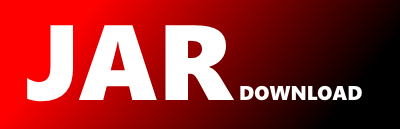
com.pulumi.azurenative.streamanalytics.inputs.AzureFunctionOutputDataSourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Defines the metadata of AzureFunctionOutputDataSource
*
*/
public final class AzureFunctionOutputDataSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureFunctionOutputDataSourceArgs Empty = new AzureFunctionOutputDataSourceArgs();
/**
* If you want to use an Azure Function from another subscription, you can do so by providing the key to access your function.
*
*/
@Import(name="apiKey")
private @Nullable Output apiKey;
/**
* @return If you want to use an Azure Function from another subscription, you can do so by providing the key to access your function.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy