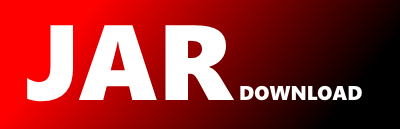
com.pulumi.azurenative.storage.outputs.GetStorageAccountResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.outputs;
import com.pulumi.azurenative.storage.outputs.AzureFilesIdentityBasedAuthenticationResponse;
import com.pulumi.azurenative.storage.outputs.BlobRestoreStatusResponse;
import com.pulumi.azurenative.storage.outputs.CustomDomainResponse;
import com.pulumi.azurenative.storage.outputs.EncryptionResponse;
import com.pulumi.azurenative.storage.outputs.EndpointsResponse;
import com.pulumi.azurenative.storage.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.storage.outputs.GeoReplicationStatsResponse;
import com.pulumi.azurenative.storage.outputs.IdentityResponse;
import com.pulumi.azurenative.storage.outputs.ImmutableStorageAccountResponse;
import com.pulumi.azurenative.storage.outputs.KeyCreationTimeResponse;
import com.pulumi.azurenative.storage.outputs.KeyPolicyResponse;
import com.pulumi.azurenative.storage.outputs.NetworkRuleSetResponse;
import com.pulumi.azurenative.storage.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.storage.outputs.RoutingPreferenceResponse;
import com.pulumi.azurenative.storage.outputs.SasPolicyResponse;
import com.pulumi.azurenative.storage.outputs.SkuResponse;
import com.pulumi.azurenative.storage.outputs.StorageAccountSkuConversionStatusResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetStorageAccountResult {
/**
* @return Required for storage accounts where kind = BlobStorage. The access tier is used for billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it cannot be changed for the premium block blobs storage account type.
*
*/
private String accessTier;
/**
* @return Allow or disallow public access to all blobs or containers in the storage account. The default interpretation is true for this property.
*
*/
private @Nullable Boolean allowBlobPublicAccess;
/**
* @return Allow or disallow cross AAD tenant object replication. The default interpretation is true for this property.
*
*/
private @Nullable Boolean allowCrossTenantReplication;
/**
* @return Indicates whether the storage account permits requests to be authorized with the account access key via Shared Key. If false, then all requests, including shared access signatures, must be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
*/
private @Nullable Boolean allowSharedKeyAccess;
/**
* @return Restrict copy to and from Storage Accounts within an AAD tenant or with Private Links to the same VNet.
*
*/
private @Nullable String allowedCopyScope;
/**
* @return Provides the identity based authentication settings for Azure Files.
*
*/
private @Nullable AzureFilesIdentityBasedAuthenticationResponse azureFilesIdentityBasedAuthentication;
/**
* @return Blob restore status
*
*/
private BlobRestoreStatusResponse blobRestoreStatus;
/**
* @return Gets the creation date and time of the storage account in UTC.
*
*/
private String creationTime;
/**
* @return Gets the custom domain the user assigned to this storage account.
*
*/
private CustomDomainResponse customDomain;
/**
* @return A boolean flag which indicates whether the default authentication is OAuth or not. The default interpretation is false for this property.
*
*/
private @Nullable Boolean defaultToOAuthAuthentication;
/**
* @return Allows you to specify the type of endpoint. Set this to AzureDNSZone to create a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint URL will have an alphanumeric DNS Zone identifier.
*
*/
private @Nullable String dnsEndpointType;
/**
* @return Allows https traffic only to storage service if sets to true.
*
*/
private @Nullable Boolean enableHttpsTrafficOnly;
/**
* @return NFS 3.0 protocol support enabled if set to true.
*
*/
private @Nullable Boolean enableNfsV3;
/**
* @return Encryption settings to be used for server-side encryption for the storage account.
*
*/
private EncryptionResponse encryption;
/**
* @return The extendedLocation of the resource.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return If the failover is in progress, the value will be true, otherwise, it will be null.
*
*/
private Boolean failoverInProgress;
/**
* @return Geo Replication Stats
*
*/
private GeoReplicationStatsResponse geoReplicationStats;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The identity of the resource.
*
*/
private @Nullable IdentityResponse identity;
/**
* @return The property is immutable and can only be set to true at the account creation time. When set to true, it enables object level immutability for all the containers in the account by default.
*
*/
private @Nullable ImmutableStorageAccountResponse immutableStorageWithVersioning;
/**
* @return Account HierarchicalNamespace enabled if sets to true.
*
*/
private @Nullable Boolean isHnsEnabled;
/**
* @return Enables local users feature, if set to true
*
*/
private @Nullable Boolean isLocalUserEnabled;
/**
* @return Enables Secure File Transfer Protocol, if set to true
*
*/
private @Nullable Boolean isSftpEnabled;
/**
* @return Storage account keys creation time.
*
*/
private KeyCreationTimeResponse keyCreationTime;
/**
* @return KeyPolicy assigned to the storage account.
*
*/
private KeyPolicyResponse keyPolicy;
/**
* @return Gets the Kind.
*
*/
private String kind;
/**
* @return Allow large file shares if sets to Enabled. It cannot be disabled once it is enabled.
*
*/
private @Nullable String largeFileSharesState;
/**
* @return Gets the timestamp of the most recent instance of a failover to the secondary location. Only the most recent timestamp is retained. This element is not returned if there has never been a failover instance. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
private String lastGeoFailoverTime;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Set the minimum TLS version to be permitted on requests to storage. The default interpretation is TLS 1.0 for this property.
*
*/
private @Nullable String minimumTlsVersion;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Network rule set
*
*/
private NetworkRuleSetResponse networkRuleSet;
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object. Note that Standard_ZRS and Premium_LRS accounts only return the blob endpoint.
*
*/
private EndpointsResponse primaryEndpoints;
/**
* @return Gets the location of the primary data center for the storage account.
*
*/
private String primaryLocation;
/**
* @return List of private endpoint connection associated with the specified storage account
*
*/
private List privateEndpointConnections;
/**
* @return Gets the status of the storage account at the time the operation was called.
*
*/
private String provisioningState;
/**
* @return Allow or disallow public network access to Storage Account. Value is optional but if passed in, must be 'Enabled' or 'Disabled'.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Maintains information about the network routing choice opted by the user for data transfer
*
*/
private @Nullable RoutingPreferenceResponse routingPreference;
/**
* @return SasPolicy assigned to the storage account.
*
*/
private SasPolicyResponse sasPolicy;
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object from the secondary location of the storage account. Only available if the SKU name is Standard_RAGRS.
*
*/
private EndpointsResponse secondaryEndpoints;
/**
* @return Gets the location of the geo-replicated secondary for the storage account. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
private String secondaryLocation;
/**
* @return Gets the SKU.
*
*/
private SkuResponse sku;
/**
* @return Gets the status indicating whether the primary location of the storage account is available or unavailable.
*
*/
private String statusOfPrimary;
/**
* @return Gets the status indicating whether the secondary location of the storage account is available or unavailable. Only available if the SKU name is Standard_GRS or Standard_RAGRS.
*
*/
private String statusOfSecondary;
/**
* @return This property is readOnly and is set by server during asynchronous storage account sku conversion operations.
*
*/
private @Nullable StorageAccountSkuConversionStatusResponse storageAccountSkuConversionStatus;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetStorageAccountResult() {}
/**
* @return Required for storage accounts where kind = BlobStorage. The access tier is used for billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it cannot be changed for the premium block blobs storage account type.
*
*/
public String accessTier() {
return this.accessTier;
}
/**
* @return Allow or disallow public access to all blobs or containers in the storage account. The default interpretation is true for this property.
*
*/
public Optional allowBlobPublicAccess() {
return Optional.ofNullable(this.allowBlobPublicAccess);
}
/**
* @return Allow or disallow cross AAD tenant object replication. The default interpretation is true for this property.
*
*/
public Optional allowCrossTenantReplication() {
return Optional.ofNullable(this.allowCrossTenantReplication);
}
/**
* @return Indicates whether the storage account permits requests to be authorized with the account access key via Shared Key. If false, then all requests, including shared access signatures, must be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
*/
public Optional allowSharedKeyAccess() {
return Optional.ofNullable(this.allowSharedKeyAccess);
}
/**
* @return Restrict copy to and from Storage Accounts within an AAD tenant or with Private Links to the same VNet.
*
*/
public Optional allowedCopyScope() {
return Optional.ofNullable(this.allowedCopyScope);
}
/**
* @return Provides the identity based authentication settings for Azure Files.
*
*/
public Optional azureFilesIdentityBasedAuthentication() {
return Optional.ofNullable(this.azureFilesIdentityBasedAuthentication);
}
/**
* @return Blob restore status
*
*/
public BlobRestoreStatusResponse blobRestoreStatus() {
return this.blobRestoreStatus;
}
/**
* @return Gets the creation date and time of the storage account in UTC.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return Gets the custom domain the user assigned to this storage account.
*
*/
public CustomDomainResponse customDomain() {
return this.customDomain;
}
/**
* @return A boolean flag which indicates whether the default authentication is OAuth or not. The default interpretation is false for this property.
*
*/
public Optional defaultToOAuthAuthentication() {
return Optional.ofNullable(this.defaultToOAuthAuthentication);
}
/**
* @return Allows you to specify the type of endpoint. Set this to AzureDNSZone to create a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint URL will have an alphanumeric DNS Zone identifier.
*
*/
public Optional dnsEndpointType() {
return Optional.ofNullable(this.dnsEndpointType);
}
/**
* @return Allows https traffic only to storage service if sets to true.
*
*/
public Optional enableHttpsTrafficOnly() {
return Optional.ofNullable(this.enableHttpsTrafficOnly);
}
/**
* @return NFS 3.0 protocol support enabled if set to true.
*
*/
public Optional enableNfsV3() {
return Optional.ofNullable(this.enableNfsV3);
}
/**
* @return Encryption settings to be used for server-side encryption for the storage account.
*
*/
public EncryptionResponse encryption() {
return this.encryption;
}
/**
* @return The extendedLocation of the resource.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return If the failover is in progress, the value will be true, otherwise, it will be null.
*
*/
public Boolean failoverInProgress() {
return this.failoverInProgress;
}
/**
* @return Geo Replication Stats
*
*/
public GeoReplicationStatsResponse geoReplicationStats() {
return this.geoReplicationStats;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The identity of the resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return The property is immutable and can only be set to true at the account creation time. When set to true, it enables object level immutability for all the containers in the account by default.
*
*/
public Optional immutableStorageWithVersioning() {
return Optional.ofNullable(this.immutableStorageWithVersioning);
}
/**
* @return Account HierarchicalNamespace enabled if sets to true.
*
*/
public Optional isHnsEnabled() {
return Optional.ofNullable(this.isHnsEnabled);
}
/**
* @return Enables local users feature, if set to true
*
*/
public Optional isLocalUserEnabled() {
return Optional.ofNullable(this.isLocalUserEnabled);
}
/**
* @return Enables Secure File Transfer Protocol, if set to true
*
*/
public Optional isSftpEnabled() {
return Optional.ofNullable(this.isSftpEnabled);
}
/**
* @return Storage account keys creation time.
*
*/
public KeyCreationTimeResponse keyCreationTime() {
return this.keyCreationTime;
}
/**
* @return KeyPolicy assigned to the storage account.
*
*/
public KeyPolicyResponse keyPolicy() {
return this.keyPolicy;
}
/**
* @return Gets the Kind.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Allow large file shares if sets to Enabled. It cannot be disabled once it is enabled.
*
*/
public Optional largeFileSharesState() {
return Optional.ofNullable(this.largeFileSharesState);
}
/**
* @return Gets the timestamp of the most recent instance of a failover to the secondary location. Only the most recent timestamp is retained. This element is not returned if there has never been a failover instance. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
public String lastGeoFailoverTime() {
return this.lastGeoFailoverTime;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Set the minimum TLS version to be permitted on requests to storage. The default interpretation is TLS 1.0 for this property.
*
*/
public Optional minimumTlsVersion() {
return Optional.ofNullable(this.minimumTlsVersion);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Network rule set
*
*/
public NetworkRuleSetResponse networkRuleSet() {
return this.networkRuleSet;
}
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object. Note that Standard_ZRS and Premium_LRS accounts only return the blob endpoint.
*
*/
public EndpointsResponse primaryEndpoints() {
return this.primaryEndpoints;
}
/**
* @return Gets the location of the primary data center for the storage account.
*
*/
public String primaryLocation() {
return this.primaryLocation;
}
/**
* @return List of private endpoint connection associated with the specified storage account
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return Gets the status of the storage account at the time the operation was called.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Allow or disallow public network access to Storage Account. Value is optional but if passed in, must be 'Enabled' or 'Disabled'.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Maintains information about the network routing choice opted by the user for data transfer
*
*/
public Optional routingPreference() {
return Optional.ofNullable(this.routingPreference);
}
/**
* @return SasPolicy assigned to the storage account.
*
*/
public SasPolicyResponse sasPolicy() {
return this.sasPolicy;
}
/**
* @return Gets the URLs that are used to perform a retrieval of a public blob, queue, or table object from the secondary location of the storage account. Only available if the SKU name is Standard_RAGRS.
*
*/
public EndpointsResponse secondaryEndpoints() {
return this.secondaryEndpoints;
}
/**
* @return Gets the location of the geo-replicated secondary for the storage account. Only available if the accountType is Standard_GRS or Standard_RAGRS.
*
*/
public String secondaryLocation() {
return this.secondaryLocation;
}
/**
* @return Gets the SKU.
*
*/
public SkuResponse sku() {
return this.sku;
}
/**
* @return Gets the status indicating whether the primary location of the storage account is available or unavailable.
*
*/
public String statusOfPrimary() {
return this.statusOfPrimary;
}
/**
* @return Gets the status indicating whether the secondary location of the storage account is available or unavailable. Only available if the SKU name is Standard_GRS or Standard_RAGRS.
*
*/
public String statusOfSecondary() {
return this.statusOfSecondary;
}
/**
* @return This property is readOnly and is set by server during asynchronous storage account sku conversion operations.
*
*/
public Optional storageAccountSkuConversionStatus() {
return Optional.ofNullable(this.storageAccountSkuConversionStatus);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetStorageAccountResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessTier;
private @Nullable Boolean allowBlobPublicAccess;
private @Nullable Boolean allowCrossTenantReplication;
private @Nullable Boolean allowSharedKeyAccess;
private @Nullable String allowedCopyScope;
private @Nullable AzureFilesIdentityBasedAuthenticationResponse azureFilesIdentityBasedAuthentication;
private BlobRestoreStatusResponse blobRestoreStatus;
private String creationTime;
private CustomDomainResponse customDomain;
private @Nullable Boolean defaultToOAuthAuthentication;
private @Nullable String dnsEndpointType;
private @Nullable Boolean enableHttpsTrafficOnly;
private @Nullable Boolean enableNfsV3;
private EncryptionResponse encryption;
private @Nullable ExtendedLocationResponse extendedLocation;
private Boolean failoverInProgress;
private GeoReplicationStatsResponse geoReplicationStats;
private String id;
private @Nullable IdentityResponse identity;
private @Nullable ImmutableStorageAccountResponse immutableStorageWithVersioning;
private @Nullable Boolean isHnsEnabled;
private @Nullable Boolean isLocalUserEnabled;
private @Nullable Boolean isSftpEnabled;
private KeyCreationTimeResponse keyCreationTime;
private KeyPolicyResponse keyPolicy;
private String kind;
private @Nullable String largeFileSharesState;
private String lastGeoFailoverTime;
private String location;
private @Nullable String minimumTlsVersion;
private String name;
private NetworkRuleSetResponse networkRuleSet;
private EndpointsResponse primaryEndpoints;
private String primaryLocation;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable RoutingPreferenceResponse routingPreference;
private SasPolicyResponse sasPolicy;
private EndpointsResponse secondaryEndpoints;
private String secondaryLocation;
private SkuResponse sku;
private String statusOfPrimary;
private String statusOfSecondary;
private @Nullable StorageAccountSkuConversionStatusResponse storageAccountSkuConversionStatus;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetStorageAccountResult defaults) {
Objects.requireNonNull(defaults);
this.accessTier = defaults.accessTier;
this.allowBlobPublicAccess = defaults.allowBlobPublicAccess;
this.allowCrossTenantReplication = defaults.allowCrossTenantReplication;
this.allowSharedKeyAccess = defaults.allowSharedKeyAccess;
this.allowedCopyScope = defaults.allowedCopyScope;
this.azureFilesIdentityBasedAuthentication = defaults.azureFilesIdentityBasedAuthentication;
this.blobRestoreStatus = defaults.blobRestoreStatus;
this.creationTime = defaults.creationTime;
this.customDomain = defaults.customDomain;
this.defaultToOAuthAuthentication = defaults.defaultToOAuthAuthentication;
this.dnsEndpointType = defaults.dnsEndpointType;
this.enableHttpsTrafficOnly = defaults.enableHttpsTrafficOnly;
this.enableNfsV3 = defaults.enableNfsV3;
this.encryption = defaults.encryption;
this.extendedLocation = defaults.extendedLocation;
this.failoverInProgress = defaults.failoverInProgress;
this.geoReplicationStats = defaults.geoReplicationStats;
this.id = defaults.id;
this.identity = defaults.identity;
this.immutableStorageWithVersioning = defaults.immutableStorageWithVersioning;
this.isHnsEnabled = defaults.isHnsEnabled;
this.isLocalUserEnabled = defaults.isLocalUserEnabled;
this.isSftpEnabled = defaults.isSftpEnabled;
this.keyCreationTime = defaults.keyCreationTime;
this.keyPolicy = defaults.keyPolicy;
this.kind = defaults.kind;
this.largeFileSharesState = defaults.largeFileSharesState;
this.lastGeoFailoverTime = defaults.lastGeoFailoverTime;
this.location = defaults.location;
this.minimumTlsVersion = defaults.minimumTlsVersion;
this.name = defaults.name;
this.networkRuleSet = defaults.networkRuleSet;
this.primaryEndpoints = defaults.primaryEndpoints;
this.primaryLocation = defaults.primaryLocation;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.routingPreference = defaults.routingPreference;
this.sasPolicy = defaults.sasPolicy;
this.secondaryEndpoints = defaults.secondaryEndpoints;
this.secondaryLocation = defaults.secondaryLocation;
this.sku = defaults.sku;
this.statusOfPrimary = defaults.statusOfPrimary;
this.statusOfSecondary = defaults.statusOfSecondary;
this.storageAccountSkuConversionStatus = defaults.storageAccountSkuConversionStatus;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder accessTier(String accessTier) {
if (accessTier == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "accessTier");
}
this.accessTier = accessTier;
return this;
}
@CustomType.Setter
public Builder allowBlobPublicAccess(@Nullable Boolean allowBlobPublicAccess) {
this.allowBlobPublicAccess = allowBlobPublicAccess;
return this;
}
@CustomType.Setter
public Builder allowCrossTenantReplication(@Nullable Boolean allowCrossTenantReplication) {
this.allowCrossTenantReplication = allowCrossTenantReplication;
return this;
}
@CustomType.Setter
public Builder allowSharedKeyAccess(@Nullable Boolean allowSharedKeyAccess) {
this.allowSharedKeyAccess = allowSharedKeyAccess;
return this;
}
@CustomType.Setter
public Builder allowedCopyScope(@Nullable String allowedCopyScope) {
this.allowedCopyScope = allowedCopyScope;
return this;
}
@CustomType.Setter
public Builder azureFilesIdentityBasedAuthentication(@Nullable AzureFilesIdentityBasedAuthenticationResponse azureFilesIdentityBasedAuthentication) {
this.azureFilesIdentityBasedAuthentication = azureFilesIdentityBasedAuthentication;
return this;
}
@CustomType.Setter
public Builder blobRestoreStatus(BlobRestoreStatusResponse blobRestoreStatus) {
if (blobRestoreStatus == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "blobRestoreStatus");
}
this.blobRestoreStatus = blobRestoreStatus;
return this;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder customDomain(CustomDomainResponse customDomain) {
if (customDomain == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "customDomain");
}
this.customDomain = customDomain;
return this;
}
@CustomType.Setter
public Builder defaultToOAuthAuthentication(@Nullable Boolean defaultToOAuthAuthentication) {
this.defaultToOAuthAuthentication = defaultToOAuthAuthentication;
return this;
}
@CustomType.Setter
public Builder dnsEndpointType(@Nullable String dnsEndpointType) {
this.dnsEndpointType = dnsEndpointType;
return this;
}
@CustomType.Setter
public Builder enableHttpsTrafficOnly(@Nullable Boolean enableHttpsTrafficOnly) {
this.enableHttpsTrafficOnly = enableHttpsTrafficOnly;
return this;
}
@CustomType.Setter
public Builder enableNfsV3(@Nullable Boolean enableNfsV3) {
this.enableNfsV3 = enableNfsV3;
return this;
}
@CustomType.Setter
public Builder encryption(EncryptionResponse encryption) {
if (encryption == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "encryption");
}
this.encryption = encryption;
return this;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder failoverInProgress(Boolean failoverInProgress) {
if (failoverInProgress == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "failoverInProgress");
}
this.failoverInProgress = failoverInProgress;
return this;
}
@CustomType.Setter
public Builder geoReplicationStats(GeoReplicationStatsResponse geoReplicationStats) {
if (geoReplicationStats == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "geoReplicationStats");
}
this.geoReplicationStats = geoReplicationStats;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable IdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder immutableStorageWithVersioning(@Nullable ImmutableStorageAccountResponse immutableStorageWithVersioning) {
this.immutableStorageWithVersioning = immutableStorageWithVersioning;
return this;
}
@CustomType.Setter
public Builder isHnsEnabled(@Nullable Boolean isHnsEnabled) {
this.isHnsEnabled = isHnsEnabled;
return this;
}
@CustomType.Setter
public Builder isLocalUserEnabled(@Nullable Boolean isLocalUserEnabled) {
this.isLocalUserEnabled = isLocalUserEnabled;
return this;
}
@CustomType.Setter
public Builder isSftpEnabled(@Nullable Boolean isSftpEnabled) {
this.isSftpEnabled = isSftpEnabled;
return this;
}
@CustomType.Setter
public Builder keyCreationTime(KeyCreationTimeResponse keyCreationTime) {
if (keyCreationTime == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "keyCreationTime");
}
this.keyCreationTime = keyCreationTime;
return this;
}
@CustomType.Setter
public Builder keyPolicy(KeyPolicyResponse keyPolicy) {
if (keyPolicy == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "keyPolicy");
}
this.keyPolicy = keyPolicy;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder largeFileSharesState(@Nullable String largeFileSharesState) {
this.largeFileSharesState = largeFileSharesState;
return this;
}
@CustomType.Setter
public Builder lastGeoFailoverTime(String lastGeoFailoverTime) {
if (lastGeoFailoverTime == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "lastGeoFailoverTime");
}
this.lastGeoFailoverTime = lastGeoFailoverTime;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder minimumTlsVersion(@Nullable String minimumTlsVersion) {
this.minimumTlsVersion = minimumTlsVersion;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkRuleSet(NetworkRuleSetResponse networkRuleSet) {
if (networkRuleSet == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "networkRuleSet");
}
this.networkRuleSet = networkRuleSet;
return this;
}
@CustomType.Setter
public Builder primaryEndpoints(EndpointsResponse primaryEndpoints) {
if (primaryEndpoints == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "primaryEndpoints");
}
this.primaryEndpoints = primaryEndpoints;
return this;
}
@CustomType.Setter
public Builder primaryLocation(String primaryLocation) {
if (primaryLocation == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "primaryLocation");
}
this.primaryLocation = primaryLocation;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder routingPreference(@Nullable RoutingPreferenceResponse routingPreference) {
this.routingPreference = routingPreference;
return this;
}
@CustomType.Setter
public Builder sasPolicy(SasPolicyResponse sasPolicy) {
if (sasPolicy == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "sasPolicy");
}
this.sasPolicy = sasPolicy;
return this;
}
@CustomType.Setter
public Builder secondaryEndpoints(EndpointsResponse secondaryEndpoints) {
if (secondaryEndpoints == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "secondaryEndpoints");
}
this.secondaryEndpoints = secondaryEndpoints;
return this;
}
@CustomType.Setter
public Builder secondaryLocation(String secondaryLocation) {
if (secondaryLocation == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "secondaryLocation");
}
this.secondaryLocation = secondaryLocation;
return this;
}
@CustomType.Setter
public Builder sku(SkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder statusOfPrimary(String statusOfPrimary) {
if (statusOfPrimary == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "statusOfPrimary");
}
this.statusOfPrimary = statusOfPrimary;
return this;
}
@CustomType.Setter
public Builder statusOfSecondary(String statusOfSecondary) {
if (statusOfSecondary == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "statusOfSecondary");
}
this.statusOfSecondary = statusOfSecondary;
return this;
}
@CustomType.Setter
public Builder storageAccountSkuConversionStatus(@Nullable StorageAccountSkuConversionStatusResponse storageAccountSkuConversionStatus) {
this.storageAccountSkuConversionStatus = storageAccountSkuConversionStatus;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetStorageAccountResult", "type");
}
this.type = type;
return this;
}
public GetStorageAccountResult build() {
final var _resultValue = new GetStorageAccountResult();
_resultValue.accessTier = accessTier;
_resultValue.allowBlobPublicAccess = allowBlobPublicAccess;
_resultValue.allowCrossTenantReplication = allowCrossTenantReplication;
_resultValue.allowSharedKeyAccess = allowSharedKeyAccess;
_resultValue.allowedCopyScope = allowedCopyScope;
_resultValue.azureFilesIdentityBasedAuthentication = azureFilesIdentityBasedAuthentication;
_resultValue.blobRestoreStatus = blobRestoreStatus;
_resultValue.creationTime = creationTime;
_resultValue.customDomain = customDomain;
_resultValue.defaultToOAuthAuthentication = defaultToOAuthAuthentication;
_resultValue.dnsEndpointType = dnsEndpointType;
_resultValue.enableHttpsTrafficOnly = enableHttpsTrafficOnly;
_resultValue.enableNfsV3 = enableNfsV3;
_resultValue.encryption = encryption;
_resultValue.extendedLocation = extendedLocation;
_resultValue.failoverInProgress = failoverInProgress;
_resultValue.geoReplicationStats = geoReplicationStats;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.immutableStorageWithVersioning = immutableStorageWithVersioning;
_resultValue.isHnsEnabled = isHnsEnabled;
_resultValue.isLocalUserEnabled = isLocalUserEnabled;
_resultValue.isSftpEnabled = isSftpEnabled;
_resultValue.keyCreationTime = keyCreationTime;
_resultValue.keyPolicy = keyPolicy;
_resultValue.kind = kind;
_resultValue.largeFileSharesState = largeFileSharesState;
_resultValue.lastGeoFailoverTime = lastGeoFailoverTime;
_resultValue.location = location;
_resultValue.minimumTlsVersion = minimumTlsVersion;
_resultValue.name = name;
_resultValue.networkRuleSet = networkRuleSet;
_resultValue.primaryEndpoints = primaryEndpoints;
_resultValue.primaryLocation = primaryLocation;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.routingPreference = routingPreference;
_resultValue.sasPolicy = sasPolicy;
_resultValue.secondaryEndpoints = secondaryEndpoints;
_resultValue.secondaryLocation = secondaryLocation;
_resultValue.sku = sku;
_resultValue.statusOfPrimary = statusOfPrimary;
_resultValue.statusOfSecondary = statusOfSecondary;
_resultValue.storageAccountSkuConversionStatus = storageAccountSkuConversionStatus;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy