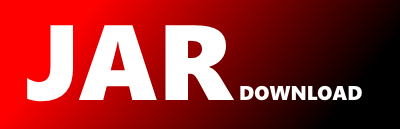
com.pulumi.azurenative.streamanalytics.inputs.EventHubV2OutputDataSourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.inputs;
import com.pulumi.azurenative.streamanalytics.enums.AuthenticationMode;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes an Event Hub output data source.
*
*/
public final class EventHubV2OutputDataSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final EventHubV2OutputDataSourceArgs Empty = new EventHubV2OutputDataSourceArgs();
/**
* Authentication Mode.
*
*/
@Import(name="authenticationMode")
private @Nullable Output> authenticationMode;
/**
* @return Authentication Mode.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy