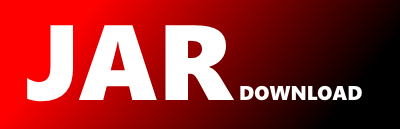
com.pulumi.azurenative.storsimple.StorsimpleFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storsimple;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storsimple.inputs.GetAccessControlRecordArgs;
import com.pulumi.azurenative.storsimple.inputs.GetAccessControlRecordPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBackupPolicyArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBackupPolicyPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBackupScheduleArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBackupSchedulePlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBandwidthSettingArgs;
import com.pulumi.azurenative.storsimple.inputs.GetBandwidthSettingPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerDevicePublicEncryptionKeyArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerDevicePublicEncryptionKeyPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerExtendedInfoArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerExtendedInfoPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetManagerPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetStorageAccountCredentialArgs;
import com.pulumi.azurenative.storsimple.inputs.GetStorageAccountCredentialPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetVolumeArgs;
import com.pulumi.azurenative.storsimple.inputs.GetVolumeContainerArgs;
import com.pulumi.azurenative.storsimple.inputs.GetVolumeContainerPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.GetVolumePlainArgs;
import com.pulumi.azurenative.storsimple.inputs.ListDeviceFailoverSetsArgs;
import com.pulumi.azurenative.storsimple.inputs.ListDeviceFailoverSetsPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.ListDeviceFailoverTarsArgs;
import com.pulumi.azurenative.storsimple.inputs.ListDeviceFailoverTarsPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.ListManagerActivationKeyArgs;
import com.pulumi.azurenative.storsimple.inputs.ListManagerActivationKeyPlainArgs;
import com.pulumi.azurenative.storsimple.inputs.ListManagerPublicEncryptionKeyArgs;
import com.pulumi.azurenative.storsimple.inputs.ListManagerPublicEncryptionKeyPlainArgs;
import com.pulumi.azurenative.storsimple.outputs.GetAccessControlRecordResult;
import com.pulumi.azurenative.storsimple.outputs.GetBackupPolicyResult;
import com.pulumi.azurenative.storsimple.outputs.GetBackupScheduleResult;
import com.pulumi.azurenative.storsimple.outputs.GetBandwidthSettingResult;
import com.pulumi.azurenative.storsimple.outputs.GetManagerDevicePublicEncryptionKeyResult;
import com.pulumi.azurenative.storsimple.outputs.GetManagerExtendedInfoResult;
import com.pulumi.azurenative.storsimple.outputs.GetManagerResult;
import com.pulumi.azurenative.storsimple.outputs.GetStorageAccountCredentialResult;
import com.pulumi.azurenative.storsimple.outputs.GetVolumeContainerResult;
import com.pulumi.azurenative.storsimple.outputs.GetVolumeResult;
import com.pulumi.azurenative.storsimple.outputs.ListDeviceFailoverSetsResult;
import com.pulumi.azurenative.storsimple.outputs.ListDeviceFailoverTarsResult;
import com.pulumi.azurenative.storsimple.outputs.ListManagerActivationKeyResult;
import com.pulumi.azurenative.storsimple.outputs.ListManagerPublicEncryptionKeyResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class StorsimpleFunctions {
/**
* Returns the properties of the specified access control record name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getAccessControlRecord(GetAccessControlRecordArgs args) {
return getAccessControlRecord(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified access control record name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getAccessControlRecordPlain(GetAccessControlRecordPlainArgs args) {
return getAccessControlRecordPlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified access control record name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getAccessControlRecord(GetAccessControlRecordArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getAccessControlRecord", TypeShape.of(GetAccessControlRecordResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified access control record name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getAccessControlRecordPlain(GetAccessControlRecordPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getAccessControlRecord", TypeShape.of(GetAccessControlRecordResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified backup policy name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBackupPolicy(GetBackupPolicyArgs args) {
return getBackupPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified backup policy name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBackupPolicyPlain(GetBackupPolicyPlainArgs args) {
return getBackupPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified backup policy name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBackupPolicy(GetBackupPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getBackupPolicy", TypeShape.of(GetBackupPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified backup policy name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBackupPolicyPlain(GetBackupPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getBackupPolicy", TypeShape.of(GetBackupPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified backup schedule name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBackupSchedule(GetBackupScheduleArgs args) {
return getBackupSchedule(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified backup schedule name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBackupSchedulePlain(GetBackupSchedulePlainArgs args) {
return getBackupSchedulePlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified backup schedule name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBackupSchedule(GetBackupScheduleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getBackupSchedule", TypeShape.of(GetBackupScheduleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified backup schedule name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBackupSchedulePlain(GetBackupSchedulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getBackupSchedule", TypeShape.of(GetBackupScheduleResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified bandwidth setting name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBandwidthSetting(GetBandwidthSettingArgs args) {
return getBandwidthSetting(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified bandwidth setting name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBandwidthSettingPlain(GetBandwidthSettingPlainArgs args) {
return getBandwidthSettingPlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified bandwidth setting name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getBandwidthSetting(GetBandwidthSettingArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getBandwidthSetting", TypeShape.of(GetBandwidthSettingResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified bandwidth setting name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getBandwidthSettingPlain(GetBandwidthSettingPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getBandwidthSetting", TypeShape.of(GetBandwidthSettingResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified manager name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static Output getManager(GetManagerArgs args) {
return getManager(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified manager name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static CompletableFuture getManagerPlain(GetManagerPlainArgs args) {
return getManagerPlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified manager name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static Output getManager(GetManagerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getManager", TypeShape.of(GetManagerResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified manager name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static CompletableFuture getManagerPlain(GetManagerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getManager", TypeShape.of(GetManagerResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the public encryption key of the device.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getManagerDevicePublicEncryptionKey(GetManagerDevicePublicEncryptionKeyArgs args) {
return getManagerDevicePublicEncryptionKey(args, InvokeOptions.Empty);
}
/**
* Returns the public encryption key of the device.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getManagerDevicePublicEncryptionKeyPlain(GetManagerDevicePublicEncryptionKeyPlainArgs args) {
return getManagerDevicePublicEncryptionKeyPlain(args, InvokeOptions.Empty);
}
/**
* Returns the public encryption key of the device.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getManagerDevicePublicEncryptionKey(GetManagerDevicePublicEncryptionKeyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getManagerDevicePublicEncryptionKey", TypeShape.of(GetManagerDevicePublicEncryptionKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the public encryption key of the device.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getManagerDevicePublicEncryptionKeyPlain(GetManagerDevicePublicEncryptionKeyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getManagerDevicePublicEncryptionKey", TypeShape.of(GetManagerDevicePublicEncryptionKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the extended information of the specified manager name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getManagerExtendedInfo(GetManagerExtendedInfoArgs args) {
return getManagerExtendedInfo(args, InvokeOptions.Empty);
}
/**
* Returns the extended information of the specified manager name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getManagerExtendedInfoPlain(GetManagerExtendedInfoPlainArgs args) {
return getManagerExtendedInfoPlain(args, InvokeOptions.Empty);
}
/**
* Returns the extended information of the specified manager name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getManagerExtendedInfo(GetManagerExtendedInfoArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getManagerExtendedInfo", TypeShape.of(GetManagerExtendedInfoResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the extended information of the specified manager name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getManagerExtendedInfoPlain(GetManagerExtendedInfoPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getManagerExtendedInfo", TypeShape.of(GetManagerExtendedInfoResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified storage account credential name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static Output getStorageAccountCredential(GetStorageAccountCredentialArgs args) {
return getStorageAccountCredential(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified storage account credential name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static CompletableFuture getStorageAccountCredentialPlain(GetStorageAccountCredentialPlainArgs args) {
return getStorageAccountCredentialPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified storage account credential name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static Output getStorageAccountCredential(GetStorageAccountCredentialArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getStorageAccountCredential", TypeShape.of(GetStorageAccountCredentialResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified storage account credential name.
* Azure REST API version: 2017-06-01.
*
* Other available API versions: 2016-10-01.
*
*/
public static CompletableFuture getStorageAccountCredentialPlain(GetStorageAccountCredentialPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getStorageAccountCredential", TypeShape.of(GetStorageAccountCredentialResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified volume name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getVolume(GetVolumeArgs args) {
return getVolume(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified volume name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args) {
return getVolumePlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties of the specified volume name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getVolume(GetVolumeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties of the specified volume name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified volume container name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getVolumeContainer(GetVolumeContainerArgs args) {
return getVolumeContainer(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified volume container name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getVolumeContainerPlain(GetVolumeContainerPlainArgs args) {
return getVolumeContainerPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified volume container name.
* Azure REST API version: 2017-06-01.
*
*/
public static Output getVolumeContainer(GetVolumeContainerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:getVolumeContainer", TypeShape.of(GetVolumeContainerResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified volume container name.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture getVolumeContainerPlain(GetVolumeContainerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:getVolumeContainer", TypeShape.of(GetVolumeContainerResult.class), args, Utilities.withVersion(options));
}
/**
* Returns all failover sets for a given device and their eligibility for participating in a failover. A failover set refers to a set of volume containers that need to be failed-over as a single unit to maintain data integrity.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listDeviceFailoverSets(ListDeviceFailoverSetsArgs args) {
return listDeviceFailoverSets(args, InvokeOptions.Empty);
}
/**
* Returns all failover sets for a given device and their eligibility for participating in a failover. A failover set refers to a set of volume containers that need to be failed-over as a single unit to maintain data integrity.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listDeviceFailoverSetsPlain(ListDeviceFailoverSetsPlainArgs args) {
return listDeviceFailoverSetsPlain(args, InvokeOptions.Empty);
}
/**
* Returns all failover sets for a given device and their eligibility for participating in a failover. A failover set refers to a set of volume containers that need to be failed-over as a single unit to maintain data integrity.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listDeviceFailoverSets(ListDeviceFailoverSetsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:listDeviceFailoverSets", TypeShape.of(ListDeviceFailoverSetsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns all failover sets for a given device and their eligibility for participating in a failover. A failover set refers to a set of volume containers that need to be failed-over as a single unit to maintain data integrity.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listDeviceFailoverSetsPlain(ListDeviceFailoverSetsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:listDeviceFailoverSets", TypeShape.of(ListDeviceFailoverSetsResult.class), args, Utilities.withVersion(options));
}
/**
* Given a list of volume containers to be failed over from a source device, this method returns the eligibility result, as a failover target, for all devices under that resource.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listDeviceFailoverTars(ListDeviceFailoverTarsArgs args) {
return listDeviceFailoverTars(args, InvokeOptions.Empty);
}
/**
* Given a list of volume containers to be failed over from a source device, this method returns the eligibility result, as a failover target, for all devices under that resource.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listDeviceFailoverTarsPlain(ListDeviceFailoverTarsPlainArgs args) {
return listDeviceFailoverTarsPlain(args, InvokeOptions.Empty);
}
/**
* Given a list of volume containers to be failed over from a source device, this method returns the eligibility result, as a failover target, for all devices under that resource.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listDeviceFailoverTars(ListDeviceFailoverTarsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:listDeviceFailoverTars", TypeShape.of(ListDeviceFailoverTarsResult.class), args, Utilities.withVersion(options));
}
/**
* Given a list of volume containers to be failed over from a source device, this method returns the eligibility result, as a failover target, for all devices under that resource.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listDeviceFailoverTarsPlain(ListDeviceFailoverTarsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:listDeviceFailoverTars", TypeShape.of(ListDeviceFailoverTarsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the activation key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listManagerActivationKey(ListManagerActivationKeyArgs args) {
return listManagerActivationKey(args, InvokeOptions.Empty);
}
/**
* Returns the activation key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listManagerActivationKeyPlain(ListManagerActivationKeyPlainArgs args) {
return listManagerActivationKeyPlain(args, InvokeOptions.Empty);
}
/**
* Returns the activation key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listManagerActivationKey(ListManagerActivationKeyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:listManagerActivationKey", TypeShape.of(ListManagerActivationKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the activation key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listManagerActivationKeyPlain(ListManagerActivationKeyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:listManagerActivationKey", TypeShape.of(ListManagerActivationKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the symmetric encrypted public encryption key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listManagerPublicEncryptionKey(ListManagerPublicEncryptionKeyArgs args) {
return listManagerPublicEncryptionKey(args, InvokeOptions.Empty);
}
/**
* Returns the symmetric encrypted public encryption key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listManagerPublicEncryptionKeyPlain(ListManagerPublicEncryptionKeyPlainArgs args) {
return listManagerPublicEncryptionKeyPlain(args, InvokeOptions.Empty);
}
/**
* Returns the symmetric encrypted public encryption key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static Output listManagerPublicEncryptionKey(ListManagerPublicEncryptionKeyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storsimple:listManagerPublicEncryptionKey", TypeShape.of(ListManagerPublicEncryptionKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the symmetric encrypted public encryption key of the manager.
* Azure REST API version: 2017-06-01.
*
*/
public static CompletableFuture listManagerPublicEncryptionKeyPlain(ListManagerPublicEncryptionKeyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storsimple:listManagerPublicEncryptionKey", TypeShape.of(ListManagerPublicEncryptionKeyResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy