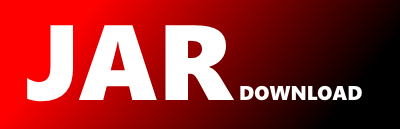
com.pulumi.azurenative.storagecache.AmlFilesystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagecache;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storagecache.AmlFilesystemArgs;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemClientInfoResponse;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemEncryptionSettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemHealthResponse;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemIdentityResponse;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemResponseHsm;
import com.pulumi.azurenative.storagecache.outputs.AmlFilesystemResponseMaintenanceWindow;
import com.pulumi.azurenative.storagecache.outputs.SkuNameResponse;
import com.pulumi.azurenative.storagecache.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An AML file system instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
* Azure REST API version: 2023-05-01.
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:storagecache:AmlFilesystem fs1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.StorageCache/amlFilesystems/{amlFilesystemName}
* ```
*
*/
@ResourceType(type="azure-native:storagecache:AmlFilesystem")
public class AmlFilesystem extends com.pulumi.resources.CustomResource {
/**
* Client information for the AML file system.
*
*/
@Export(name="clientInfo", refs={AmlFilesystemClientInfoResponse.class}, tree="[0]")
private Output clientInfo;
/**
* @return Client information for the AML file system.
*
*/
public Output clientInfo() {
return this.clientInfo;
}
/**
* Specifies encryption settings of the AML file system.
*
*/
@Export(name="encryptionSettings", refs={AmlFilesystemEncryptionSettingsResponse.class}, tree="[0]")
private Output* @Nullable */ AmlFilesystemEncryptionSettingsResponse> encryptionSettings;
/**
* @return Specifies encryption settings of the AML file system.
*
*/
public Output> encryptionSettings() {
return Codegen.optional(this.encryptionSettings);
}
/**
* Subnet used for managing the AML file system and for client-facing operations. This subnet should have at least a /24 subnet mask within the VNET's address space.
*
*/
@Export(name="filesystemSubnet", refs={String.class}, tree="[0]")
private Output filesystemSubnet;
/**
* @return Subnet used for managing the AML file system and for client-facing operations. This subnet should have at least a /24 subnet mask within the VNET's address space.
*
*/
public Output filesystemSubnet() {
return this.filesystemSubnet;
}
/**
* Health of the AML file system.
*
*/
@Export(name="health", refs={AmlFilesystemHealthResponse.class}, tree="[0]")
private Output health;
/**
* @return Health of the AML file system.
*
*/
public Output health() {
return this.health;
}
/**
* Hydration and archive settings and status
*
*/
@Export(name="hsm", refs={AmlFilesystemResponseHsm.class}, tree="[0]")
private Output* @Nullable */ AmlFilesystemResponseHsm> hsm;
/**
* @return Hydration and archive settings and status
*
*/
public Output> hsm() {
return Codegen.optional(this.hsm);
}
/**
* The managed identity used by the AML file system, if configured.
*
*/
@Export(name="identity", refs={AmlFilesystemIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ AmlFilesystemIdentityResponse> identity;
/**
* @return The managed identity used by the AML file system, if configured.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Start time of a 30-minute weekly maintenance window.
*
*/
@Export(name="maintenanceWindow", refs={AmlFilesystemResponseMaintenanceWindow.class}, tree="[0]")
private Output maintenanceWindow;
/**
* @return Start time of a 30-minute weekly maintenance window.
*
*/
public Output maintenanceWindow() {
return this.maintenanceWindow;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* ARM provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return ARM provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* SKU for the resource.
*
*/
@Export(name="sku", refs={SkuNameResponse.class}, tree="[0]")
private Output* @Nullable */ SkuNameResponse> sku;
/**
* @return SKU for the resource.
*
*/
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* The size of the AML file system, in TiB. This might be rounded up.
*
*/
@Export(name="storageCapacityTiB", refs={Double.class}, tree="[0]")
private Output storageCapacityTiB;
/**
* @return The size of the AML file system, in TiB. This might be rounded up.
*
*/
public Output storageCapacityTiB() {
return this.storageCapacityTiB;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Throughput provisioned in MB per sec, calculated as storageCapacityTiB * per-unit storage throughput
*
*/
@Export(name="throughputProvisionedMBps", refs={Integer.class}, tree="[0]")
private Output throughputProvisionedMBps;
/**
* @return Throughput provisioned in MB per sec, calculated as storageCapacityTiB * per-unit storage throughput
*
*/
public Output throughputProvisionedMBps() {
return this.throughputProvisionedMBps;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* Availability zones for resources. This field should only contain a single element in the array.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return Availability zones for resources. This field should only contain a single element in the array.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AmlFilesystem(java.lang.String name) {
this(name, AmlFilesystemArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AmlFilesystem(java.lang.String name, AmlFilesystemArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AmlFilesystem(java.lang.String name, AmlFilesystemArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storagecache:AmlFilesystem", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AmlFilesystem(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storagecache:AmlFilesystem", name, null, makeResourceOptions(options, id), false);
}
private static AmlFilesystemArgs makeArgs(AmlFilesystemArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AmlFilesystemArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:storagecache:amlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20230301preview:AmlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20230301preview:amlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20230501:AmlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20230501:amlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20231101preview:AmlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20231101preview:amlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20240301:AmlFilesystem").build()),
Output.of(Alias.builder().type("azure-native:storagecache/v20240301:amlFilesystem").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AmlFilesystem get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AmlFilesystem(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy