access token takes effect. This is represented as the number of seconds from epoch.
*
*/
private @Nullable String notBefore;
/**
* @return The Azure resource ID for the issued token. This is either the managed application ID or the user-assigned identity ID.
*
*/
private @Nullable String resourceId;
/**
* @return The type of the token.
*
*/
private @Nullable String tokenType;
private ManagedIdentityTokenResponse() {}
/**
* @return The requested access token.
*
*/
public Optional accessToken() {
return Optional.ofNullable(this.accessToken);
}
/**
* @return The aud (audience) the access token was request for. This is the same as what was provided in the listTokens request.
*
*/
public Optional authorizationAudience() {
return Optional.ofNullable(this.authorizationAudience);
}
/**
* @return The number of seconds the access token will be valid.
*
*/
public Optional expiresIn() {
return Optional.ofNullable(this.expiresIn);
}
/**
* @return The timespan when the access token expires. This is represented as the number of seconds from epoch.
*
*/
public Optional expiresOn() {
return Optional.ofNullable(this.expiresOn);
}
/**
* @return The timespan when the access token takes effect. This is represented as the number of seconds from epoch.
*
*/
public Optional notBefore() {
return Optional.ofNullable(this.notBefore);
}
/**
* @return The Azure resource ID for the issued token. This is either the managed application ID or the user-assigned identity ID.
*
*/
public Optional resourceId() {
return Optional.ofNullable(this.resourceId);
}
/**
* @return The type of the token.
*
*/
public Optional tokenType() {
return Optional.ofNullable(this.tokenType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ManagedIdentityTokenResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String accessToken;
private @Nullable String authorizationAudience;
private @Nullable String expiresIn;
private @Nullable String expiresOn;
private @Nullable String notBefore;
private @Nullable String resourceId;
private @Nullable String tokenType;
public Builder() {}
public Builder(ManagedIdentityTokenResponse defaults) {
Objects.requireNonNull(defaults);
this.accessToken = defaults.accessToken;
this.authorizationAudience = defaults.authorizationAudience;
this.expiresIn = defaults.expiresIn;
this.expiresOn = defaults.expiresOn;
this.notBefore = defaults.notBefore;
this.resourceId = defaults.resourceId;
this.tokenType = defaults.tokenType;
}
@CustomType.Setter
public Builder accessToken(@Nullable String accessToken) {
this.accessToken = accessToken;
return this;
}
@CustomType.Setter
public Builder authorizationAudience(@Nullable String authorizationAudience) {
this.authorizationAudience = authorizationAudience;
return this;
}
@CustomType.Setter
public Builder expiresIn(@Nullable String expiresIn) {
this.expiresIn = expiresIn;
return this;
}
@CustomType.Setter
public Builder expiresOn(@Nullable String expiresOn) {
this.expiresOn = expiresOn;
return this;
}
@CustomType.Setter
public Builder notBefore(@Nullable String notBefore) {
this.notBefore = notBefore;
return this;
}
@CustomType.Setter
public Builder resourceId(@Nullable String resourceId) {
this.resourceId = resourceId;
return this;
}
@CustomType.Setter
public Builder tokenType(@Nullable String tokenType) {
this.tokenType = tokenType;
return this;
}
public ManagedIdentityTokenResponse build() {
final var _resultValue = new ManagedIdentityTokenResponse();
_resultValue.accessToken = accessToken;
_resultValue.authorizationAudience = authorizationAudience;
_resultValue.expiresIn = expiresIn;
_resultValue.expiresOn = expiresOn;
_resultValue.notBefore = notBefore;
_resultValue.resourceId = resourceId;
_resultValue.tokenType = tokenType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy