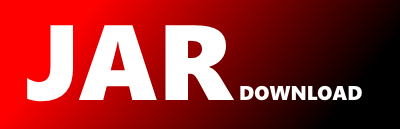
com.pulumi.azurenative.streamanalytics.outputs.AzureSqlReferenceInputDataSourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureSqlReferenceInputDataSourceResponse {
/**
* @return This element is associated with the datasource element. This is the name of the database that output will be written to.
*
*/
private @Nullable String database;
/**
* @return This element is associated with the datasource element. This query is used to fetch incremental changes from the SQL database. To use this option, we recommend using temporal tables in Azure SQL Database.
*
*/
private @Nullable String deltaSnapshotQuery;
/**
* @return This element is associated with the datasource element. This query is used to fetch data from the sql database.
*
*/
private @Nullable String fullSnapshotQuery;
/**
* @return This element is associated with the datasource element. This is the password that will be used to connect to the SQL Database instance.
*
*/
private @Nullable String password;
/**
* @return This element is associated with the datasource element. This indicates how frequently the data will be fetched from the database. It is of DateTime format.
*
*/
private @Nullable String refreshRate;
/**
* @return Indicates the type of data refresh option.
*
*/
private @Nullable String refreshType;
/**
* @return This element is associated with the datasource element. This is the name of the server that contains the database that will be written to.
*
*/
private @Nullable String server;
/**
* @return This element is associated with the datasource element. The name of the table in the Azure SQL database..
*
*/
private @Nullable String table;
/**
* @return Indicates the type of input data source containing reference data. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.Sql/Server/Database'.
*
*/
private String type;
/**
* @return This element is associated with the datasource element. This is the user name that will be used to connect to the SQL Database instance.
*
*/
private @Nullable String user;
private AzureSqlReferenceInputDataSourceResponse() {}
/**
* @return This element is associated with the datasource element. This is the name of the database that output will be written to.
*
*/
public Optional database() {
return Optional.ofNullable(this.database);
}
/**
* @return This element is associated with the datasource element. This query is used to fetch incremental changes from the SQL database. To use this option, we recommend using temporal tables in Azure SQL Database.
*
*/
public Optional deltaSnapshotQuery() {
return Optional.ofNullable(this.deltaSnapshotQuery);
}
/**
* @return This element is associated with the datasource element. This query is used to fetch data from the sql database.
*
*/
public Optional fullSnapshotQuery() {
return Optional.ofNullable(this.fullSnapshotQuery);
}
/**
* @return This element is associated with the datasource element. This is the password that will be used to connect to the SQL Database instance.
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return This element is associated with the datasource element. This indicates how frequently the data will be fetched from the database. It is of DateTime format.
*
*/
public Optional refreshRate() {
return Optional.ofNullable(this.refreshRate);
}
/**
* @return Indicates the type of data refresh option.
*
*/
public Optional refreshType() {
return Optional.ofNullable(this.refreshType);
}
/**
* @return This element is associated with the datasource element. This is the name of the server that contains the database that will be written to.
*
*/
public Optional server() {
return Optional.ofNullable(this.server);
}
/**
* @return This element is associated with the datasource element. The name of the table in the Azure SQL database..
*
*/
public Optional table() {
return Optional.ofNullable(this.table);
}
/**
* @return Indicates the type of input data source containing reference data. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.Sql/Server/Database'.
*
*/
public String type() {
return this.type;
}
/**
* @return This element is associated with the datasource element. This is the user name that will be used to connect to the SQL Database instance.
*
*/
public Optional user() {
return Optional.ofNullable(this.user);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureSqlReferenceInputDataSourceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String database;
private @Nullable String deltaSnapshotQuery;
private @Nullable String fullSnapshotQuery;
private @Nullable String password;
private @Nullable String refreshRate;
private @Nullable String refreshType;
private @Nullable String server;
private @Nullable String table;
private String type;
private @Nullable String user;
public Builder() {}
public Builder(AzureSqlReferenceInputDataSourceResponse defaults) {
Objects.requireNonNull(defaults);
this.database = defaults.database;
this.deltaSnapshotQuery = defaults.deltaSnapshotQuery;
this.fullSnapshotQuery = defaults.fullSnapshotQuery;
this.password = defaults.password;
this.refreshRate = defaults.refreshRate;
this.refreshType = defaults.refreshType;
this.server = defaults.server;
this.table = defaults.table;
this.type = defaults.type;
this.user = defaults.user;
}
@CustomType.Setter
public Builder database(@Nullable String database) {
this.database = database;
return this;
}
@CustomType.Setter
public Builder deltaSnapshotQuery(@Nullable String deltaSnapshotQuery) {
this.deltaSnapshotQuery = deltaSnapshotQuery;
return this;
}
@CustomType.Setter
public Builder fullSnapshotQuery(@Nullable String fullSnapshotQuery) {
this.fullSnapshotQuery = fullSnapshotQuery;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder refreshRate(@Nullable String refreshRate) {
this.refreshRate = refreshRate;
return this;
}
@CustomType.Setter
public Builder refreshType(@Nullable String refreshType) {
this.refreshType = refreshType;
return this;
}
@CustomType.Setter
public Builder server(@Nullable String server) {
this.server = server;
return this;
}
@CustomType.Setter
public Builder table(@Nullable String table) {
this.table = table;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("AzureSqlReferenceInputDataSourceResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder user(@Nullable String user) {
this.user = user;
return this;
}
public AzureSqlReferenceInputDataSourceResponse build() {
final var _resultValue = new AzureSqlReferenceInputDataSourceResponse();
_resultValue.database = database;
_resultValue.deltaSnapshotQuery = deltaSnapshotQuery;
_resultValue.fullSnapshotQuery = fullSnapshotQuery;
_resultValue.password = password;
_resultValue.refreshRate = refreshRate;
_resultValue.refreshType = refreshType;
_resultValue.server = server;
_resultValue.table = table;
_resultValue.type = type;
_resultValue.user = user;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy