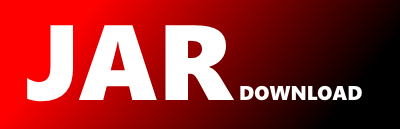
com.pulumi.azurenative.sql.BackupShortTermRetentionPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BackupShortTermRetentionPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final BackupShortTermRetentionPolicyArgs Empty = new BackupShortTermRetentionPolicyArgs();
/**
* The name of the database.
*
*/
@Import(name="databaseName", required=true)
private Output databaseName;
/**
* @return The name of the database.
*
*/
public Output databaseName() {
return this.databaseName;
}
/**
* The differential backup interval in hours. This is how many interval hours between each differential backup will be supported. This is only applicable to live databases but not dropped databases.
*
*/
@Import(name="diffBackupIntervalInHours")
private @Nullable Output diffBackupIntervalInHours;
/**
* @return The differential backup interval in hours. This is how many interval hours between each differential backup will be supported. This is only applicable to live databases but not dropped databases.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy