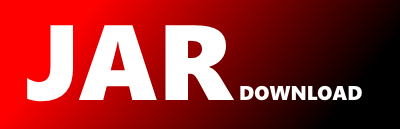
com.pulumi.azurenative.storage.BlobContainerImmutabilityPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BlobContainerImmutabilityPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final BlobContainerImmutabilityPolicyArgs Empty = new BlobContainerImmutabilityPolicyArgs();
/**
* The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to an append blob while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API.
*
*/
@Import(name="allowProtectedAppendWrites")
private @Nullable Output allowProtectedAppendWrites;
/**
* @return This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to an append blob while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy