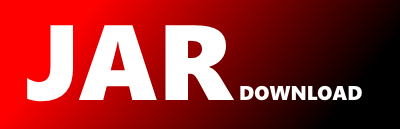
com.pulumi.azurenative.storage.BlobArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.asset.AssetOrArchive;
import com.pulumi.azurenative.storage.enums.BlobAccessTier;
import com.pulumi.azurenative.storage.enums.BlobType;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BlobArgs extends com.pulumi.resources.ResourceArgs {
public static final BlobArgs Empty = new BlobArgs();
/**
* The access tier of the storage blob. Only supported for standard storage accounts, not premium.
*
*/
@Import(name="accessTier")
private @Nullable Output accessTier;
/**
* @return The access tier of the storage blob. Only supported for standard storage accounts, not premium.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy