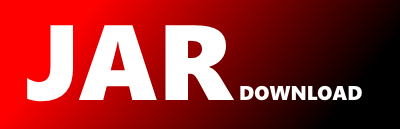
com.pulumi.azurenative.storagesync.outputs.GetServerEndpointResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagesync.outputs;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointCloudTieringStatusResponse;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointRecallStatusResponse;
import com.pulumi.azurenative.storagesync.outputs.ServerEndpointSyncStatusResponse;
import com.pulumi.azurenative.storagesync.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetServerEndpointResult {
/**
* @return Cloud Tiering.
*
*/
private @Nullable String cloudTiering;
/**
* @return Cloud tiering status. Only populated if cloud tiering is enabled.
*
*/
private ServerEndpointCloudTieringStatusResponse cloudTieringStatus;
/**
* @return Friendly Name
*
*/
private @Nullable String friendlyName;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Policy for how namespace and files are recalled during FastDr.
*
*/
private @Nullable String initialDownloadPolicy;
/**
* @return Policy for how the initial upload sync session is performed.
*
*/
private @Nullable String initialUploadPolicy;
/**
* @return Resource Last Operation Name
*
*/
private String lastOperationName;
/**
* @return ServerEndpoint lastWorkflowId
*
*/
private String lastWorkflowId;
/**
* @return Policy for enabling follow-the-sun business models: link local cache to cloud behavior to pre-populate before local access.
*
*/
private @Nullable String localCacheMode;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Offline data transfer
*
*/
private @Nullable String offlineDataTransfer;
/**
* @return Offline data transfer share name
*
*/
private @Nullable String offlineDataTransferShareName;
/**
* @return Offline data transfer storage account resource ID
*
*/
private String offlineDataTransferStorageAccountResourceId;
/**
* @return Offline data transfer storage account tenant ID
*
*/
private String offlineDataTransferStorageAccountTenantId;
/**
* @return ServerEndpoint Provisioning State
*
*/
private String provisioningState;
/**
* @return Recall status. Only populated if cloud tiering is enabled.
*
*/
private ServerEndpointRecallStatusResponse recallStatus;
/**
* @return Server Local path.
*
*/
private @Nullable String serverLocalPath;
/**
* @return Server name
*
*/
private String serverName;
/**
* @return Server Resource Id.
*
*/
private @Nullable String serverResourceId;
/**
* @return Server Endpoint sync status
*
*/
private ServerEndpointSyncStatusResponse syncStatus;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Tier files older than days.
*
*/
private @Nullable Integer tierFilesOlderThanDays;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Level of free space to be maintained by Cloud Tiering if it is enabled.
*
*/
private @Nullable Integer volumeFreeSpacePercent;
private GetServerEndpointResult() {}
/**
* @return Cloud Tiering.
*
*/
public Optional cloudTiering() {
return Optional.ofNullable(this.cloudTiering);
}
/**
* @return Cloud tiering status. Only populated if cloud tiering is enabled.
*
*/
public ServerEndpointCloudTieringStatusResponse cloudTieringStatus() {
return this.cloudTieringStatus;
}
/**
* @return Friendly Name
*
*/
public Optional friendlyName() {
return Optional.ofNullable(this.friendlyName);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Policy for how namespace and files are recalled during FastDr.
*
*/
public Optional initialDownloadPolicy() {
return Optional.ofNullable(this.initialDownloadPolicy);
}
/**
* @return Policy for how the initial upload sync session is performed.
*
*/
public Optional initialUploadPolicy() {
return Optional.ofNullable(this.initialUploadPolicy);
}
/**
* @return Resource Last Operation Name
*
*/
public String lastOperationName() {
return this.lastOperationName;
}
/**
* @return ServerEndpoint lastWorkflowId
*
*/
public String lastWorkflowId() {
return this.lastWorkflowId;
}
/**
* @return Policy for enabling follow-the-sun business models: link local cache to cloud behavior to pre-populate before local access.
*
*/
public Optional localCacheMode() {
return Optional.ofNullable(this.localCacheMode);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Offline data transfer
*
*/
public Optional offlineDataTransfer() {
return Optional.ofNullable(this.offlineDataTransfer);
}
/**
* @return Offline data transfer share name
*
*/
public Optional offlineDataTransferShareName() {
return Optional.ofNullable(this.offlineDataTransferShareName);
}
/**
* @return Offline data transfer storage account resource ID
*
*/
public String offlineDataTransferStorageAccountResourceId() {
return this.offlineDataTransferStorageAccountResourceId;
}
/**
* @return Offline data transfer storage account tenant ID
*
*/
public String offlineDataTransferStorageAccountTenantId() {
return this.offlineDataTransferStorageAccountTenantId;
}
/**
* @return ServerEndpoint Provisioning State
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Recall status. Only populated if cloud tiering is enabled.
*
*/
public ServerEndpointRecallStatusResponse recallStatus() {
return this.recallStatus;
}
/**
* @return Server Local path.
*
*/
public Optional serverLocalPath() {
return Optional.ofNullable(this.serverLocalPath);
}
/**
* @return Server name
*
*/
public String serverName() {
return this.serverName;
}
/**
* @return Server Resource Id.
*
*/
public Optional serverResourceId() {
return Optional.ofNullable(this.serverResourceId);
}
/**
* @return Server Endpoint sync status
*
*/
public ServerEndpointSyncStatusResponse syncStatus() {
return this.syncStatus;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Tier files older than days.
*
*/
public Optional tierFilesOlderThanDays() {
return Optional.ofNullable(this.tierFilesOlderThanDays);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Level of free space to be maintained by Cloud Tiering if it is enabled.
*
*/
public Optional volumeFreeSpacePercent() {
return Optional.ofNullable(this.volumeFreeSpacePercent);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerEndpointResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String cloudTiering;
private ServerEndpointCloudTieringStatusResponse cloudTieringStatus;
private @Nullable String friendlyName;
private String id;
private @Nullable String initialDownloadPolicy;
private @Nullable String initialUploadPolicy;
private String lastOperationName;
private String lastWorkflowId;
private @Nullable String localCacheMode;
private String name;
private @Nullable String offlineDataTransfer;
private @Nullable String offlineDataTransferShareName;
private String offlineDataTransferStorageAccountResourceId;
private String offlineDataTransferStorageAccountTenantId;
private String provisioningState;
private ServerEndpointRecallStatusResponse recallStatus;
private @Nullable String serverLocalPath;
private String serverName;
private @Nullable String serverResourceId;
private ServerEndpointSyncStatusResponse syncStatus;
private SystemDataResponse systemData;
private @Nullable Integer tierFilesOlderThanDays;
private String type;
private @Nullable Integer volumeFreeSpacePercent;
public Builder() {}
public Builder(GetServerEndpointResult defaults) {
Objects.requireNonNull(defaults);
this.cloudTiering = defaults.cloudTiering;
this.cloudTieringStatus = defaults.cloudTieringStatus;
this.friendlyName = defaults.friendlyName;
this.id = defaults.id;
this.initialDownloadPolicy = defaults.initialDownloadPolicy;
this.initialUploadPolicy = defaults.initialUploadPolicy;
this.lastOperationName = defaults.lastOperationName;
this.lastWorkflowId = defaults.lastWorkflowId;
this.localCacheMode = defaults.localCacheMode;
this.name = defaults.name;
this.offlineDataTransfer = defaults.offlineDataTransfer;
this.offlineDataTransferShareName = defaults.offlineDataTransferShareName;
this.offlineDataTransferStorageAccountResourceId = defaults.offlineDataTransferStorageAccountResourceId;
this.offlineDataTransferStorageAccountTenantId = defaults.offlineDataTransferStorageAccountTenantId;
this.provisioningState = defaults.provisioningState;
this.recallStatus = defaults.recallStatus;
this.serverLocalPath = defaults.serverLocalPath;
this.serverName = defaults.serverName;
this.serverResourceId = defaults.serverResourceId;
this.syncStatus = defaults.syncStatus;
this.systemData = defaults.systemData;
this.tierFilesOlderThanDays = defaults.tierFilesOlderThanDays;
this.type = defaults.type;
this.volumeFreeSpacePercent = defaults.volumeFreeSpacePercent;
}
@CustomType.Setter
public Builder cloudTiering(@Nullable String cloudTiering) {
this.cloudTiering = cloudTiering;
return this;
}
@CustomType.Setter
public Builder cloudTieringStatus(ServerEndpointCloudTieringStatusResponse cloudTieringStatus) {
if (cloudTieringStatus == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "cloudTieringStatus");
}
this.cloudTieringStatus = cloudTieringStatus;
return this;
}
@CustomType.Setter
public Builder friendlyName(@Nullable String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder initialDownloadPolicy(@Nullable String initialDownloadPolicy) {
this.initialDownloadPolicy = initialDownloadPolicy;
return this;
}
@CustomType.Setter
public Builder initialUploadPolicy(@Nullable String initialUploadPolicy) {
this.initialUploadPolicy = initialUploadPolicy;
return this;
}
@CustomType.Setter
public Builder lastOperationName(String lastOperationName) {
if (lastOperationName == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "lastOperationName");
}
this.lastOperationName = lastOperationName;
return this;
}
@CustomType.Setter
public Builder lastWorkflowId(String lastWorkflowId) {
if (lastWorkflowId == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "lastWorkflowId");
}
this.lastWorkflowId = lastWorkflowId;
return this;
}
@CustomType.Setter
public Builder localCacheMode(@Nullable String localCacheMode) {
this.localCacheMode = localCacheMode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder offlineDataTransfer(@Nullable String offlineDataTransfer) {
this.offlineDataTransfer = offlineDataTransfer;
return this;
}
@CustomType.Setter
public Builder offlineDataTransferShareName(@Nullable String offlineDataTransferShareName) {
this.offlineDataTransferShareName = offlineDataTransferShareName;
return this;
}
@CustomType.Setter
public Builder offlineDataTransferStorageAccountResourceId(String offlineDataTransferStorageAccountResourceId) {
if (offlineDataTransferStorageAccountResourceId == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "offlineDataTransferStorageAccountResourceId");
}
this.offlineDataTransferStorageAccountResourceId = offlineDataTransferStorageAccountResourceId;
return this;
}
@CustomType.Setter
public Builder offlineDataTransferStorageAccountTenantId(String offlineDataTransferStorageAccountTenantId) {
if (offlineDataTransferStorageAccountTenantId == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "offlineDataTransferStorageAccountTenantId");
}
this.offlineDataTransferStorageAccountTenantId = offlineDataTransferStorageAccountTenantId;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder recallStatus(ServerEndpointRecallStatusResponse recallStatus) {
if (recallStatus == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "recallStatus");
}
this.recallStatus = recallStatus;
return this;
}
@CustomType.Setter
public Builder serverLocalPath(@Nullable String serverLocalPath) {
this.serverLocalPath = serverLocalPath;
return this;
}
@CustomType.Setter
public Builder serverName(String serverName) {
if (serverName == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "serverName");
}
this.serverName = serverName;
return this;
}
@CustomType.Setter
public Builder serverResourceId(@Nullable String serverResourceId) {
this.serverResourceId = serverResourceId;
return this;
}
@CustomType.Setter
public Builder syncStatus(ServerEndpointSyncStatusResponse syncStatus) {
if (syncStatus == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "syncStatus");
}
this.syncStatus = syncStatus;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tierFilesOlderThanDays(@Nullable Integer tierFilesOlderThanDays) {
this.tierFilesOlderThanDays = tierFilesOlderThanDays;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetServerEndpointResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder volumeFreeSpacePercent(@Nullable Integer volumeFreeSpacePercent) {
this.volumeFreeSpacePercent = volumeFreeSpacePercent;
return this;
}
public GetServerEndpointResult build() {
final var _resultValue = new GetServerEndpointResult();
_resultValue.cloudTiering = cloudTiering;
_resultValue.cloudTieringStatus = cloudTieringStatus;
_resultValue.friendlyName = friendlyName;
_resultValue.id = id;
_resultValue.initialDownloadPolicy = initialDownloadPolicy;
_resultValue.initialUploadPolicy = initialUploadPolicy;
_resultValue.lastOperationName = lastOperationName;
_resultValue.lastWorkflowId = lastWorkflowId;
_resultValue.localCacheMode = localCacheMode;
_resultValue.name = name;
_resultValue.offlineDataTransfer = offlineDataTransfer;
_resultValue.offlineDataTransferShareName = offlineDataTransferShareName;
_resultValue.offlineDataTransferStorageAccountResourceId = offlineDataTransferStorageAccountResourceId;
_resultValue.offlineDataTransferStorageAccountTenantId = offlineDataTransferStorageAccountTenantId;
_resultValue.provisioningState = provisioningState;
_resultValue.recallStatus = recallStatus;
_resultValue.serverLocalPath = serverLocalPath;
_resultValue.serverName = serverName;
_resultValue.serverResourceId = serverResourceId;
_resultValue.syncStatus = syncStatus;
_resultValue.systemData = systemData;
_resultValue.tierFilesOlderThanDays = tierFilesOlderThanDays;
_resultValue.type = type;
_resultValue.volumeFreeSpacePercent = volumeFreeSpacePercent;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy