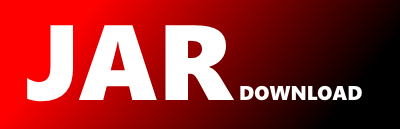
com.pulumi.azurenative.storagecache.outputs.CacheUsernameDownloadSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagecache.outputs;
import com.pulumi.azurenative.storagecache.outputs.CacheUsernameDownloadSettingsResponseCredentials;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CacheUsernameDownloadSettingsResponse {
/**
* @return Determines if the certificate should be automatically downloaded. This applies to 'caCertificateURI' only if 'requireValidCertificate' is true.
*
*/
private @Nullable Boolean autoDownloadCertificate;
/**
* @return The URI of the CA certificate to validate the LDAP secure connection. This field must be populated when 'requireValidCertificate' is set to true.
*
*/
private @Nullable String caCertificateURI;
/**
* @return When present, these are the credentials for the secure LDAP connection.
*
*/
private @Nullable CacheUsernameDownloadSettingsResponseCredentials credentials;
/**
* @return Whether or not the LDAP connection should be encrypted.
*
*/
private @Nullable Boolean encryptLdapConnection;
/**
* @return Whether or not Extended Groups is enabled.
*
*/
private @Nullable Boolean extendedGroups;
/**
* @return The URI of the file containing group information (in /etc/group file format). This field must be populated when 'usernameSource' is set to 'File'.
*
*/
private @Nullable String groupFileURI;
/**
* @return The base distinguished name for the LDAP domain.
*
*/
private @Nullable String ldapBaseDN;
/**
* @return The fully qualified domain name or IP address of the LDAP server to use.
*
*/
private @Nullable String ldapServer;
/**
* @return Determines if the certificates must be validated by a certificate authority. When true, caCertificateURI must be provided.
*
*/
private @Nullable Boolean requireValidCertificate;
/**
* @return The URI of the file containing user information (in /etc/passwd file format). This field must be populated when 'usernameSource' is set to 'File'.
*
*/
private @Nullable String userFileURI;
/**
* @return Indicates whether or not the HPC Cache has performed the username download successfully.
*
*/
private String usernameDownloaded;
/**
* @return This setting determines how the cache gets username and group names for clients.
*
*/
private @Nullable String usernameSource;
private CacheUsernameDownloadSettingsResponse() {}
/**
* @return Determines if the certificate should be automatically downloaded. This applies to 'caCertificateURI' only if 'requireValidCertificate' is true.
*
*/
public Optional autoDownloadCertificate() {
return Optional.ofNullable(this.autoDownloadCertificate);
}
/**
* @return The URI of the CA certificate to validate the LDAP secure connection. This field must be populated when 'requireValidCertificate' is set to true.
*
*/
public Optional caCertificateURI() {
return Optional.ofNullable(this.caCertificateURI);
}
/**
* @return When present, these are the credentials for the secure LDAP connection.
*
*/
public Optional credentials() {
return Optional.ofNullable(this.credentials);
}
/**
* @return Whether or not the LDAP connection should be encrypted.
*
*/
public Optional encryptLdapConnection() {
return Optional.ofNullable(this.encryptLdapConnection);
}
/**
* @return Whether or not Extended Groups is enabled.
*
*/
public Optional extendedGroups() {
return Optional.ofNullable(this.extendedGroups);
}
/**
* @return The URI of the file containing group information (in /etc/group file format). This field must be populated when 'usernameSource' is set to 'File'.
*
*/
public Optional groupFileURI() {
return Optional.ofNullable(this.groupFileURI);
}
/**
* @return The base distinguished name for the LDAP domain.
*
*/
public Optional ldapBaseDN() {
return Optional.ofNullable(this.ldapBaseDN);
}
/**
* @return The fully qualified domain name or IP address of the LDAP server to use.
*
*/
public Optional ldapServer() {
return Optional.ofNullable(this.ldapServer);
}
/**
* @return Determines if the certificates must be validated by a certificate authority. When true, caCertificateURI must be provided.
*
*/
public Optional requireValidCertificate() {
return Optional.ofNullable(this.requireValidCertificate);
}
/**
* @return The URI of the file containing user information (in /etc/passwd file format). This field must be populated when 'usernameSource' is set to 'File'.
*
*/
public Optional userFileURI() {
return Optional.ofNullable(this.userFileURI);
}
/**
* @return Indicates whether or not the HPC Cache has performed the username download successfully.
*
*/
public String usernameDownloaded() {
return this.usernameDownloaded;
}
/**
* @return This setting determines how the cache gets username and group names for clients.
*
*/
public Optional usernameSource() {
return Optional.ofNullable(this.usernameSource);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CacheUsernameDownloadSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean autoDownloadCertificate;
private @Nullable String caCertificateURI;
private @Nullable CacheUsernameDownloadSettingsResponseCredentials credentials;
private @Nullable Boolean encryptLdapConnection;
private @Nullable Boolean extendedGroups;
private @Nullable String groupFileURI;
private @Nullable String ldapBaseDN;
private @Nullable String ldapServer;
private @Nullable Boolean requireValidCertificate;
private @Nullable String userFileURI;
private String usernameDownloaded;
private @Nullable String usernameSource;
public Builder() {}
public Builder(CacheUsernameDownloadSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.autoDownloadCertificate = defaults.autoDownloadCertificate;
this.caCertificateURI = defaults.caCertificateURI;
this.credentials = defaults.credentials;
this.encryptLdapConnection = defaults.encryptLdapConnection;
this.extendedGroups = defaults.extendedGroups;
this.groupFileURI = defaults.groupFileURI;
this.ldapBaseDN = defaults.ldapBaseDN;
this.ldapServer = defaults.ldapServer;
this.requireValidCertificate = defaults.requireValidCertificate;
this.userFileURI = defaults.userFileURI;
this.usernameDownloaded = defaults.usernameDownloaded;
this.usernameSource = defaults.usernameSource;
}
@CustomType.Setter
public Builder autoDownloadCertificate(@Nullable Boolean autoDownloadCertificate) {
this.autoDownloadCertificate = autoDownloadCertificate;
return this;
}
@CustomType.Setter
public Builder caCertificateURI(@Nullable String caCertificateURI) {
this.caCertificateURI = caCertificateURI;
return this;
}
@CustomType.Setter
public Builder credentials(@Nullable CacheUsernameDownloadSettingsResponseCredentials credentials) {
this.credentials = credentials;
return this;
}
@CustomType.Setter
public Builder encryptLdapConnection(@Nullable Boolean encryptLdapConnection) {
this.encryptLdapConnection = encryptLdapConnection;
return this;
}
@CustomType.Setter
public Builder extendedGroups(@Nullable Boolean extendedGroups) {
this.extendedGroups = extendedGroups;
return this;
}
@CustomType.Setter
public Builder groupFileURI(@Nullable String groupFileURI) {
this.groupFileURI = groupFileURI;
return this;
}
@CustomType.Setter
public Builder ldapBaseDN(@Nullable String ldapBaseDN) {
this.ldapBaseDN = ldapBaseDN;
return this;
}
@CustomType.Setter
public Builder ldapServer(@Nullable String ldapServer) {
this.ldapServer = ldapServer;
return this;
}
@CustomType.Setter
public Builder requireValidCertificate(@Nullable Boolean requireValidCertificate) {
this.requireValidCertificate = requireValidCertificate;
return this;
}
@CustomType.Setter
public Builder userFileURI(@Nullable String userFileURI) {
this.userFileURI = userFileURI;
return this;
}
@CustomType.Setter
public Builder usernameDownloaded(String usernameDownloaded) {
if (usernameDownloaded == null) {
throw new MissingRequiredPropertyException("CacheUsernameDownloadSettingsResponse", "usernameDownloaded");
}
this.usernameDownloaded = usernameDownloaded;
return this;
}
@CustomType.Setter
public Builder usernameSource(@Nullable String usernameSource) {
this.usernameSource = usernameSource;
return this;
}
public CacheUsernameDownloadSettingsResponse build() {
final var _resultValue = new CacheUsernameDownloadSettingsResponse();
_resultValue.autoDownloadCertificate = autoDownloadCertificate;
_resultValue.caCertificateURI = caCertificateURI;
_resultValue.credentials = credentials;
_resultValue.encryptLdapConnection = encryptLdapConnection;
_resultValue.extendedGroups = extendedGroups;
_resultValue.groupFileURI = groupFileURI;
_resultValue.ldapBaseDN = ldapBaseDN;
_resultValue.ldapServer = ldapServer;
_resultValue.requireValidCertificate = requireValidCertificate;
_resultValue.userFileURI = userFileURI;
_resultValue.usernameDownloaded = usernameDownloaded;
_resultValue.usernameSource = usernameSource;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy