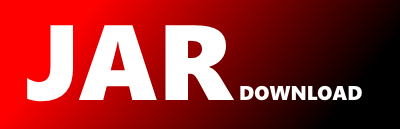
com.pulumi.azurenative.storage.outputs.GetFileShareResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.outputs;
import com.pulumi.azurenative.storage.outputs.SignedIdentifierResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetFileShareResult {
/**
* @return Access tier for specific share. GpV2 account can choose between TransactionOptimized (default), Hot, and Cool. FileStorage account can choose Premium.
*
*/
private @Nullable String accessTier;
/**
* @return Indicates the last modification time for share access tier.
*
*/
private String accessTierChangeTime;
/**
* @return Indicates if there is a pending transition for access tier.
*
*/
private String accessTierStatus;
/**
* @return Indicates whether the share was deleted.
*
*/
private Boolean deleted;
/**
* @return The deleted time if the share was deleted.
*
*/
private String deletedTime;
/**
* @return The authentication protocol that is used for the file share. Can only be specified when creating a share.
*
*/
private @Nullable String enabledProtocols;
/**
* @return Resource Etag.
*
*/
private String etag;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Returns the date and time the share was last modified.
*
*/
private String lastModifiedTime;
/**
* @return Specifies whether the lease on a share is of infinite or fixed duration, only when the share is leased.
*
*/
private String leaseDuration;
/**
* @return Lease state of the share.
*
*/
private String leaseState;
/**
* @return The lease status of the share.
*
*/
private String leaseStatus;
/**
* @return A name-value pair to associate with the share as metadata.
*
*/
private @Nullable Map metadata;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Remaining retention days for share that was soft deleted.
*
*/
private Integer remainingRetentionDays;
/**
* @return The property is for NFS share only. The default is NoRootSquash.
*
*/
private @Nullable String rootSquash;
/**
* @return The maximum size of the share, in gigabytes. Must be greater than 0, and less than or equal to 5TB (5120). For Large File Shares, the maximum size is 102400.
*
*/
private @Nullable Integer shareQuota;
/**
* @return The approximate size of the data stored on the share. Note that this value may not include all recently created or recently resized files.
*
*/
private Double shareUsageBytes;
/**
* @return List of stored access policies specified on the share.
*
*/
private @Nullable List signedIdentifiers;
/**
* @return Creation time of share snapshot returned in the response of list shares with expand param "snapshots".
*
*/
private String snapshotTime;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The version of the share.
*
*/
private String version;
private GetFileShareResult() {}
/**
* @return Access tier for specific share. GpV2 account can choose between TransactionOptimized (default), Hot, and Cool. FileStorage account can choose Premium.
*
*/
public Optional accessTier() {
return Optional.ofNullable(this.accessTier);
}
/**
* @return Indicates the last modification time for share access tier.
*
*/
public String accessTierChangeTime() {
return this.accessTierChangeTime;
}
/**
* @return Indicates if there is a pending transition for access tier.
*
*/
public String accessTierStatus() {
return this.accessTierStatus;
}
/**
* @return Indicates whether the share was deleted.
*
*/
public Boolean deleted() {
return this.deleted;
}
/**
* @return The deleted time if the share was deleted.
*
*/
public String deletedTime() {
return this.deletedTime;
}
/**
* @return The authentication protocol that is used for the file share. Can only be specified when creating a share.
*
*/
public Optional enabledProtocols() {
return Optional.ofNullable(this.enabledProtocols);
}
/**
* @return Resource Etag.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Returns the date and time the share was last modified.
*
*/
public String lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* @return Specifies whether the lease on a share is of infinite or fixed duration, only when the share is leased.
*
*/
public String leaseDuration() {
return this.leaseDuration;
}
/**
* @return Lease state of the share.
*
*/
public String leaseState() {
return this.leaseState;
}
/**
* @return The lease status of the share.
*
*/
public String leaseStatus() {
return this.leaseStatus;
}
/**
* @return A name-value pair to associate with the share as metadata.
*
*/
public Map metadata() {
return this.metadata == null ? Map.of() : this.metadata;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Remaining retention days for share that was soft deleted.
*
*/
public Integer remainingRetentionDays() {
return this.remainingRetentionDays;
}
/**
* @return The property is for NFS share only. The default is NoRootSquash.
*
*/
public Optional rootSquash() {
return Optional.ofNullable(this.rootSquash);
}
/**
* @return The maximum size of the share, in gigabytes. Must be greater than 0, and less than or equal to 5TB (5120). For Large File Shares, the maximum size is 102400.
*
*/
public Optional shareQuota() {
return Optional.ofNullable(this.shareQuota);
}
/**
* @return The approximate size of the data stored on the share. Note that this value may not include all recently created or recently resized files.
*
*/
public Double shareUsageBytes() {
return this.shareUsageBytes;
}
/**
* @return List of stored access policies specified on the share.
*
*/
public List signedIdentifiers() {
return this.signedIdentifiers == null ? List.of() : this.signedIdentifiers;
}
/**
* @return Creation time of share snapshot returned in the response of list shares with expand param "snapshots".
*
*/
public String snapshotTime() {
return this.snapshotTime;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The version of the share.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFileShareResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String accessTier;
private String accessTierChangeTime;
private String accessTierStatus;
private Boolean deleted;
private String deletedTime;
private @Nullable String enabledProtocols;
private String etag;
private String id;
private String lastModifiedTime;
private String leaseDuration;
private String leaseState;
private String leaseStatus;
private @Nullable Map metadata;
private String name;
private Integer remainingRetentionDays;
private @Nullable String rootSquash;
private @Nullable Integer shareQuota;
private Double shareUsageBytes;
private @Nullable List signedIdentifiers;
private String snapshotTime;
private String type;
private String version;
public Builder() {}
public Builder(GetFileShareResult defaults) {
Objects.requireNonNull(defaults);
this.accessTier = defaults.accessTier;
this.accessTierChangeTime = defaults.accessTierChangeTime;
this.accessTierStatus = defaults.accessTierStatus;
this.deleted = defaults.deleted;
this.deletedTime = defaults.deletedTime;
this.enabledProtocols = defaults.enabledProtocols;
this.etag = defaults.etag;
this.id = defaults.id;
this.lastModifiedTime = defaults.lastModifiedTime;
this.leaseDuration = defaults.leaseDuration;
this.leaseState = defaults.leaseState;
this.leaseStatus = defaults.leaseStatus;
this.metadata = defaults.metadata;
this.name = defaults.name;
this.remainingRetentionDays = defaults.remainingRetentionDays;
this.rootSquash = defaults.rootSquash;
this.shareQuota = defaults.shareQuota;
this.shareUsageBytes = defaults.shareUsageBytes;
this.signedIdentifiers = defaults.signedIdentifiers;
this.snapshotTime = defaults.snapshotTime;
this.type = defaults.type;
this.version = defaults.version;
}
@CustomType.Setter
public Builder accessTier(@Nullable String accessTier) {
this.accessTier = accessTier;
return this;
}
@CustomType.Setter
public Builder accessTierChangeTime(String accessTierChangeTime) {
if (accessTierChangeTime == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "accessTierChangeTime");
}
this.accessTierChangeTime = accessTierChangeTime;
return this;
}
@CustomType.Setter
public Builder accessTierStatus(String accessTierStatus) {
if (accessTierStatus == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "accessTierStatus");
}
this.accessTierStatus = accessTierStatus;
return this;
}
@CustomType.Setter
public Builder deleted(Boolean deleted) {
if (deleted == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "deleted");
}
this.deleted = deleted;
return this;
}
@CustomType.Setter
public Builder deletedTime(String deletedTime) {
if (deletedTime == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "deletedTime");
}
this.deletedTime = deletedTime;
return this;
}
@CustomType.Setter
public Builder enabledProtocols(@Nullable String enabledProtocols) {
this.enabledProtocols = enabledProtocols;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(String lastModifiedTime) {
if (lastModifiedTime == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "lastModifiedTime");
}
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder leaseDuration(String leaseDuration) {
if (leaseDuration == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "leaseDuration");
}
this.leaseDuration = leaseDuration;
return this;
}
@CustomType.Setter
public Builder leaseState(String leaseState) {
if (leaseState == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "leaseState");
}
this.leaseState = leaseState;
return this;
}
@CustomType.Setter
public Builder leaseStatus(String leaseStatus) {
if (leaseStatus == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "leaseStatus");
}
this.leaseStatus = leaseStatus;
return this;
}
@CustomType.Setter
public Builder metadata(@Nullable Map metadata) {
this.metadata = metadata;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder remainingRetentionDays(Integer remainingRetentionDays) {
if (remainingRetentionDays == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "remainingRetentionDays");
}
this.remainingRetentionDays = remainingRetentionDays;
return this;
}
@CustomType.Setter
public Builder rootSquash(@Nullable String rootSquash) {
this.rootSquash = rootSquash;
return this;
}
@CustomType.Setter
public Builder shareQuota(@Nullable Integer shareQuota) {
this.shareQuota = shareQuota;
return this;
}
@CustomType.Setter
public Builder shareUsageBytes(Double shareUsageBytes) {
if (shareUsageBytes == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "shareUsageBytes");
}
this.shareUsageBytes = shareUsageBytes;
return this;
}
@CustomType.Setter
public Builder signedIdentifiers(@Nullable List signedIdentifiers) {
this.signedIdentifiers = signedIdentifiers;
return this;
}
public Builder signedIdentifiers(SignedIdentifierResponse... signedIdentifiers) {
return signedIdentifiers(List.of(signedIdentifiers));
}
@CustomType.Setter
public Builder snapshotTime(String snapshotTime) {
if (snapshotTime == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "snapshotTime");
}
this.snapshotTime = snapshotTime;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("GetFileShareResult", "version");
}
this.version = version;
return this;
}
public GetFileShareResult build() {
final var _resultValue = new GetFileShareResult();
_resultValue.accessTier = accessTier;
_resultValue.accessTierChangeTime = accessTierChangeTime;
_resultValue.accessTierStatus = accessTierStatus;
_resultValue.deleted = deleted;
_resultValue.deletedTime = deletedTime;
_resultValue.enabledProtocols = enabledProtocols;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.leaseDuration = leaseDuration;
_resultValue.leaseState = leaseState;
_resultValue.leaseStatus = leaseStatus;
_resultValue.metadata = metadata;
_resultValue.name = name;
_resultValue.remainingRetentionDays = remainingRetentionDays;
_resultValue.rootSquash = rootSquash;
_resultValue.shareQuota = shareQuota;
_resultValue.shareUsageBytes = shareUsageBytes;
_resultValue.signedIdentifiers = signedIdentifiers;
_resultValue.snapshotTime = snapshotTime;
_resultValue.type = type;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy